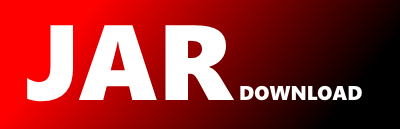
com.daon.identityx.rest.model.pojo.Audit Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import java.util.Date;
import com.daon.identityx.rest.model.support.AuditedError;
public class Audit extends RestResource {
private Date created;
private String request;
private String clientId;
private String clientVersion;
private String authType;
private String authUser;
private Long processingTime;
private String subjectId;
private String result;
private String correlationId;
private String additionalInfo;
private String auditType;
private AuditedError error;
// Links
private Registration registration;
private Tenant tenant;
private Token token;
private Application application;
private RestCollection relatedRecords;
public Date getCreated() {
return created;
}
public void setCreated(Date createdDate) {
this.created = createdDate;
}
public String getRequest() {
return request;
}
public void setRequest(String request) {
this.request = request;
}
public String getClientId() {
return clientId;
}
public void setClientId(String clientId) {
this.clientId = clientId;
}
public String getClientVersion() {
return clientVersion;
}
public void setClientVersion(String clientVersion) {
this.clientVersion = clientVersion;
}
public String getAuthType() {
return authType;
}
public void setAuthType(String authType) {
this.authType = authType;
}
public String getAuthUser() {
return authUser;
}
public void setAuthUser(String authUser) {
this.authUser = authUser;
}
public Long getProcessingTime() {
return processingTime;
}
public void setProcessingTime(Long processingTime) {
this.processingTime = processingTime;
}
public String getSubjectId() {
return subjectId;
}
public void setSubjectId(String subjectId) {
this.subjectId = subjectId;
}
public String getResult() {
return result;
}
public void setResult(String result) {
this.result = result;
}
public String getCorrelationId() {
return correlationId;
}
public void setCorrelationId(String correlationId) {
this.correlationId = correlationId;
}
public String getAdditionalInfo() {
return additionalInfo;
}
public void setAdditionalInfo(String additionalInfo) {
this.additionalInfo = additionalInfo;
}
public String getAuditType() {
return auditType;
}
public void setAuditType(String auditType) {
this.auditType = auditType;
}
public AuditedError getError() {
return error;
}
public void setError(AuditedError error) {
this.error = error;
}
public Token getToken() {
return token;
}
public void setToken(Token token) {
this.token = token;
}
public Application getApplication() {
return application;
}
public void setApplication(Application application) {
this.application = application;
}
public Registration getRegistration() {
return registration;
}
public void setRegistration(Registration registration) {
this.registration = registration;
}
public Tenant getTenant() {
return tenant;
}
public void setTenant(Tenant tenant) {
this.tenant = tenant;
}
public RestCollection getRelatedRecords() {
return relatedRecords;
}
public void setRelatedRecords(RestCollection relatedRecords) {
this.relatedRecords = relatedRecords;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy