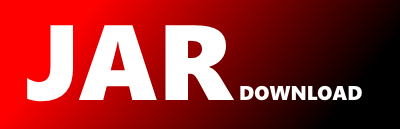
com.daon.identityx.rest.model.pojo.Application Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import java.util.Date;
import com.daon.identityx.rest.model.def.ApplicationStatusEnum;
public class Application extends RestResource
{
private String applicationId;
private byte[] applicationIcon;
private String name;
private String description;
private String primaryFirstName;
private String primaryLastName;
private String primaryEmail;
private String primaryPhone;
private String primaryPhoneCountryCode;
private String primaryAlternatePhone;
private String primaryAlternatePhoneCountryCode;
private String primaryFax;
private String secondaryFirstName;
private String secondaryLastName;
private String secondaryEmail;
private String secondaryPhone;
private String secondaryPhoneCountryCode;
private String secondaryAlternatePhone;
private String secondaryAlternatePhoneCountryCode;
private String secondaryFax;
private Boolean certificateCreated;
private Boolean publishFacetsLocally;
private String addressLine1;
private String addressLine2;
private String city;
private String state;
private String postalCode;
private String country;
private Date updated;
private Date created;
private String fidoApplicationId;
private Date archived;
private ApplicationStatusEnum status;
private byte[] certificate;
private FIDOFacets fidoFacets;
private String rpsaURL;
private Boolean hasApnsKeystore;
private Boolean hasApnsKeystorePassword;
private Boolean hasApnsKeystoreKeyPassword;
private Boolean hasGcmAPIKey;
private Boolean apnsSandboxEnabled;
private Boolean apnsSoundEnabled;
private String gcmURL;
private byte[] apnsKeystore;
private String apnsKeystorePassword;
private String apnsKeystoreKeyPassword;
private String gcmAPIKey;
private String pushNotificationProxyHost;
private String pushNotificationProxyPort;
private String w3cRpId;
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// Links
private Tenant tenant;
private RestCollection users;
private RestCollection registrations;
private RestCollection authenticationRequests;
private RestCollection policies;
private RestCollection sponsorships;
public String getApplicationId() {
return applicationId;
}
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
public byte[] getApplicationIcon() {
return applicationIcon;
}
public void setApplicationIcon(byte[] applicationIcon) {
this.applicationIcon = applicationIcon;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getPrimaryFirstName() {
return primaryFirstName;
}
public void setPrimaryFirstName(String primaryFirstName) {
this.primaryFirstName = primaryFirstName;
}
public String getPrimaryLastName() {
return primaryLastName;
}
public void setPrimaryLastName(String primaryLastName) {
this.primaryLastName = primaryLastName;
}
public String getPrimaryEmail() {
return primaryEmail;
}
public void setPrimaryEmail(String primaryEmail) {
this.primaryEmail = primaryEmail;
}
public String getPrimaryPhone() {
return primaryPhone;
}
public void setPrimaryPhone(String primaryPhone) {
this.primaryPhone = primaryPhone;
}
public String getPrimaryPhoneCountryCode() {
return primaryPhoneCountryCode;
}
public void setPrimaryPhoneCountryCode(String primaryPhoneCountryCode) {
this.primaryPhoneCountryCode = primaryPhoneCountryCode;
}
public String getPrimaryAlternatePhone() {
return primaryAlternatePhone;
}
public void setPrimaryAlternatePhone(String primaryAlternatePhone) {
this.primaryAlternatePhone = primaryAlternatePhone;
}
public String getPrimaryAlternatePhoneCountryCode() {
return primaryAlternatePhoneCountryCode;
}
public void setPrimaryAlternatePhoneCountryCode(
String primaryAlternatePhoneCountryCode) {
this.primaryAlternatePhoneCountryCode = primaryAlternatePhoneCountryCode;
}
public String getPrimaryFax() {
return primaryFax;
}
public void setPrimaryFax(String primaryFax) {
this.primaryFax = primaryFax;
}
public String getSecondaryFirstName() {
return secondaryFirstName;
}
public void setSecondaryFirstName(String secondaryFirstName) {
this.secondaryFirstName = secondaryFirstName;
}
public String getSecondaryLastName() {
return secondaryLastName;
}
public void setSecondaryLastName(String secondaryLastName) {
this.secondaryLastName = secondaryLastName;
}
public String getSecondaryEmail() {
return secondaryEmail;
}
public void setSecondaryEmail(String secondaryEmail) {
this.secondaryEmail = secondaryEmail;
}
public String getSecondaryPhone() {
return secondaryPhone;
}
public void setSecondaryPhone(String secondaryPhone) {
this.secondaryPhone = secondaryPhone;
}
public String getSecondaryPhoneCountryCode() {
return secondaryPhoneCountryCode;
}
public void setSecondaryPhoneCountryCode(String secondaryPhoneCountryCode) {
this.secondaryPhoneCountryCode = secondaryPhoneCountryCode;
}
public String getSecondaryAlternatePhone() {
return secondaryAlternatePhone;
}
public void setSecondaryAlternatePhone(String secondaryAlternatePhone) {
this.secondaryAlternatePhone = secondaryAlternatePhone;
}
public String getSecondaryAlternatePhoneCountryCode() {
return secondaryAlternatePhoneCountryCode;
}
public void setSecondaryAlternatePhoneCountryCode(
String secondaryAlternatePhoneCountryCode) {
this.secondaryAlternatePhoneCountryCode = secondaryAlternatePhoneCountryCode;
}
public String getSecondaryFax() {
return secondaryFax;
}
public void setSecondaryFax(String secondaryFax) {
this.secondaryFax = secondaryFax;
}
public Boolean getCertificateCreated() {
return certificateCreated;
}
public void setCertificateCreated(Boolean certificateCreated) {
this.certificateCreated = certificateCreated;
}
public Boolean getPublishFacetsLocally() {
return publishFacetsLocally;
}
public void setPublishFacetsLocally(Boolean publishFacetsLocally) {
this.publishFacetsLocally = publishFacetsLocally;
}
public Tenant getTenant() {
return tenant;
}
public void setTenant(Tenant tenant) {
this.tenant = tenant;
}
public RestCollection getUsers() {
return users;
}
public void setUsers(RestCollection users) {
this.users = users;
}
public String getAddressLine1() {
return addressLine1;
}
public void setAddressLine1(String addressLine1) {
this.addressLine1 = addressLine1;
}
public String getAddressLine2() {
return addressLine2;
}
public void setAddressLine2(String addressLine2) {
this.addressLine2 = addressLine2;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public String getPostalCode() {
return postalCode;
}
public void setPostalCode(String postalCode) {
this.postalCode = postalCode;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public Date getUpdated() {
return updated;
}
public void setUpdated(Date updatedDate) {
this.updated = updatedDate;
}
public Date getCreated() {
return created;
}
public void setCreated(Date createdDate) {
this.created = createdDate;
}
public String getFidoApplicationId() {
return fidoApplicationId;
}
public void setFidoApplicationId(String fidoApplicationId) {
this.fidoApplicationId = fidoApplicationId;
}
public Date getArchived() {
return archived;
}
public void setArchived(Date archivedDate) {
this.archived = archivedDate;
}
public ApplicationStatusEnum getStatus() {
return status;
}
public void setStatus(ApplicationStatusEnum status) {
this.status = status;
}
public byte[] getCertificate() {
return certificate;
}
public void setCertificate(byte[] certificate) {
this.certificate = certificate;
}
public String getRpsaURL() {
return rpsaURL;
}
public void setRpsaURL(String rpsaURL) {
this.rpsaURL = rpsaURL;
}
public FIDOFacets getFidoFacets() {
return this.fidoFacets;
}
public void setFidoFacets(FIDOFacets facets) {
this.fidoFacets = facets;
}
public Boolean isHasApnsKeystore() {
return hasApnsKeystore;
}
public void setHasApnsKeystore(Boolean hasApnsKeystore) {
this.hasApnsKeystore = hasApnsKeystore;
}
public Boolean isHasApnsKeystorePassword() {
return hasApnsKeystorePassword;
}
public void setHasApnsKeystorePassword(Boolean hasApnsKeystorePassword) {
this.hasApnsKeystorePassword = hasApnsKeystorePassword;
}
public Boolean isHasApnsKeystoreKeyPassword() {
return hasApnsKeystoreKeyPassword;
}
public void setHasApnsKeystoreKeyPassword(Boolean hasApnsKeystoreKeyPassword) {
this.hasApnsKeystoreKeyPassword = hasApnsKeystoreKeyPassword;
}
public Boolean isHasGcmAPIKey() {
return hasGcmAPIKey;
}
public void setHasGcmAPIKey(Boolean hasGcmAPIKey) {
this.hasGcmAPIKey = hasGcmAPIKey;
}
public Boolean isApnsSandboxEnabled() {
return apnsSandboxEnabled;
}
public void setApnsSandboxEnabled(Boolean apnsSandboxEnabled) {
this.apnsSandboxEnabled = apnsSandboxEnabled;
}
public Boolean isApnsSoundEnabled() {
return apnsSoundEnabled;
}
public void setApnsSoundEnabled(Boolean apnsSoundEnabled) {
this.apnsSoundEnabled = apnsSoundEnabled;
}
public RestCollection getRegistrations() {
return registrations;
}
public void setRegistrations(RestCollection registrations) {
this.registrations = registrations;
}
public RestCollection getAuthenticationRequests() {
return authenticationRequests;
}
public void setAuthenticationRequests(
RestCollection authenticationRequests) {
this.authenticationRequests = authenticationRequests;
}
public RestCollection getPolicies() {
return policies;
}
public void setPolicies(RestCollection policies) {
this.policies = policies;
}
public RestCollection getSponsorships() {
return sponsorships;
}
public void setSponsorships(RestCollection sponsorships) {
this.sponsorships = sponsorships;
}
public String getW3cRpId() {
return w3cRpId;
}
public void setW3cRpId(String w3cRpId) {
this.w3cRpId = w3cRpId;
}
public String getGcmURL() {
return gcmURL;
}
public void setGcmURL(String gcmURL) {
this.gcmURL = gcmURL;
}
public byte[] getApnsKeystore() {
return apnsKeystore;
}
public void setApnsKeystore(byte[] apnsKeystore) {
this.apnsKeystore = apnsKeystore;
}
public String getApnsKeystorePassword() {
return apnsKeystorePassword;
}
public void setApnsKeystorePassword(String apnsKeystorePassword) {
this.apnsKeystorePassword = apnsKeystorePassword;
}
public String getApnsKeystoreKeyPassword() {
return apnsKeystoreKeyPassword;
}
public void setApnsKeystoreKeyPassword(String apnsKeystoreKeyPassword) {
this.apnsKeystoreKeyPassword = apnsKeystoreKeyPassword;
}
public String getGcmAPIKey() {
return gcmAPIKey;
}
public void setGcmAPIKey(String gcmAPIKey) {
this.gcmAPIKey = gcmAPIKey;
}
public String getPushNotificationProxyHost() {
return pushNotificationProxyHost;
}
public void setPushNotificationProxyHost(String pushNotificationProxyHost) {
this.pushNotificationProxyHost = pushNotificationProxyHost;
}
public String getPushNotificationProxyPort() {
return pushNotificationProxyPort;
}
public void setPushNotificationProxyPort(String pushNotificationProxyPort) {
this.pushNotificationProxyPort = pushNotificationProxyPort;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy