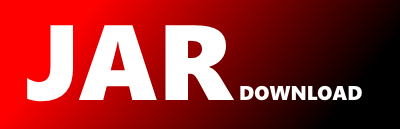
com.identityx.auth.impl.DefaultSSLConnectionSocketFactoryProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of daon-http-digest-auth Show documentation
Show all versions of daon-http-digest-auth Show documentation
Client library used for adding authentication to http messages as required by IdentityX Rest Services
package com.identityx.auth.impl;
import java.security.KeyManagementException;
import java.security.KeyStore;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
import java.security.UnrecoverableKeyException;
import javax.net.ssl.SSLContext;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.ssl.SSLContexts;
import org.apache.http.ssl.TrustStrategy;
/**
*
* @author hanucuta
* Just a sample class to show how to create a SSLConnectionSocketFactory that can be used with mutual auth.
*
*/
public class DefaultSSLConnectionSocketFactoryProvider {
public SSLConnectionSocketFactory get(KeyStore trustStore, TrustStrategy trustStrategy, KeyStore clientStore, String clientStorePass) {
SSLContext sslcontext = null;
if (trustStore == null) throw new IllegalArgumentException("trustStore cannot be null");
if (clientStore == null) throw new IllegalArgumentException("clientStore cannot be null");
if (clientStorePass == null) throw new IllegalArgumentException("clientStorePass cannot be null");
try {
sslcontext = SSLContexts.custom().useProtocol("TLS")
.loadTrustMaterial(trustStore, trustStrategy)
.loadKeyMaterial(clientStore, clientStorePass.toCharArray())
.build();
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(sslcontext);
return sslsf;
} catch (KeyManagementException | UnrecoverableKeyException
| NoSuchAlgorithmException | KeyStoreException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
public SSLConnectionSocketFactory get(KeyStore trustStore, TrustStrategy trustStrategy) {
SSLContext sslcontext = null;
if (trustStore == null) throw new IllegalArgumentException("trustStore cannot be null");
try {
sslcontext = SSLContexts.custom().useProtocol("TLS")
.loadTrustMaterial(trustStore, trustStrategy)
.build();
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(sslcontext);
return sslsf;
} catch (KeyManagementException | NoSuchAlgorithmException | KeyStoreException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy