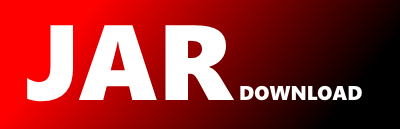
com.identityx.clientSDK.SystemRepoFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IdentityXClientSDK Show documentation
Show all versions of IdentityXClientSDK Show documentation
Client SDK for IdentityX Rest Services
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.identityx.clientSDK;
import java.security.KeyStore;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import com.identityx.auth.impl.DefaultSSLConnectionSocketFactoryProvider;
import com.identityx.auth.impl.Proxy;
import com.identityx.clientSDK.base.BaseRepoFactory;
import com.identityx.clientSDK.base.RestClient;
import com.identityx.clientSDK.def.ICredentialsProvider;
import com.identityx.clientSDK.exceptions.IdxRestException;
import com.identityx.clientSDK.repositories.AuditRepository;
import com.identityx.clientSDK.repositories.ConfigurationRepository;
import com.identityx.clientSDK.repositories.StatisticRepository;
import com.identityx.clientSDK.repositories.SystemAuthenticatorTypeRepository;
import com.identityx.clientSDK.repositories.RoleRepository;
import com.identityx.clientSDK.repositories.TenantRepository;
import com.identityx.clientSDK.repositories.TokenRepository;
/**
* Class used to get repositories related to the system.
* Once this class is created and initialised correctly, the repositories are provided configured and ready to use.
* In order to use the system repositories a system account is required, that means the token or the Active Directory credentials used for access
* need to have system level permissions.
*
*/
public class SystemRepoFactory extends BaseRepoFactory {
private AuditRepository auditRepo = new AuditRepository();
private SystemAuthenticatorTypeRepository authenticatorTypeRepo = new SystemAuthenticatorTypeRepository();
private RoleRepository roleRepo = new RoleRepository();
private TenantRepository tenantRepo = new TenantRepository();
private TokenRepository tokenRepo = new TokenRepository();
private ConfigurationRepository configRepo = new ConfigurationRepository();
private StatisticRepository statisticRepo = new StatisticRepository();
public static class SystemRepoFactoryBuilder extends BaseRepoFactory.RepoFactoryBuilder {
public SystemRepoFactoryBuilder() { }
public SystemRepoFactoryBuilder setRestClient(RestClient restClient) {
super.setRestClient(restClient);
return this;
}
public SystemRepoFactoryBuilder setCredentialsProvider(ICredentialsProvider credentialProvider) {
super.setCredentialsProvider(credentialProvider);
return this;
}
public SystemRepoFactoryBuilder setCreateBrowserSession(boolean createBrowserSession) {
super.setCreateBrowserSession(createBrowserSession);
return this;
}
public SystemRepoFactoryBuilder setBaseUrl(String baseUrl) {
super.setBaseUrl(baseUrl);
return this;
}
public SystemRepoFactory build() throws IdxRestException {
SystemRepoFactory systemRepoFactory = new SystemRepoFactory();
return (SystemRepoFactory)super.build(systemRepoFactory);
}
}
protected SystemRepoFactory() { }
/**
* Creates a new instance of the SystemRepoFactory and initialises it with a credential provider {@link ICredentialsProvider}
* @param credentialProvider {@link ICredentialsProvider}. Needs to contain the system url and credentials that have the correct permissions.
* @throws IdxRestException
*/
public SystemRepoFactory(ICredentialsProvider credentialProvider) throws IdxRestException {
if (credentialProvider == null) throw new IllegalArgumentException("Missing credentialProvider");
init(credentialProvider.getApiKey(), credentialProvider.getResponseApiKey(), credentialProvider.getBaseUrl(), null, null);
}
/**
* Creates a new instance of the TenantRepoFactory and initialises it with a credential provider {@link ICredentialsProvider} and a proxy for the http connection.
* @param credentialProvider {@link ICredentialsProvider}. Needs to contain the system url and credentials that have the correct permissions.
* @param proxy {@link Proxy} for the http connection
* @throws IdxRestException
*/
public SystemRepoFactory(ICredentialsProvider credentialProvider, Proxy proxy) throws IdxRestException {
if (credentialProvider == null) throw new IllegalArgumentException("Missing credentialProvider");
init(credentialProvider.getApiKey(), credentialProvider.getResponseApiKey(), credentialProvider.getBaseUrl(), proxy, null);
}
/**
* Creates a new instance of the TenantRepoFactory and initialises it with a credential provider {@link ICredentialsProvider}, a proxy for the http connection
* and the ssl connection details.
* @param credentialProvider {@link ICredentialsProvider}. Needs to contain the system url and credentials that have the correct permissions.
* @param proxy {@link Proxy} for the http connection
* @param sslCSF {@link SSLConnectionSocketFactory} configured SSLConnectionSocketFactory.
* @throws IdxRestException
*/
public SystemRepoFactory(ICredentialsProvider credentialProvider, Proxy proxy, SSLConnectionSocketFactory sslCSF) throws IdxRestException {
if (credentialProvider == null) throw new IllegalArgumentException("Missing credentialProvider");
init(credentialProvider.getApiKey(), credentialProvider.getResponseApiKey(), credentialProvider.getBaseUrl(), proxy, sslCSF);
}
protected void initRepos(RestClient restClient, String baseUrl) {
this.setRestClient(restClient);
auditRepo.init(restClient, baseUrl);
authenticatorTypeRepo.init(restClient, baseUrl);
roleRepo.init(restClient, baseUrl);
tenantRepo.init(restClient, baseUrl);
tokenRepo.init(restClient, baseUrl);
configRepo.init(restClient, baseUrl);
statisticRepo.init(restClient, baseUrl);
//getSessionTokenRepo().init(ssKey, baseUrl, "");
}
/**
* Handy method to use for https connections: creates a new instance of the TenantRepoFactory and initialises it with a
* credential provider {@link ICredentialsProvider}, a custom trust keystore
* @param credentialProvider
* @param trustStore
* @throws IdxRestException
*/
public SystemRepoFactory(ICredentialsProvider credentialProvider, KeyStore trustStore) throws IdxRestException {
SSLConnectionSocketFactory sslCSF = null;
if (credentialProvider == null) throw new IllegalArgumentException("Missing credentialProvider");
DefaultSSLConnectionSocketFactoryProvider sslCSFP = new DefaultSSLConnectionSocketFactoryProvider();
sslCSF = sslCSFP.get(trustStore, null);
init(credentialProvider.getApiKey(), credentialProvider.getResponseApiKey(), credentialProvider.getBaseUrl(), null, sslCSF);
}
/**
*
* @return {@link AuditRepository} for the system
*/
public AuditRepository getAuditRepo() {
return auditRepo;
}
/**
*
* @return {@link SystemAuthenticatorTypeRepository} for the system
*/
public SystemAuthenticatorTypeRepository getAuthenticatorTypeRepo() {
return authenticatorTypeRepo;
}
/**
*
* @return {@link RoleRepository} for the system
*/
public RoleRepository getRoleRepo() {
return roleRepo;
}
/**
*
* @return {@link TenantRepository} for the system
*/
public TenantRepository getTenantRepo() {
return tenantRepo;
}
/**
*
* @return {@link TokenRepository} for the system
*/
public TokenRepository getTokenRepo() {
return tokenRepo;
}
/**
*
* @return {@link ConfigurationRepository} for the system
*/
public ConfigurationRepository getConfigRepo() {
return configRepo;
}
/**
*
* @return {@link StatisticRepository} for the required tenant
*/
public StatisticRepository getStatisticRepo() {
return statisticRepo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy