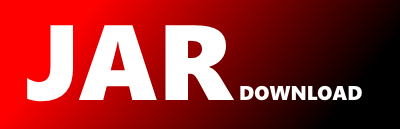
com.identityx.clientSDK.piiSupport.transactionContentProviders.ImageTransactionContentProvider Maven / Gradle / Ivy
package com.identityx.clientSDK.piiSupport.transactionContentProviders;
import java.awt.RenderingHints;
import java.awt.image.BufferedImage;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import javax.imageio.ImageIO;
import org.apache.commons.codec.binary.Base64;
import com.identityx.clientSDK.piiSupport.DisplayPNGCharacteristics;
import com.identityx.clientSDK.piiSupport.PNGUtility;
public class ImageTransactionContentProvider implements ITransactionContentProvider {
private byte[] imageToDisplay = null;
private String textToDisplay = null;
private Map createdContent = new HashMap();
private int defaultWidth = 320;
public int getDefaultWidth() {
return defaultWidth;
}
public void setDefaultWidth(int defaultWidth) {
this.defaultWidth = defaultWidth;
}
public int getDefaultHeight() {
return defaultHeight;
}
public void setDefaultHeight(int defaultHeight) {
this.defaultHeight = defaultHeight;
}
public byte[] getImageContent() {
return imageToDisplay;
}
public String getTextContent() {
return textToDisplay;
}
private int defaultHeight = 320;
public ImageTransactionContentProvider(byte[] imageToDisplay) {
this.imageToDisplay = imageToDisplay;
}
public ImageTransactionContentProvider(String textToDisplay) {
this.textToDisplay = textToDisplay;
}
@Override
public String getContent() {
String content = null;
try {
PNGUtility pngUtility = new PNGUtility();
if (imageToDisplay != null) {
BufferedImage bufferedImage = null;
bufferedImage = ImageIO.read(new ByteArrayInputStream(imageToDisplay));
content = pngUtility.createBase64DatafromPNG(bufferedImage);
}
else {
content = pngUtility.createBase64ImageFromText(new String(Base64.decodeBase64(textToDisplay)), defaultWidth, defaultHeight);
}
}
catch (IOException ex) {
throw new RuntimeException(ex);
}
return content;
}
@Override
public String getContent(DisplayPNGCharacteristics descriptor) {
if (descriptor == null) return getContent();
if (createdContent.containsKey(descriptor)) return createdContent.get(descriptor);
String content = null;
try {
PNGUtility pngUtility = new PNGUtility();
if (imageToDisplay != null) {
BufferedImage scaledImage = null;
BufferedImage bufferedImage = null;
bufferedImage = ImageIO.read(new ByteArrayInputStream(imageToDisplay));
scaledImage = pngUtility.getScaledInstance(bufferedImage, (int)descriptor.getWidth(), (int)descriptor.getHeight(), RenderingHints.VALUE_INTERPOLATION_NEAREST_NEIGHBOR, true);
scaledImage = pngUtility.addTransparentBackground(scaledImage, (int)descriptor.getWidth(), (int)descriptor.getHeight(), true);
content = pngUtility.createBase64DatafromPNG(scaledImage);
}
else {
content = pngUtility.createBase64ImageFromText(new String(Base64.decodeBase64(textToDisplay)), (int)descriptor.getWidth(), (int)descriptor.getHeight());
}
}
catch (IOException ex) {
throw new RuntimeException(ex);
}
createdContent.put(descriptor, content);
return content;
}
public Map getCreatedContent() {
return createdContent;
}
@Override
public boolean isContentTypeSupported(String contentType) {
if (contentType.toLowerCase().equals("image/png")) {
return true;
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy