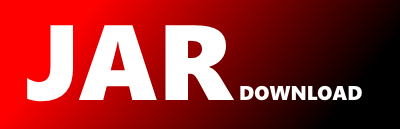
com.identityx.auth.util.CertsUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of daon-http-digest-auth Show documentation
Show all versions of daon-http-digest-auth Show documentation
Client library used for adding authentication to http messages as required by IdentityX Rest Services
package com.identityx.auth.util;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.security.PublicKey;
import java.security.SignatureException;
import java.security.cert.CertificateException;
import java.security.cert.CertificateFactory;
import java.security.cert.X509Certificate;
import java.util.ArrayList;
import java.util.List;
public class CertsUtil {
public static final String certBegin = "-----BEGIN CERTIFICATE-----";
public static final String certEnd = "-----END CERTIFICATE-----";
public static List certsFromString(String certs) throws CertificateException, IOException {
List clientCertPathList = null;
String certBeginNoSpace = certBegin.replace(" ", "");
String certEndNoSpace = certEnd.replace(" ", "");
// some pems have a ----BEGINCERTIFICATE----
if (certs.contains(certBeginNoSpace)) {
String allCerts = certs;
allCerts = allCerts.replace(certBegin, certBeginNoSpace);
allCerts = allCerts.replace(certEnd, certEndNoSpace);
String splits[] = allCerts.split(certBeginNoSpace);
if (splits == null || splits.length < 1) {
return null;
}
clientCertPathList = new ArrayList();
for (int i = 1; i < splits.length; i++) {
String iSplit = splits[i].replace(certEndNoSpace, "").replace("\n", "").replace("\r", "");
byte[] certBytes = Base64.decodeBase64(iSplit);
CertificateFactory fact = CertificateFactory.getInstance("X.509");
X509Certificate iCert = (X509Certificate) fact.generateCertificate(new ByteArrayInputStream(certBytes));
clientCertPathList.add(iCert);
}
}
else {
CertificateFactory fact = CertificateFactory.getInstance("X.509");
InputStream certsStream = new ByteArrayInputStream(certs.getBytes(StandardCharsets.UTF_8));
clientCertPathList = new ArrayList(fact.generateCertificates(certsStream));
}
return clientCertPathList;
}
/**
* Checks whether given X.509 certificate is self-signed.
*/
public static boolean isSelfSigned(X509Certificate cert) throws CertificateException, NoSuchAlgorithmException, NoSuchProviderException {
try {
// Try to verify certificate signature with its own public key
PublicKey key = cert.getPublicKey();
cert.verify(key);
return true;
} catch (SignatureException sigEx) {
// Invalid signature --> not self-signed
return false;
} catch (InvalidKeyException keyEx) {
// Invalid key --> not self-signed
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy