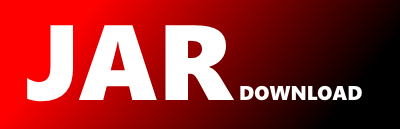
com.identityx.clientSDK.repositories.BaseRepository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of IdentityXClientSDK Show documentation
Show all versions of IdentityXClientSDK Show documentation
Client SDK for IdentityX Rest Services
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.identityx.clientSDK.repositories;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import com.daon.identityx.rest.model.def.IRestResource;
import com.daon.identityx.rest.model.pojo.RestCollection;
import com.identityx.auth.def.IApiKey;
import com.identityx.auth.impl.QueryString;
import com.identityx.clientSDK.base.RestClient;
import com.identityx.clientSDK.exceptions.IdxRestException;
import com.identityx.clientSDK.queryHolders.QueryHolder;
/**
* Base class for creating a repository for a particular entity type.
* A repository is a client abstraction used to access a set of Rest services related to an entity type.
*
* @param type of an entity. Entities implement {@link IRestResource}
* @param type of an entity collection. Entity collections implement {@link com.daon.identityx.rest.model.pojo.RestCollection}
*/
public class BaseRepository, V extends QueryHolder> {
private String baseUrl;
private Class type;
private Class collectionClass;
private String resourcePath;
private RestClient restClient = new RestClient();
/**
*
* @param type entity type
* @param collectionClass entity collection type
*/
public BaseRepository(Class type, Class collectionClass) {
this.type = type;
this.collectionClass = collectionClass;
}
public void init(RestClient restClient, String baseUrl) {
this.restClient = restClient;
this.baseUrl = baseUrl;
}
public void init(RestClient restClient, String baseUrl, String resourcePath) {
this.restClient = restClient;
this.baseUrl = baseUrl;
this.resourcePath = resourcePath;
}
/**
* Initialises the repository.
*
* @param apiKey key used to access the Rest resources
* @param baseUrl the url of the Rest service, resourcePath is appended to this to create the resource url
*/
public void init(IApiKey apiKey, String baseUrl, SSLConnectionSocketFactory sslCSF, boolean ignoreCookies) {
getRestClient().init(apiKey, sslCSF, ignoreCookies);
this.baseUrl = baseUrl;
}
/**
* Initialises the repository.
*
* @param apiKey used to access the Rest resources
* @param baseUrl the url of the Rest service, resourcePath is appended to this to create the resource url
* @param cookie required when the token used is a session token; cookie is obtained at the same time with the session token
* @param resourcePath path of the resource, to be appended to the baseUrl
*/
public void init(IApiKey apiKey, String baseUrl, String cookie, String resourcePath, SSLConnectionSocketFactory sslCSF) {
this.resourcePath = resourcePath;
init(apiKey, baseUrl, sslCSF, true);
getRestClient().setCookie(cookie);
}
/**
* Initialises the repository.
*
* @param apiKey used to access the Rest resources
* @param baseUrl url of the Rest service, resourcePath is appended to this to create the resource url
* @param cookie required when the token used is a session token; cookie is obtained at the same time with the session token
*/
public void init(IApiKey apiKey, String baseUrl, String cookie, SSLConnectionSocketFactory sslCSF) {
init(apiKey, baseUrl, sslCSF, true);
getRestClient().setCookie(cookie);
}
/**
*
* @return url of the Rest service, resourcePath is appended to this to create the resource url
*/
public String getBaseUrl() {
return baseUrl;
}
/**
*
* @param baseUrl url of the Rest service, resourcePath is appended to this to create the resource url
*/
public void setBaseUrl(String baseUrl) {
this.baseUrl = baseUrl;
}
/**
* Creates a new entity
*
* @param entity to create
* @return the created entity
*/
public T create(T entity) throws IdxRestException {
T result = getRestClient().post(entity, getBaseUrl() + "/" + getResourcePath(), type);
return result;
}
/**
* updates an entity
*
* @param entity to update
* @return the updated entity
*/
public T update(T entity) throws IdxRestException {
T result = getRestClient().post(entity, entity.getHref(), type);
return result;
}
/**
* Gets a resource from a specified location
*
* @param href an absolute url of the resource
* @return a resource of type T
*/
public T get(String href) throws IdxRestException {
T result = getRestClient().get(new QueryString(), href, type);
return result;
}
/**
* Gets a resource for the specified unique id
*
* @param id of the resource
* @return a resource of type T
*/
public T getById(String id) throws IdxRestException {
String href = getBaseUrl() + "/" + getResourcePath() + "/" + id;
T result = getRestClient().get(new QueryString(), href, type);
return result;
}
/**
* Gets a resource from a specified location
*
* @param href an absolute url of the resource
* @param queryString an object containing various name-value pairs to be included in the http query string. Can be null null. See {@link QueryString}
* @return a resource of type T
*/
public T get(String href, QueryString queryString) throws IdxRestException {
T result = getRestClient().get(queryString, href, type);
return result;
}
/**
* Lists resources from a specified location
*
* @param href an absolute url of the resource list
* @param queryHolder an object specifying various options for the list operation. See {@link QueryHolder}
* @return a collection of resources of type U, extends {@link RestCollection}
*/
public U list(String href, V queryHolder) throws IdxRestException {
U result = getRestClient().list(queryHolder, href, collectionClass);
return result;
}
/**
* Lists resources from the default location
*
* @param queryHolder an object specifying various options for the list operation. See {@link QueryHolder}
* @return a collection of resources of type U, extends {@link RestCollection}
*/
public U list(V queryHolder) throws IdxRestException {
U result = getRestClient().list(queryHolder, getBaseUrl() + "/" + getResourcePath(), collectionClass);
return result;
}
/**
* Blocks an entity. Blocked entities cannot be updated but can be unblocked.
* Also, entities that have one or more blocked parents cannot be updated either.
*
* @param entity to block
* @return the blocked entity
* @throws IdxRestException
*/
public T block(T entity) throws IdxRestException {
T result = getRestClient().post(null, entity.getHref() + "/blocked", type);
return result;
}
/**
* Unblocks an entity. Will raise an exception if already blocked.
*
* @param entity to be unblocked
* @return the unblocked entity
* @throws IdxRestException
*/
public T unblock(T entity) throws IdxRestException {
T result = getRestClient().delete(entity.getHref() + "/blocked", type);
return result;
}
/**
* Archives an entity. Archived entities become permanently read-only.
*
* @param entity to be archived.
* @return the archived entity.
* @throws IdxRestException
*/
public T archive(T entity) throws IdxRestException {
T result = getRestClient().post(null, entity.getHref() + "/archived", type);
return result;
}
/**
*
* @return path of the resource, to be appended to the baseUrl
*/
public String getResourcePath() {
return resourcePath;
}
/**
*
* @param resourcePath path of the resource, to be appended to the baseUrl
*/
public void setResourcePath(String resourcePath) {
this.resourcePath = resourcePath;
}
/**
*
* @return the {@link RestClient} used to perform the Rest calls
*/
public RestClient getRestClient() {
return restClient;
}
/**
*
* @param restClient {@link RestClient} used to perform the Rest calls
*/
public void setRestClient(RestClient restClient) {
this.restClient = restClient;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy