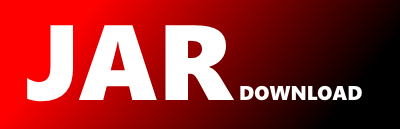
com.icthh.xm.commons.search.ElasticsearchOperations Maven / Gradle / Ivy
/*
* Original version of this file is located at:
* https://github.com/spring-projects/spring-data-elasticsearch/blob/3.1.12.RELEASE/src/main/java/org/springframework/data/elasticsearch/core/ElasticsearchOperations.java
*
* Copyright 2013-2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.icthh.xm.commons.search;
import com.icthh.xm.commons.search.dto.SearchDto;
import com.icthh.xm.commons.search.mapper.extractor.ResultsExtractor;
import com.icthh.xm.commons.search.query.SearchQuery;
import com.icthh.xm.commons.search.query.dto.DeleteQuery;
import com.icthh.xm.commons.search.query.dto.GetQuery;
import com.icthh.xm.commons.search.query.dto.IndexQuery;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import java.util.List;
import java.util.Set;
public interface ElasticsearchOperations {
static final String INDEX_QUERY_TYPE = "xmentity";
String composeIndexName(String tenantCode);
String getIndexName();
boolean createIndex(Class clazz);
boolean createIndex(String indexName);
boolean createIndex(String indexName, Object settings);
boolean putMapping(Class clazz);
boolean putMapping(String indexName, String type, Object mappings);
boolean putMapping(Class clazz, Object mappings);
T queryForObject(GetQuery query, Class clazz);
Page queryForPage(SearchQuery query, Class clazz);
List queryForList(SearchQuery query, Class clazz);
List queryForIds(SearchQuery query);
long count(SearchQuery query, Class clazz);
long count(SearchQuery query);
String index(IndexQuery query);
boolean indexExists(Class clazz);
boolean indexExists(String indexName);
void bulkIndex(List queries);
String delete(String indexName, String type, String id);
String delete(Class clazz, String id);
void delete(DeleteQuery query, Class clazz);
void delete(DeleteQuery query);
boolean deleteIndex(String indexName);
boolean deleteIndex(Class clazz);
void refresh(String tenantCode);
void refresh(Class clazz);
Page startScroll(long scrollTimeInMillis, SearchQuery query, Class clazz);
Page continueScroll(String scrollId, long scrollTimeInMillis, Class clazz);
void clearScroll(String scrollId);
T query(SearchQuery query, ResultsExtractor resultsExtractor);
List search(String query, Class entityClass, String privilegeKey);
Page searchForPage(SearchDto searchDto, String privilegeKey);
Page search(Long scrollTimeInMillis,
String query,
Pageable pageable,
Class entityClass,
String privilegeKey);
Page searchByQueryAndTypeKey(String query,
String typeKey,
Pageable pageable,
Class entityClass,
String privilegeKey);
public Page searchWithIdNotIn(String query,
Set ids,
String targetEntityTypeKey,
Pageable pageable,
Class entityClass,
String privilegeKey);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy