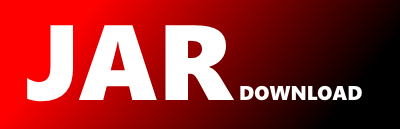
com.daon.identityx.rest.model.pojo.AuthenticationAttemptItem Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import java.util.Date;
import com.daon.identityx.rest.model.support.ChallengeDetail;
import com.daon.identityx.rest.model.support.FIDOChallengeDetail;
import com.daon.identityx.rest.model.support.FaceData;
import com.daon.identityx.rest.model.support.VoiceData;
public class AuthenticationAttemptItem extends RestResource {
private Date created;
private Authenticator authenticator;
private String type;
private Double score;
private Double fmr;
private FaceData[] faceData;
private VoiceData[] voiceData;
private ChallengeDetail challengeDetail;
private FIDOChallengeDetail fidoChallengeDetail;
private String location;
private String result;
private String reason;
private Long authenticatorCounter;
private String aaid;
private DataSample dataSample;
public Date getCreated() {
return created;
}
public void setCreated(Date createdDate) {
this.created = createdDate;
}
public Authenticator getAuthenticator() {
return authenticator;
}
public void setAuthenticator(Authenticator authenticator) {
this.authenticator = authenticator;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public Double getScore() {
return score;
}
public void setScore(Double score) {
this.score = score;
}
public Double getFmr() {
return fmr;
}
public void setFmr(Double fmr) {
this.fmr = fmr;
}
public FaceData[] getFaceData() {
return faceData;
}
public void setFaceData(FaceData[] data) {
this.faceData = data;
}
public VoiceData[] getVoiceData() {
return voiceData;
}
public void setVoiceData(VoiceData[] data) {
this.voiceData = data;
}
public ChallengeDetail getChallengeDetail() {
return challengeDetail;
}
public void setChallengeDetail(ChallengeDetail detail) {
this.challengeDetail = detail;
}
public FIDOChallengeDetail getFidoChallengeDetail() {
return fidoChallengeDetail;
}
public void setFidoChallengeDetail(FIDOChallengeDetail detail) {
this.fidoChallengeDetail = detail;
}
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
public String getResult() {
return result;
}
public void setResult(String result) {
this.result = result;
}
public Long getAuthenticatorCounter() {
return authenticatorCounter;
}
public void setAuthenticatorCounter(Long authenticatorCounter) {
this.authenticatorCounter = authenticatorCounter;
}
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
public String getAaid() {
return aaid;
}
public void setAaid(String aaid) {
this.aaid = aaid;
}
public DataSample getDataSample() {
return dataSample;
}
public void setDataSample(DataSample dataSample) {
this.dataSample = dataSample;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy