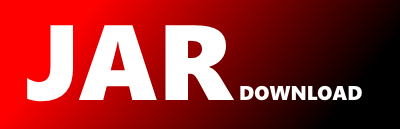
com.identityx.auth.support.DefaultRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of daon-http-digest-auth Show documentation
Show all versions of daon-http-digest-auth Show documentation
Client library used for adding authentication to http messages as required by IdentityX Rest Services
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.identityx.auth.support;
import java.io.InputStream;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.NoSuchElementException;
import java.util.Scanner;
import com.identityx.auth.def.IRequest;
import com.identityx.auth.impl.HttpHeaders;
import com.identityx.auth.impl.HttpMethod;
import com.identityx.auth.impl.QueryString;
import com.identityx.auth.lang.Strings;
import com.identityx.auth.util.StringInputStream;
public class DefaultRequest extends AbstractHttpMessage implements IRequest {
private final HttpMethod method;
private final URI resourceUrl;
private final HttpHeaders headers;
private final QueryString queryString;
private InputStream body;
public DefaultRequest(HttpMethod method, String href) {
this(method, href, null, null, null, -1L);
}
public DefaultRequest(HttpMethod method, String href, QueryString query) {
this(method, href, query, null, null, -1L);
}
public DefaultRequest(HttpMethod method, String href, QueryString query, HttpHeaders headers, InputStream body) {
this.method = method;
href = href.replaceAll(" ", "%20");
if ((method.equals(HttpMethod.GET)) && HasContent(body)) {
throw new RequestAuthenticationException("Unable to create GET request with a body content");
}
URI uri;
String[] split = Strings.split(href, "?");
if (split != null) {
uri = URI.create(split[0]);
this.queryString = QueryString.create(split[1]);
if (query != null && !query.isEmpty()) {
this.queryString.putAll(query);
}
} else {
//no question mark = no query string, so create a new one:
uri = URI.create(href);
this.queryString = query != null ? query : new QueryString();
}
try {
this.resourceUrl = normalizeURI(uri);
} catch (URISyntaxException e) {
throw new IllegalArgumentException("href", e);
}
this.headers = headers != null ? headers : new HttpHeaders();
this.body = body;
}
public static URI normalizeURI(URI uri) throws URISyntaxException {
URI resUri;
if (uri.isOpaque()) {
resUri = new URI(uri.getScheme().toLowerCase(),
uri.getSchemeSpecificPart(), uri.getFragment());
}
else {
resUri = new URI(uri.getScheme().toLowerCase(), uri.getUserInfo(),
uri.getHost().toLowerCase(), uri.getPort(), uri.getPath(), uri.getQuery(), uri.getFragment());
}
return resUri.normalize();
}
public DefaultRequest(HttpMethod method, String href, QueryString query, HttpHeaders headers, InputStream body, long contentLength) {
this(method, href, query, headers, body);
this.headers.setContentLength(contentLength);
}
public boolean HasContent(InputStream body) {
boolean hasContent = true;
try {
if (body == null) {
hasContent = false;
return hasContent;
}
if (body instanceof StringInputStream) {
hasContent = ((StringInputStream)body).toString().length() > 0;
return hasContent;
}
} catch (Exception e) {
//failed read
hasContent = false;
return hasContent;
}
if (!body.markSupported()) {
throw new RequestAuthenticationException("Unable to read request payload to authenticate request (mark not supported).");
}
body.mark(-1);
//convert InputStream into a String in one shot:
String string;
try {
string = new Scanner(body, "UTF-8").useDelimiter("\\A").next();
if (string.length() == 0) {
hasContent = false;
}
} catch (NoSuchElementException nsee) {
hasContent = false;
}
return hasContent;
}
public HttpMethod getMethod() {
return method;
}
public URI getResourceUrl() {
return this.resourceUrl;
}
public HttpHeaders getHeaders() {
return headers;
}
public void setHeaders(HttpHeaders headers) {
this.headers.clear();
this.headers.putAll(headers);
}
public QueryString getQueryString() {
return this.queryString;
}
public void setQueryString(QueryString queryString) {
this.queryString.clear();
this.queryString.putAll(queryString);
}
public InputStream getBody() {
return body;
}
public void setBody(InputStream body, long length) {
this.body = body;
getHeaders().setContentLength(length);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy