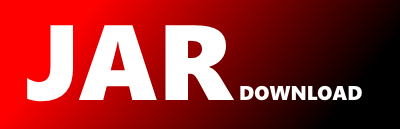
com.daon.identityx.rest.model.pojo.PasscodeCriteria Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
/**
*
* @author pryan
*
*/
public class PasscodeCriteria {
/**
* The value N, restricts a new passcode being the same as any of the past N passcodes.
* The value must be greater or equal to zero. Zero implies no passcode history restriction.
*/
private Integer passcodeHistory;
/**
* Specifies the minimum number of symbols that the users passcode should contain
*/
private Integer requiredSymbolCount;
/**
* Specifies the minimum number of upper case characters that the users passcode should contain
*/
private Integer requiredUpperCaseCount;
/**
* Specifies the minimum number of lower case characters that the users passcode should contain
*/
private Integer requiredLowerCaseCount;
/**
* Specifies the minimum number of digits that the users passcode should contain
*/
private Integer requiredDigitCount;
/**
* Specifies the minimum number of different classes of character that the users passcode
* should contain. The various classes considered are symbols, digits, upper case and lower
* case.
*/
private Integer characterVariety;
/**
* A set of regular expressions. A match of the users passcode on any of these means that
* the users passcode is too weak.
* An entry '\b(.+)\1+\b' prevents repeating patterns.
* Other entries should prevent weak codes such as '1234' or '4321'.
*/
private RegularExpression[] disallowedRegExArray;
/**
* A set of regular expressions. A non-match of the users passcode against any of these
* means that the users passcode does not satisfy passcode criteria.
* An entry of '.{4,256}' requires the users passcode to be between 4 and 256 characters long.
*/
private RegularExpression[] requiredRegExArray;
/**
* An array of regular expressions that define valid patterns for a passcode.
* A passcode must match on at least one of these regular expressions in
* order to be considered valid.
*/
private RegularExpression[] allowedRegExArray;
/**
* Creates a new instance of PasscodeCriteria with no passcode requirements.
*/
public PasscodeCriteria() {
super();
}
/**
* Creates a new instance of PasscodeCriteria with all passcode requirements set.
* If null is specified, the disallowed and required regEx sets will be created as empty sets.
*
* @param passcodeHistory
* @param requiredSymbolCount
* @param requiredUpperCaseCount
* @param requiredLowerCaseCount
* @param requiredDigitCount
* @param characterVariety
* @param disallowedRegExArray
* @param requiredRegExArray
* @param allowedRegExArray
*/
public PasscodeCriteria(Integer passcodeHistory, Integer requiredSymbolCount, Integer requiredUpperCaseCount,
Integer requiredLowerCaseCount, Integer requiredDigitCount, Integer characterVariety,
RegularExpression[] disallowedRegExArray, RegularExpression[] requiredRegExArray,
RegularExpression[] allowedRegExArray) {
this();
this.passcodeHistory = passcodeHistory;
this.requiredSymbolCount = requiredSymbolCount;
this.requiredUpperCaseCount = requiredUpperCaseCount;
this.requiredLowerCaseCount = requiredLowerCaseCount;
this.requiredDigitCount = requiredDigitCount;
this.characterVariety = characterVariety;
this.disallowedRegExArray = disallowedRegExArray;
this.requiredRegExArray = requiredRegExArray;
this.allowedRegExArray = allowedRegExArray;
}
@Override
public String toString() {
return getClass().getName() + "@" + hashCode()
+ "[passcodeHistory=" + passcodeHistory + ", requiredSymbolCount=" + requiredSymbolCount
+ ", requiredUpperCaseCount=" + requiredUpperCaseCount + ", requiredLowerCaseCount=" + requiredLowerCaseCount
+ ", requiredDigitCount=" + requiredDigitCount + ", characterVariety=" + characterVariety
+ ", disallowedRegExArray=" + disallowedRegExArray
+ ", requiredRegExArray=" + requiredRegExArray + ", allowedRegExArray=" + allowedRegExArray + "]";
}
public Integer getPasscodeHistory() {
return passcodeHistory;
}
public void setPasscodeHistory(Integer passcodeHistory) {
this.passcodeHistory = passcodeHistory;
}
public Integer getRequiredSymbolCount() {
return requiredSymbolCount;
}
public void setRequiredSymbolCount(Integer requiredSymbolCount) {
this.requiredSymbolCount = requiredSymbolCount;
}
public Integer getRequiredUpperCaseCount() {
return requiredUpperCaseCount;
}
public void setRequiredUpperCaseCount(Integer requiredUpperCaseCount) {
this.requiredUpperCaseCount = requiredUpperCaseCount;
}
public Integer getRequiredLowerCaseCount() {
return requiredLowerCaseCount;
}
public void setRequiredLowerCaseCount(Integer requiredLowerCaseCount) {
this.requiredLowerCaseCount = requiredLowerCaseCount;
}
public Integer getRequiredDigitCount() {
return requiredDigitCount;
}
public void setRequiredDigitCount(Integer requiredDigitCount) {
this.requiredDigitCount = requiredDigitCount;
}
public Integer getCharacterVariety() {
return characterVariety;
}
public void setCharacterVariety(Integer characterVariety) {
this.characterVariety = characterVariety;
}
public RegularExpression[] getDisallowedRegExArray() {
return disallowedRegExArray;
}
public void setDisallowedRegExArray(RegularExpression[] disallowedRegExArray) {
this.disallowedRegExArray = disallowedRegExArray;
}
public RegularExpression[] getRequiredRegExArray() {
return requiredRegExArray;
}
public void setRequiredRegExArray(RegularExpression[] requiredRegExArray) {
this.requiredRegExArray = requiredRegExArray;
}
public RegularExpression[] getAllowedRegExArray() {
return allowedRegExArray;
}
public void setAllowedRegExArray(RegularExpression[] allowedRegExArray) {
this.allowedRegExArray = allowedRegExArray;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy