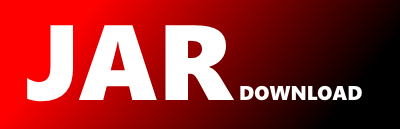
com.identityx.clientSDK.base.BaseRepoFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IdentityXClientSDK Show documentation
Show all versions of IdentityXClientSDK Show documentation
Client SDK for IdentityX Rest Services
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.identityx.clientSDK.base;
import java.io.IOException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import javax.crypto.BadPaddingException;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import com.daon.identityx.rest.model.pojo.Token;
import com.identityx.auth.def.IApiKey;
import com.identityx.auth.impl.Proxy;
import com.identityx.auth.impl.keys.ADCredentialsKey;
import com.identityx.auth.impl.keys.SharedSecretApiKey;
import com.identityx.clientSDK.def.ICredentialsProvider;
import com.identityx.clientSDK.exceptions.IdxRestException;
import com.identityx.clientSDK.repositories.SessionTokenRepository;
/**
* Base class for the {@link com.identityx.clientSDK.SystemRepoFactory} and {link com.identityx.clientSDK.TenantRepoFactory}
*
*
*/
public abstract class BaseRepoFactory {
private String cookie = null;
protected SessionTokenRepository sessionTokenRepo = new SessionTokenRepository();
protected String defaultCn = "daon.com";
private RestClient restClient = new RestClient();
/**
* Use this to configure the RestClient
* @return
*/
public RestClient getRestClient() {
return restClient;
}
/**
* A RestClient object is created by default, you should not need to call this method.
* @param restClient
*/
public void setRestClient(RestClient restClient) {
this.restClient = restClient;
}
/**
* Gets a session token
* @return a session token in the form of {@link SharedSecretApiKey}
* @throws IdxRestException
*/
protected SharedSecretApiKey getSessionKey() throws IdxRestException {
KeyHelper keyHelper;
SharedSecretApiKey newApiKey = null;
try {
keyHelper = new KeyHelper();
Token newToken = new Token();
newToken.setPublicKey(keyHelper.getPublicKeyForTokenRequest(defaultCn));
newToken = sessionTokenRepo.create(newToken);
newApiKey = keyHelper.createFromToken(newToken);
setCookie("idx=" + newToken.getCookie());
} catch (NoSuchAlgorithmException | InvalidKeyException | NoSuchPaddingException | IllegalBlockSizeException | BadPaddingException | IOException e) {
throw new IdxRestException("Failed to get a session key", e);
}
return newApiKey;
}
public String getCookie() {
return cookie;
}
public void setCookie(String cookie) {
this.cookie = cookie;
}
public SessionTokenRepository getSessionTokenRepo() {
return sessionTokenRepo;
}
protected abstract void initRepos(RestClient restClient, String baseUrl);
protected SharedSecretApiKey initSessionAccessToken(IApiKey apiKey, String baseUrl, Proxy proxy, SSLConnectionSocketFactory sslCSF) throws IdxRestException {
SharedSecretApiKey ssKey = null;
if (apiKey instanceof ADCredentialsKey) {
getSessionTokenRepo().getRestClient().setProxy(proxy);
getSessionTokenRepo().init(apiKey, baseUrl, getCookie(), sslCSF);
ssKey = getSessionKey(); // this will create the cookie
}
else if (apiKey instanceof SharedSecretApiKey) {
ssKey = (SharedSecretApiKey)apiKey;
}
else {
throw new IllegalArgumentException("apiKey");
}
return ssKey;
}
protected void init(IApiKey apiKey, String baseUrl, Proxy proxy, SSLConnectionSocketFactory sslCSF) throws IdxRestException {
SharedSecretApiKey ssKey = initSessionAccessToken(apiKey, baseUrl, proxy, sslCSF);
initRestClient(ssKey, proxy, sslCSF);
initRepos(getRestClient(), baseUrl);
}
protected RestClient initRestClient(IApiKey apiKey, Proxy proxy, SSLConnectionSocketFactory sslCSF) {
restClient.init(apiKey, sslCSF);
restClient.setProxy(proxy);
restClient.setCookie(getCookie());
return restClient;
}
public static abstract class RepoFactoryBuilder {
private String baseUrl;
private RestClient restClient;
private boolean createBrowserSession = false;
private ICredentialsProvider credentialProvider;
public RepoFactoryBuilder() {
}
public RepoFactoryBuilder setRestClient(RestClient restClient) {
this.restClient = restClient;
return this;
}
public RepoFactoryBuilder setCredentialsProvider(ICredentialsProvider credentialProvider) {
this.credentialProvider = credentialProvider;
return this;
}
public RepoFactoryBuilder setCreateBrowserSession(boolean createBrowserSession) {
this.createBrowserSession = createBrowserSession;
return this;
}
public RepoFactoryBuilder setBaseUrl(String baseUrl) {
this.baseUrl = baseUrl;
return this;
}
protected abstract BaseRepoFactory build() throws IdxRestException;
protected BaseRepoFactory build(BaseRepoFactory repoFactory) throws IdxRestException {
if (credentialProvider != null) {
this.baseUrl = credentialProvider.getBaseUrl();
IApiKey apiKey = credentialProvider.getApiKey();
if (restClient != null) {
if (createBrowserSession) {
SharedSecretApiKey newSSKey = null;
if (apiKey instanceof ADCredentialsKey) {
repoFactory.getSessionTokenRepo().init(restClient, baseUrl);
newSSKey = repoFactory.getSessionKey(); // this will create the cookie
}
else if (apiKey instanceof SharedSecretApiKey) {
newSSKey = (SharedSecretApiKey)apiKey;
}
else {
throw new IllegalArgumentException("apiKey");
}
RestClient newRestClient = new RestClient.RestClientBuilder().setApiKey(newSSKey)
.setCookie(repoFactory.getCookie())
.setObjectMapper(restClient.getObjectMapper())
.setProxy(restClient.getProxy())
.setSSLConnectionSocketFactory(restClient.getSSLConnectionSocketFactory())
.setRequestExecutor(restClient.getRequestExecutor())
.build();
repoFactory.initRepos(newRestClient, baseUrl);
}
else {
repoFactory.initRepos(restClient, baseUrl);
}
return repoFactory;
}
else {
RestClient newRestClient = new RestClient.RestClientBuilder().setApiKey(apiKey).build();
if (createBrowserSession) {
SharedSecretApiKey newSSKey = null;
if (apiKey instanceof ADCredentialsKey) {
repoFactory.getSessionTokenRepo().init(newRestClient, baseUrl);
newSSKey = repoFactory.getSessionKey(); // this will create the cookie
newRestClient.setCookie(repoFactory.getCookie());
}
else if (apiKey instanceof SharedSecretApiKey) {
newSSKey = (SharedSecretApiKey)apiKey;
}
else {
throw new IllegalArgumentException("apiKey");
}
newRestClient = new RestClient.RestClientBuilder().setApiKey(newSSKey).setCookie(repoFactory.getCookie()).build();
}
repoFactory.initRepos(newRestClient, baseUrl);
return repoFactory;
}
}
else {
if (restClient == null || restClient.getRequestExecutor() == null) {
throw new IllegalStateException("Cannot build without a restClient or restClient.requestExecutor");
}
if (baseUrl == null) {
throw new IllegalStateException("Cannot build without a baseUrl");
}
if (createBrowserSession) {
IApiKey apiKey = restClient.getApiKey();
RestClient newRestClient = new RestClient.RestClientBuilder().setApiKey(apiKey).build();
SharedSecretApiKey newSSKey = null;
if (apiKey instanceof ADCredentialsKey) {
repoFactory.getSessionTokenRepo().init(newRestClient, baseUrl);
newSSKey = repoFactory.getSessionKey(); // this will create the cookie
newRestClient.setCookie(repoFactory.getCookie());
}
else if (apiKey instanceof SharedSecretApiKey) {
newSSKey = (SharedSecretApiKey)apiKey;
}
else {
throw new IllegalArgumentException("apiKey");
}
newRestClient = new RestClient.RestClientBuilder().setApiKey(newSSKey).setCookie(repoFactory.getCookie()).build();
repoFactory.initRepos(newRestClient, baseUrl);
}
else {
repoFactory.initRepos(restClient, baseUrl);
}
return repoFactory;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy