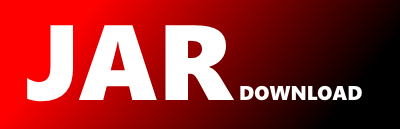
com.daon.identityx.rest.model.pojo.Permission Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
public class Permission {
public static final int CREATE = 1; // 0001
public static final int UPDATE = 2; // 0010
public static final int GET = 4; // 0100
public static final int DELETE = 8; // 1000
public static final int BLOCK = 16; // 1000
public static final int UNBLOCK = 32; // 1000
public static final int ALL_OPERATIONS = CREATE | UPDATE | GET | DELETE | BLOCK | UNBLOCK; // 1111
public static final String operationSeparator = ",";
public static final String entitySeparator = ",";
public static final String entityNameAll = "*";
public static final String operationsAll = "*";
public static final int Application = 1;
public static final int Audit = 2;
public static final int AuthenticationRequest = 4;
public static final int Authenticator = 8;
public static final int SystemAuthenticatorType = 16;
public static final int Policy = 32;
public static final int RegistrationChallenge = 64;
public static final int Registration = 128;
public static final int Role = 256;
public static final int Sponsorship = 512;
public static final int AuthenticatorType = 1024;
public static final int Tenant = 2048;
public static final int Token = 4096;
public static final int User = 8192;
public static final int Configuration = 16384;
public static final int AuthenticationRequestSensitiveData = 32768;
public static final int AuthenticatorSensitiveData = 65536;
public static final int UserSensitiveData = 131072;
public static final int Statistics = 262144;
public static final int SponsorshipSensitiveData = 524288;
public static final int DataSampleSensitiveData = 1048576;
public static final int Enrollment = 2097152;
public static final int DataSample = 4194304;
public static final int All_Entities = 8388607;
public enum EntityNameEnum {
Application,
Audit,
AuthenticationRequest,
Authenticator,
SystemAuthenticatorType,
Policy,
RegistrationChallenge,
Registration,
Role,
Sponsorship,
AuthenticatorType,
Tenant,
Token,
User,
Configuration,
AuthenticationRequestSensitiveData,
AuthenticatorSensitiveData,
UserSensitiveData,
Statistics,
SponsorshipSensitiveData,
DataSampleSensitiveData,
Enrollment,
DataSample
}
public enum OperationTypeEnum {
CREATE,
UPDATE,
DELETE,
GET,
BLOCK,
UNBLOCK,
ALL
}
public static final String permissionPartsSeparator = ":";
private String entity = "*";
private String operation = "*";
private PermissionSelector permissionSelector = new PermissionSelector();
public Permission() { }
public String getEntity() {
return entity;
}
/*
* either EntityNameEnum values separated by "," or "*"
*/
public void setEntity(String entity) {
this.entity = entity;
}
/*
* operation is either a combination of "GET", "CREATE", "UPDATE", "DELETE" separated by ","
* or "*"
*/
public String getOperation() {
return operation.replaceAll("\\s","");
}
/*
* operation is either a combination of "GET", "CREATE", "UPDATE", "DELETE" separated by ","
* or "*"
*/
public void setOperation(String operation) {
this.operation = operation;
}
/*
* helper method
*/
public static String entitiesFromFlags(int entity) {
String value = "";
if ((entity & All_Entities) == All_Entities) {
return "*";
}
if ((entity & Application) == Application) {
value = EntityNameEnum.Application + entitySeparator;
}
if ((entity & Audit) == Audit) {
value = EntityNameEnum.Audit + entitySeparator;
}
if ((entity & AuthenticationRequest) == AuthenticationRequest) {
value = EntityNameEnum.AuthenticationRequest + entitySeparator;
}
if ((entity & Authenticator) == Authenticator) {
value = EntityNameEnum.Authenticator + entitySeparator;
}
if ((entity & SystemAuthenticatorType) == SystemAuthenticatorType) {
value = EntityNameEnum.SystemAuthenticatorType + entitySeparator;
}
if ((entity & Policy) == Policy) {
value = EntityNameEnum.Policy + entitySeparator;
}
if ((entity & RegistrationChallenge) == RegistrationChallenge) {
value = EntityNameEnum.RegistrationChallenge + entitySeparator;
}
if ((entity & Registration) == Registration) {
value = EntityNameEnum.Registration + entitySeparator;
}
if ((entity & Role) == Role) {
value = EntityNameEnum.Role + entitySeparator;
}
if ((entity & Sponsorship) == Sponsorship) {
value = EntityNameEnum.Sponsorship + entitySeparator;
}
if ((entity & AuthenticatorType) == AuthenticatorType) {
value = EntityNameEnum.AuthenticatorType + entitySeparator;
}
if ((entity & Tenant) == Tenant) {
value = EntityNameEnum.Tenant + entitySeparator;
}
if ((entity & Token) == Token) {
value = EntityNameEnum.Token + entitySeparator;
}
if ((entity & User) == User) {
value = EntityNameEnum.User + entitySeparator;
}
if ((entity & Configuration) == Configuration) {
value = EntityNameEnum.Configuration + entitySeparator;
}
if ((entity & AuthenticationRequestSensitiveData) == AuthenticationRequestSensitiveData) {
value = EntityNameEnum.AuthenticationRequestSensitiveData + entitySeparator;
}
if ((entity & AuthenticatorSensitiveData) == AuthenticatorSensitiveData) {
value = EntityNameEnum.AuthenticatorSensitiveData + entitySeparator;
}
if ((entity & UserSensitiveData) == UserSensitiveData) {
value = EntityNameEnum.UserSensitiveData + entitySeparator;
}
if ((entity & SponsorshipSensitiveData) == SponsorshipSensitiveData) {
value = EntityNameEnum.SponsorshipSensitiveData + entitySeparator;
}
if ((entity & DataSampleSensitiveData) == DataSampleSensitiveData) {
value = EntityNameEnum.DataSampleSensitiveData + entitySeparator;
}
if ((entity & Enrollment) == Enrollment) {
value = EntityNameEnum.Enrollment + entitySeparator;
}
if ((entity & DataSample) == DataSample) {
value = EntityNameEnum.DataSample + entitySeparator;
}
if (value != null) {
value = value.substring(0, value.length() - 1);
}
return value;
}
/*
* helper method
*/
public static String operationFromFlags(int operation) {
String value = "";
if ((operation & ALL_OPERATIONS) == ALL_OPERATIONS) {
return "*";
}
if ((operation & CREATE) == CREATE) {
value = OperationTypeEnum.CREATE + operationSeparator;
}
if ((operation & DELETE) == DELETE) {
value += OperationTypeEnum.DELETE + operationSeparator;
}
if ((operation & GET) == GET) {
value += OperationTypeEnum.GET + operationSeparator;
}
if ((operation & UPDATE) == UPDATE) {
value += OperationTypeEnum.UPDATE + operationSeparator;
}
if ((operation & BLOCK) == BLOCK) {
value += OperationTypeEnum.BLOCK + operationSeparator;
}
if ((operation & UNBLOCK) == UNBLOCK) {
value += OperationTypeEnum.UNBLOCK + operationSeparator;
}
if (value != null) {
value = value.substring(0, value.length() - 1);
}
return value;
}
/*
* helper method
*/
public static int flagsFromOperation(String operation) {
int result = 0;
if (operation != null) {
if (operation.equals("*")) {
return ALL_OPERATIONS;
}
String[] parts = operation.split(operationSeparator);
for (String part : parts) {
if (part.equals(OperationTypeEnum.CREATE)) {
result = result | CREATE;
}
if (part.equals(OperationTypeEnum.GET)) {
result = result | GET;
}
if (part.equals(OperationTypeEnum.UPDATE)) {
result = result | UPDATE;
}
if (part.equals(OperationTypeEnum.DELETE)) {
result = result | DELETE;
}
if (part.equals(OperationTypeEnum.BLOCK)) {
result = result | BLOCK;
}
if (part.equals(OperationTypeEnum.UNBLOCK)) {
result = result | UNBLOCK;
}
}
}
return result;
}
public PermissionSelector getPermissionSelector() {
return permissionSelector;
}
public void setPermissionSelector(PermissionSelector permissionSelector) {
this.permissionSelector = permissionSelector;
}
public String toString() {
return getEntity() + permissionPartsSeparator + getOperation() + permissionPartsSeparator + getPermissionSelector().toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy