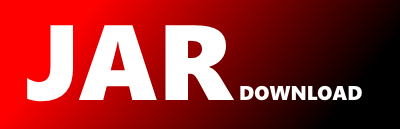
com.daon.identityx.rest.model.pojo.PermissionSelector Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import com.daon.identityx.rest.model.def.ResourcePaths;
public class PermissionSelector {
public enum SelectorNamesEnum {
WITHIN,
GENERIC,
WITHIN_CURRENT_USER,
WITHIN_CURRENT_INSTANCE,
}
private String selectorType = null;
private RestResource resource = null;
protected final static Map selectorTypes;
protected final static Map selectorEntities;
static {
Map aMap = new HashMap();
aMap.put(SelectorNamesEnum.WITHIN.toString(), "__Within");
aMap.put(SelectorNamesEnum.WITHIN_CURRENT_USER.toString(), "__WithinCurrentUser");
aMap.put(SelectorNamesEnum.WITHIN_CURRENT_INSTANCE.toString(), "__WithinCurrentInstance");
aMap.put(SelectorNamesEnum.GENERIC.toString(), "*"); // generic must be *
selectorTypes = Collections.unmodifiableMap(aMap);
aMap = new HashMap();
aMap.put(ResourcePaths.defaultTenantPath, "Tenant");
aMap.put(ResourcePaths.defaultApplicationPath, "Application");
selectorEntities = Collections.unmodifiableMap(aMap);
}
/*
* Creates a generic selector type (*) - includes all entities
*/
public PermissionSelector() {
this.selectorType = SelectorNamesEnum.GENERIC.toString();
}
/*
* Creates a subset selector type - includes all entities associated with the resource specified
* If a tenant is specified, the permission that includes this permissionSelector will only be applied to entities belonging to the tenant
* Possible values: a Tenant or a Application
*/
public PermissionSelector(RestResource resource) {
if (resource == null || resource.getHref() == null) throw new IllegalArgumentException();
String parts[] = resource.getHref().split("/");
if (parts.length < 3) {
throw new IllegalArgumentException("Bad href");
}
String entity = parts[parts.length - 2];
if (!selectorEntities.keySet().contains(entity)) {
throw new IllegalArgumentException("Entity type not allowed or bad href");
}
this.selectorType = SelectorNamesEnum.WITHIN.toString();
this.resource = new RestResource(resource.getHref());
}
public String getSelectorType() {
return selectorType;
}
public void setSelectorType(String selectorType) {
if (selectorTypes.containsKey(selectorType)) {
this.selectorType = selectorType;
}
else {
throw new IllegalArgumentException("A value of " + selectorType + " is invalid");
}
}
public RestResource getResource() {
return resource;
}
public void setResource(RestResource resource) {
this.resource = resource;
}
public String toString() {
if (this.selectorType.equals("GENERIC")) return "*";
if (!selectorTypes.keySet().contains(selectorType)) {
throw new IllegalStateException();
}
if (this.selectorType.equals("WITHIN") && (resource == null || resource.getHref() == null)) {
throw new IllegalStateException();
}
if (resource != null && resource.getHref() != null) {
String parts[] = resource.getHref().split("/");
if (parts.length < 3) {
throw new IllegalStateException();
}
String entity = parts[parts.length - 2];
if (!selectorEntities.keySet().contains(entity)) {
throw new IllegalStateException();
}
String entityId = parts[parts.length - 1];
return selectorTypes.get(selectorType) + selectorEntities.get(entity) + "(" + entityId + ")";
}
return selectorTypes.get(selectorType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy