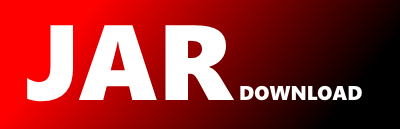
com.daon.identityx.rest.model.pojo.DataSample Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import java.util.Date;
import com.daon.identityx.rest.model.support.DataHolder;
import com.daon.identityx.rest.model.support.DataSampleEvaluation;
public class DataSample extends RestResource {
private DataSampleTypeEnum type;
private String subtype;
private Boolean usable;
private Date lastEvaluated;
private String evaluationResult;
private DataSampleEvaluation[] evaluations;
private Boolean fraudulent;
private Date rejected;
private DataSampleFormatEnum format;
private Date created;
private Date updated;
private DataHolder data;
private DataSampleContext context;
// Links
private Tenant tenant;
private AuthenticationRequest authenticationRequest;
private User user;
private RestCollection enrollments;
public String getSubtype() {
return subtype;
}
public Boolean getUsable() {
return usable;
}
public Date getLastEvaluated() {
return lastEvaluated;
}
public String getEvaluationResult() {
return evaluationResult;
}
public DataSampleEvaluation[] getEvaluations() {
return evaluations;
}
public Boolean getFraudulent() {
return fraudulent;
}
public Date getRejected() {
return rejected;
}
public Date getCreated() {
return created;
}
public Date getUpdated() {
return updated;
}
public DataSampleContext getContext() {
return context;
}
public Tenant getTenant() {
return tenant;
}
public AuthenticationRequest getAuthenticationRequest() {
return authenticationRequest;
}
public User getUser() {
return user;
}
public RestCollection getEnrollments() {
return enrollments;
}
public void setSubtype(String subtype) {
this.subtype = subtype;
}
public void setUsable(Boolean usable) {
this.usable = usable;
}
public void setLastEvaluated(Date lastEvaluated) {
this.lastEvaluated = lastEvaluated;
}
public void setEvaluationResult(String evaluationResult) {
this.evaluationResult = evaluationResult;
}
public void setEvaluations(DataSampleEvaluation[] evaluations) {
this.evaluations = evaluations;
}
public void setFraudulent(Boolean fraudulent) {
this.fraudulent = fraudulent;
}
public void setRejected(Date rejected) {
this.rejected = rejected;
}
public void setCreated(Date created) {
this.created = created;
}
public void setUpdated(Date updated) {
this.updated = updated;
}
public void setContext(DataSampleContext context) {
this.context = context;
}
public void setTenant(Tenant tenant) {
this.tenant = tenant;
}
public void setAuthenticationRequest(AuthenticationRequest authenticationRequest) {
this.authenticationRequest = authenticationRequest;
}
public void setUser(User user) {
this.user = user;
}
public void setEnrollments(RestCollection enrollments) {
this.enrollments = enrollments;
}
public DataSampleTypeEnum getType() {
return type;
}
public DataSampleFormatEnum getFormat() {
return format;
}
public void setType(DataSampleTypeEnum type) {
this.type = type;
}
public void setFormat(DataSampleFormatEnum format) {
this.format = format;
}
public DataHolder getData() {
return data;
}
public void setData(DataHolder data) {
this.data = data;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy