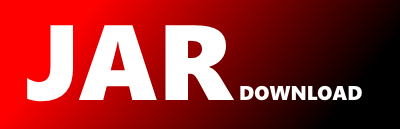
com.identityx.clientSDK.repositories.SessionTokenRepository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IdentityXClientSDK Show documentation
Show all versions of IdentityXClientSDK Show documentation
Client SDK for IdentityX Rest Services
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.identityx.clientSDK.repositories;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import com.daon.identityx.rest.model.def.ResourcePaths;
import com.daon.identityx.rest.model.pojo.Token;
import com.identityx.auth.def.IApiKey;
import com.identityx.clientSDK.collections.TokenCollection;
import com.identityx.clientSDK.exceptions.IdxRestException;
import com.identityx.clientSDK.queryHolders.QueryHolder;
/**
*
* repository for the SessionToken entity. Only create operations are supported on this entity.
* All other operations can be performed on the {@link TokenRepository}
*
*/
public class SessionTokenRepository extends BaseRepository {
public SessionTokenRepository() {
super(Token.class, TokenCollection.class);
setResourcePath(ResourcePaths.defaultSessionTokenPath);
}
@Override
public Token update(Token entity) throws IdxRestException {
throw new UnsupportedOperationException("Use TokenRepository");
}
@Override
public Token get(String appHref) throws IdxRestException {
throw new UnsupportedOperationException("Use TokenRepository");
}
@Override
public TokenCollection list(QueryHolder queryHolder) throws IdxRestException {
throw new UnsupportedOperationException("Use TokenRepository");
}
@Override
public Token block(Token entity) throws IdxRestException {
throw new UnsupportedOperationException("Use TokenRepository");
}
@Override
public Token unblock(Token entity) throws IdxRestException {
throw new UnsupportedOperationException("Use TokenRepository");
}
@Override
public Token archive(Token entity) throws IdxRestException {
throw new UnsupportedOperationException("Use TokenRepository");
}
/**
* Initialises the repository.
*
* @param apiKey used to access the Rest resources
* @param useBasicIDX should use the BasicIDX scheme to pass the authentication information
* @param baseUrl the url of the Rest service, resourcePath is appended to this to create the resource url
* @param sslCSF optional socketFactory
* @param ignoreCookies the HttpClient behind should ignore cookies
*/
public void init(IApiKey apiKey, boolean useBasicIDX, String baseUrl, SSLConnectionSocketFactory sslCSF, boolean ignoreCookies) {
getRestClient().init(apiKey, useBasicIDX, sslCSF, ignoreCookies);
setBaseUrl(baseUrl);
}
/**
* Initialises the repository.
*
* @param apiKey used to access the Rest resources
* @param useBasicIDX should use the BasicIDX scheme to pass the authentication information
* @param baseUrl the url of the Rest service, resourcePath is appended to this to create the resource url
* @param cookie required when the token used is a session token; cookie is obtained at the same time with the session token
* @param resourcePath path of the resource, to be appended to the baseUrl
* @param sslCSF optional socketFactory
*/
public void init(IApiKey apiKey, boolean useBasicIDX, String baseUrl, String cookie, String resourcePath, SSLConnectionSocketFactory sslCSF) {
setResourcePath(resourcePath);
init(apiKey, useBasicIDX, baseUrl, sslCSF, true);
getRestClient().setCookie(cookie);
}
/**
* Initialises the repository.
*
* @param apiKey used to access the Rest resources
* @param useBasicIDX should use the BasicIDX scheme to pass the authentication information
* @param baseUrl url of the Rest service, resourcePath is appended to this to create the resource url
* @param cookie required when the token used is a session token; cookie is obtained at the same time with the session token
* @param sslCSF optional socketFactory
*/
public void init(IApiKey apiKey, boolean useBasicIDX, String baseUrl, String cookie, SSLConnectionSocketFactory sslCSF) {
init(apiKey, useBasicIDX, baseUrl, sslCSF, true);
getRestClient().setCookie(cookie);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy