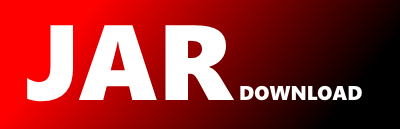
com.identityx.clientSDK.TenantRepoFactory Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.identityx.clientSDK;
import java.security.KeyStore;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import com.identityx.auth.impl.DefaultSSLConnectionSocketFactoryProvider;
import com.identityx.auth.impl.Proxy;
import com.identityx.clientSDK.base.BaseRepoFactory;
import com.identityx.clientSDK.base.RestClient;
import com.identityx.clientSDK.def.ICredentialsProvider;
import com.identityx.clientSDK.exceptions.IdxRestException;
import com.identityx.clientSDK.repositories.ApplicationRepository;
import com.identityx.clientSDK.repositories.AuditRepository;
import com.identityx.clientSDK.repositories.AuthenticationRequestRepository;
import com.identityx.clientSDK.repositories.AuthenticatorRepository;
import com.identityx.clientSDK.repositories.AuthenticatorTypeRepository;
import com.identityx.clientSDK.repositories.ConfigurationRepository;
import com.identityx.clientSDK.repositories.FidoAAIDStatisticRepository;
import com.identityx.clientSDK.repositories.MetadataRepository;
import com.identityx.clientSDK.repositories.PolicyRepository;
import com.identityx.clientSDK.repositories.RegistrationChallengeRepository;
import com.identityx.clientSDK.repositories.RegistrationRepository;
import com.identityx.clientSDK.repositories.RoleRepository;
import com.identityx.clientSDK.repositories.SponsorshipRepository;
import com.identityx.clientSDK.repositories.StatisticRepository;
import com.identityx.clientSDK.repositories.TokenRepository;
import com.identityx.clientSDK.repositories.UserRepository;
/**
* Class used to get repositories related to a tenant.
* Once this class is created and initialised correctly, the repositories are provided configured and ready to use.
*
*/
public class TenantRepoFactory extends BaseRepoFactory {
private ApplicationRepository applicationRepo = new ApplicationRepository();
private AuditRepository auditRepo = new AuditRepository();
private AuthenticationRequestRepository authenticationRequestRepo = new AuthenticationRequestRepository();
private AuthenticatorRepository authenticatorRepo = new AuthenticatorRepository();
private PolicyRepository policyRepo = new PolicyRepository();
private RegistrationRepository registrationRepo = new RegistrationRepository();
private RegistrationChallengeRepository registrationChallengeRepo = new RegistrationChallengeRepository();
private RoleRepository roleRepo = new RoleRepository();
private SponsorshipRepository sponsorshipRepo = new SponsorshipRepository();
private AuthenticatorTypeRepository authenticatorTypeRepo = new AuthenticatorTypeRepository();
private TokenRepository tokenRepo = new TokenRepository();
private UserRepository userRepo = new UserRepository();
private ConfigurationRepository configRepo = new ConfigurationRepository();
private StatisticRepository statisticRepo = new StatisticRepository();
private FidoAAIDStatisticRepository fidoAAIDStatisticRepo = new FidoAAIDStatisticRepository();
private MetadataRepository metadataRepo = new MetadataRepository();
public static class TenantRepoFactoryBuilder extends BaseRepoFactory.RepoFactoryBuilder {
public TenantRepoFactoryBuilder() { }
public TenantRepoFactoryBuilder setRestClient(RestClient restClient) {
super.setRestClient(restClient);
return this;
}
public TenantRepoFactoryBuilder setCredentialsProvider(ICredentialsProvider credentialProvider) {
super.setCredentialsProvider(credentialProvider);
return this;
}
public TenantRepoFactoryBuilder setCreateBrowserSession(boolean createBrowserSession) {
super.setCreateBrowserSession(createBrowserSession);
return this;
}
public TenantRepoFactoryBuilder setBaseUrl(String baseUrl) {
super.setBaseUrl(baseUrl);
return this;
}
public TenantRepoFactory build() throws IdxRestException {
TenantRepoFactory tenantRepoFactory = new TenantRepoFactory();
return (TenantRepoFactory)super.build(tenantRepoFactory);
}
}
protected TenantRepoFactory() {
}
/**
* Creates a new instance of the TenantRepoFactory and initialises it with a credential provider {@link ICredentialsProvider}
* @param credentialProvider {@link ICredentialsProvider}. Needs to contain a tenant url and credentials that have the correct permissions for the required tenant.
* This can be used for SSL as well (except mutual auth), as long as the server's cert is trusted by default (the signing certs are present in cacerts file)
* @throws IdxRestException
*/
public TenantRepoFactory(ICredentialsProvider credentialProvider) throws IdxRestException {
if (credentialProvider == null) throw new IllegalArgumentException("Missing credentialProvider");
init(credentialProvider.getApiKey(), credentialProvider.getResponseApiKey(), credentialProvider.getBaseUrl(), null, null, true);
}
/**
* Creates a new instance of the TenantRepoFactory and initialises it with a credential provider {@link ICredentialsProvider} and a proxy for the http connection.
* @param credentialProvider {@link ICredentialsProvider}. Needs to contain a tenant url and credentials that have the correct permissions for the required tenant.
* @param proxy {@link Proxy} for the http connection
* @throws IdxRestException
*/
public TenantRepoFactory(ICredentialsProvider credentialProvider, Proxy proxy) throws IdxRestException {
if (credentialProvider == null) throw new IllegalArgumentException("Missing credentialProvider");
init(credentialProvider.getApiKey(), credentialProvider.getResponseApiKey(), credentialProvider.getBaseUrl(), proxy, null, true);
}
/**
* Creates a new instance of the TenantRepoFactory and initialises it with a credential provider {@link ICredentialsProvider}, a proxy for the http connection
* and the ssl connection details.
* @param credentialProvider {@link ICredentialsProvider}. Needs to contain a tenant url and credentials that have the correct permissions for the required tenant.
* @param proxy {@link Proxy} for the http connection
* @param sslCSF {@link SSLConnectionSocketFactory} configured SSLConnectionSocketFactory.
* @throws IdxRestException
*/
public TenantRepoFactory(ICredentialsProvider credentialProvider, Proxy proxy, SSLConnectionSocketFactory sslCSF) throws IdxRestException {
if (credentialProvider == null) throw new IllegalArgumentException("Missing credentialProvider");
init(credentialProvider.getApiKey(), credentialProvider.getResponseApiKey(), credentialProvider.getBaseUrl(), proxy, sslCSF, true);
}
protected void initRepos(RestClient restClient, String baseUrl) {
this.setRestClient(restClient);
applicationRepo.init(restClient, baseUrl);
auditRepo.init(restClient, baseUrl);
authenticationRequestRepo.init(restClient, baseUrl);
authenticatorRepo.init(restClient, baseUrl);
policyRepo.init(restClient, baseUrl);
registrationRepo.init(restClient, baseUrl);
registrationChallengeRepo.init(restClient, baseUrl);
roleRepo.init(restClient, baseUrl);
sponsorshipRepo.init(restClient, baseUrl);
authenticatorTypeRepo.init(restClient, baseUrl);
tokenRepo.init(restClient, baseUrl);
//userRepo.getRestClient().setProxy(proxy);
//userRepo.init(ssKey, baseUrl, getCookie(), sslCSF);
userRepo.init(restClient, baseUrl);
configRepo.init(restClient, baseUrl);
statisticRepo.init(restClient, baseUrl);
fidoAAIDStatisticRepo.init(restClient, baseUrl);
metadataRepo.init(restClient, baseUrl);
}
/**
* Handy method to use for https connections: creates a new instance of the TenantRepoFactory and initialises it with a
* credential provider {@link ICredentialsProvider}, a custom trust keystore
* @param credentialProvider
* @param trustStore
* @throws IdxRestException
*/
public TenantRepoFactory(ICredentialsProvider credentialProvider, KeyStore trustStore) throws IdxRestException {
SSLConnectionSocketFactory sslCSF = null;
if (credentialProvider == null) throw new IllegalArgumentException("Missing credentialProvider");
DefaultSSLConnectionSocketFactoryProvider sslCSFP = new DefaultSSLConnectionSocketFactoryProvider();
sslCSF = sslCSFP.get(trustStore, null);
init(credentialProvider.getApiKey(), credentialProvider.getResponseApiKey(), credentialProvider.getBaseUrl(), null, sslCSF, true);
}
/**
*
* @return {@link ApplicationRepository} for the required tenant
*/
public ApplicationRepository getApplicationRepo() {
return applicationRepo;
}
/**
*
* @return {@link AuditRepository} for the required tenant
*/
public AuditRepository getAuditRepo() {
return auditRepo;
}
/**
*
* @return {@link AuthenticationRequestRepository} for the required tenant
*/
public AuthenticationRequestRepository getAuthenticationRequestRepo() {
return authenticationRequestRepo;
}
/**
*
* @return {@link AuthenticatorRepository} for the required tenant
*/
public AuthenticatorRepository getAuthenticatorRepo() {
return authenticatorRepo;
}
/**
*
* @return {@link PolicyRepository} for the required tenant
*/
public PolicyRepository getPolicyRepo() {
return policyRepo;
}
/**
*
* @return {@link RegistrationRepository} for the required tenant
*/
public RegistrationRepository getRegistrationRepo() {
return registrationRepo;
}
/**
*
* @return {@link RegistrationChallengeRepository} for the required tenant
*/
public RegistrationChallengeRepository getRegistrationChallengeRepo() {
return registrationChallengeRepo;
}
/**
*
* @return {@link RoleRepository} for the required tenant
*/
public RoleRepository getRoleRepo() {
return roleRepo;
}
/**
*
* @return {@link SponsorshipRepository} for the required tenant
*/
public SponsorshipRepository getSponsorshipRepo() {
return sponsorshipRepo;
}
/**
*
* @return {@link AuthenticatorTypeRepository} for the required tenant
*/
public AuthenticatorTypeRepository getAuthenticatorTypeRepo() {
return authenticatorTypeRepo;
}
/**
*
* @return {@link TokenRepository} for the required tenant
*/
public TokenRepository getTokenRepo() {
return tokenRepo;
}
/**
*
* @return {@link UserRepository} for the required tenant
*/
public UserRepository getUserRepo() {
return userRepo;
}
/**
*
* @return {@link ConfigurationRepository} for the required tenant
*/
public ConfigurationRepository getConfigRepo() {
return configRepo;
}
/**
*
* @return {@link StatisticRepository} for the required tenant
*/
public StatisticRepository getStatisticRepo() {
return statisticRepo;
}
/**
*
* @return {@link StatisticRepository} for the required tenant
*/
public FidoAAIDStatisticRepository getFidoAAIDStatisticRepo() {
return fidoAAIDStatisticRepo;
}
/**
*
* @return {@link MetadataRepository} for the required tenant
*/
public MetadataRepository getMetadataRepo() {
return metadataRepo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy