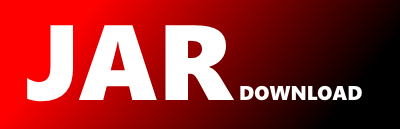
com.identityx.clientSDK.credentialsProviders.EncryptedKeyPropFileCredentialsProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of daon-http-digest-auth Show documentation
Show all versions of daon-http-digest-auth Show documentation
Client library used for adding authentication to http messages as required by IdentityX Rest Services
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.identityx.clientSDK.credentialsProviders;
import java.io.IOException;
import java.io.InputStream;
import java.security.Key;
import java.security.KeyStore;
import java.util.Properties;
import javax.crypto.Cipher;
import org.apache.commons.codec.binary.Base64;
import com.identityx.auth.def.IApiKey;
import com.identityx.auth.def.ITokenKey;
import com.identityx.auth.impl.keys.SharedSecretApiKey;
import com.identityx.clientSDK.def.ICredentialsProvider;
import com.identityx.clientSDK.exceptions.ClientInitializationException;
/**
* Provides credentials from a properties file containing an encrypted shared secret.
* The credentials file is loaded from the class path.
* The file has to be a ".properties" file and contain the fields
* sharedKeyId, encryptedSharedKey, serviceUrl.
* The key is decrypted based on the jks file provided to the constructor.
*
*/
public class EncryptedKeyPropFileCredentialsProvider implements ICredentialsProvider {
protected static String defaultCredentialsFileName = "credentials.properties";
private String baseUrl;
private ITokenKey apiKey;
private IApiKey responseApiKey = null;
/*
* Initialises credentials based on the input .properties file. The encrypted shared key in the properties file
* will be decrypted with the private key in the provided jks file.
*
* @param jksFileName - required, the name of the jks file
* @param jksPassword - required, password for accessing the jks file
* @param credentialsFileName - optional, name of the credentials properties file name. If null default "credentials.properties" is assumed
* @param keyAlias - optional, the alias of the private key in the jks file. If null, the first key found is used
* @param keyPassword - optional, the password required to read the private key. If null, same as jksPassword is used
*
*/
public EncryptedKeyPropFileCredentialsProvider(String jksFileName, String jksPassword, String credentialsFileName, String keyAlias, String keyPassword) throws ClientInitializationException {
init(jksFileName, jksPassword, credentialsFileName, keyAlias, keyPassword);
}
/*
* Initialises credentials based on the input .properties file. The encrypted shared key in the properties file
* will be decrypted with the private key in the provided jks file.
*
* @param jksInputStream - required, input stream of the jks file
* @param jksPassword - required, password for accessing the jks file
* @param credentialsInputStream - required, input stream of the credentials properties file name.
* @param keyAlias - optional, the alias of the private key in the jks file. If null, the first key found is used
* @param keyPassword - optional, the password required to read the private key. If null, same as jksPassword is used
*
*/
public EncryptedKeyPropFileCredentialsProvider(InputStream jksInputStream, String jksPassword, InputStream credentialsInputStream, String keyAlias, String keyPassword) throws ClientInitializationException {
init(jksInputStream, jksPassword, credentialsInputStream, keyAlias, keyPassword);
}
public EncryptedKeyPropFileCredentialsProvider(KeyStore keyStore, InputStream credentialsInputStream, String keyAlias, String keyPassword) throws ClientInitializationException {
init(keyStore, credentialsInputStream, keyAlias, keyPassword);
}
protected void init(String jksFileName, String jksPassword, String credentialsFileName, String keyAlias, String keyPassword) throws ClientInitializationException {
if (jksFileName == null) throw new IllegalArgumentException("Param jksFileName cannot be null");
if (jksPassword == null) throw new IllegalArgumentException("Param password cannot be null");
InputStream credentialsInputStream = null;
InputStream jksInputStream = null;
if (credentialsFileName == null) credentialsFileName = defaultCredentialsFileName;
try {
ClassLoader loader = Thread.currentThread().getContextClassLoader();
credentialsInputStream = loader.getResourceAsStream(credentialsFileName);
if (credentialsInputStream == null) throw new IOException("Cannot open the file " + credentialsFileName);
jksInputStream = loader.getResourceAsStream(jksFileName);
init(jksInputStream, jksPassword, credentialsInputStream, keyAlias, keyPassword);
} catch (Exception ex) {
throw new ClientInitializationException("Failed to initialize the Credential Provider", ex);
} finally {
if (jksInputStream != null) {
try {
jksInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (credentialsInputStream != null) {
try {
credentialsInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
protected void init(InputStream jksInputStream, String jksPassword, InputStream credentialsInputStream, String keyAlias, String keyPassword) throws ClientInitializationException {
if (jksInputStream == null) throw new IllegalArgumentException("Param jksInputStream cannot be null");
if (credentialsInputStream == null) throw new IllegalArgumentException("Param credentialsInputStream cannot be null");
if (jksPassword == null) throw new IllegalArgumentException("Param password cannot be null");
Properties properties = new Properties();
try {
properties.load(credentialsInputStream);
String sharedKeyId = properties.getProperty("sharedKeyId");
String encryptedSharedKey = properties.getProperty("encryptedSharedKey");
String sharedKey;
baseUrl = properties.getProperty("serviceUrl");
KeyStore ks = KeyStore.getInstance(KeyStore.getDefaultType());
ks.load(jksInputStream, jksPassword.toCharArray());
// List the aliases
boolean foundPrivateKey = false;
String alias = keyAlias;
if (alias == null) {
for (; ks.aliases().hasMoreElements(); ) {
String ialias = (String)ks.aliases().nextElement();
foundPrivateKey = ks.isKeyEntry(ialias);
if (foundPrivateKey) {
alias = ialias;
break;
}
}
}
if (alias != null) {
if (keyPassword == null) keyPassword = jksPassword;
Key key = ks.getKey(alias, keyPassword.toCharArray());
Cipher rsa;
rsa = Cipher.getInstance("RSA");
rsa.init(Cipher.DECRYPT_MODE, key);
byte[] data = Base64.decodeBase64(encryptedSharedKey);
byte[] decryptedkey = rsa.doFinal(data);
sharedKey = Base64.encodeBase64String(decryptedkey);
apiKey = new SharedSecretApiKey(sharedKeyId, sharedKey);
}
else {
throw new Exception("Failed to find a private key in the supplied jks file");
}
} catch (Exception ex) {
throw new ClientInitializationException("Failed to initialize the Credential Provider", ex);
}
}
protected void init(KeyStore keyStore, InputStream credentialsInputStream, String keyAlias, String keyPassword) throws ClientInitializationException {
if (keyStore == null) throw new IllegalArgumentException("Param keyStore cannot be null");
if (credentialsInputStream == null) throw new IllegalArgumentException("Param credentialsInputStream cannot be null");
Properties properties = new Properties();
try {
properties.load(credentialsInputStream);
String sharedKeyId = properties.getProperty("sharedKeyId");
String encryptedSharedKey = properties.getProperty("encryptedSharedKey");
String sharedKey;
baseUrl = properties.getProperty("serviceUrl");
// List the aliases
boolean foundPrivateKey = false;
String alias = keyAlias;
if (alias == null) {
for (; keyStore.aliases().hasMoreElements(); ) {
String ialias = (String)keyStore.aliases().nextElement();
foundPrivateKey = keyStore.isKeyEntry(ialias);
if (foundPrivateKey) {
alias = ialias;
break;
}
}
}
if (alias != null) {
Key key = keyStore.getKey(alias, keyPassword.toCharArray());
Cipher rsa;
rsa = Cipher.getInstance("RSA");
rsa.init(Cipher.DECRYPT_MODE, key);
byte[] data = Base64.decodeBase64(encryptedSharedKey);
byte[] decryptedkey = rsa.doFinal(data);
sharedKey = Base64.encodeBase64String(decryptedkey);
apiKey = new SharedSecretApiKey(sharedKeyId, sharedKey);
}
else {
throw new Exception("Failed to find a private key in the supplied jks file");
}
} catch (Exception ex) {
throw new ClientInitializationException("Failed to initialize the Credential Provider", ex);
}
}
@Override
public ITokenKey getApiKey() {
return apiKey;
}
public void setApiKey(ITokenKey apiKey) {
this.apiKey = apiKey;
}
@Override
public String getBaseUrl() {
return baseUrl;
}
public void setBaseUrl(String baseUrl) {
this.baseUrl = baseUrl;
}
@Override
public IApiKey getResponseApiKey() {
if (responseApiKey == null) return apiKey;
return responseApiKey;
}
public void setResponseApiKey(IApiKey responseApiKey) {
this.responseApiKey = responseApiKey;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy