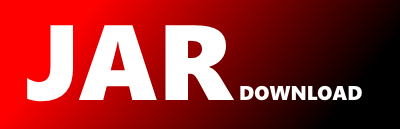
com.daon.identityx.rest.model.pojo.Policy Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import java.util.Date;
import com.daon.identityx.rest.model.def.PolicyStatusEnum;
import com.daon.identityx.rest.model.support.LegacyAuthenticationPolicy;
public class Policy extends RestResource {
public enum PolicyTypeEnum {
/**
* IdentityX enrollment
*/
IE,
/**
* IdentityX authentication
*/
IA,
/**
* FIDO Registration
*/
FR,
/**
* FIDO Authentication
*/
FA,
/**
* FIDO Offline Authentiction
*/
FO,
/**
* W3C Registration
*/
WR,
/**
* W3C Authentication
*/
WA,
/**
* REST Authentication
*/
RA,
/**
* REST Enrollment
*/
RE,
/**
* OTP
*/
OP
}
private String policyId;
private String description;
private Integer otpRetryAttempts;
private String otpConfiguration;
private Integer retryAttempts;
private Date updated;
private Date created;
private Date archived;
private Long timeToLive;
private PolicyTypeEnum type;
private PolicyStatusEnum status;
private LegacyAuthenticationPolicy legacyAuthenticationPolicy;
// data elements
private ActivityDefinition activityDefinition;
// these are not used
private Object factorsDefinition;
private Object scripts;
private FIDOPolicy fidoPolicy;
private OtpPolicy otpPolicy;
private CredentialExtensions credentialExtensions;
private W3CPolicy w3cPolicy;
// Links
private Application application;
private Tenant tenant;
private RestCollection authenticationRequests;
public Policy()
{
super();
}
public Policy(String href)
{
super(href);
}
public Integer getOtpRetryAttempts() {
return otpRetryAttempts;
}
public void setOtpRetryAttempts(Integer otpRetryAttempts) {
this.otpRetryAttempts = otpRetryAttempts;
}
public String getOtpConfiguration() {
return otpConfiguration;
}
public void setOtpConfiguration(String otpConfiguration) {
this.otpConfiguration = otpConfiguration;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getPolicyId() {
return policyId;
}
public void setPolicyId(String policyId) {
this.policyId = policyId;
}
public Integer getRetryAttempts() {
return retryAttempts;
}
public void setRetryAttempts(Integer retryAttempts) {
this.retryAttempts = retryAttempts;
}
public Long getTimeToLive() {
return timeToLive;
}
public void setTimeToLive(Long timeToLive) {
this.timeToLive = timeToLive;
}
public Date getUpdated() {
return updated;
}
public void setUpdated(Date updatedDate) {
this.updated = updatedDate;
}
public Date getCreated() {
return created;
}
public void setCreated(Date createdDate) {
this.created = createdDate;
}
public Date getArchived() {
return archived;
}
public void setArchived(Date archiveDate) {
this.archived = archiveDate;
}
public PolicyTypeEnum getType() {
return type;
}
public void setType(PolicyTypeEnum type) {
this.type = type;
}
public PolicyStatusEnum getStatus() {
return status;
}
public void setStatus(PolicyStatusEnum status) {
this.status = status;
}
public LegacyAuthenticationPolicy getLegacyAuthenticationPolicy() {
return legacyAuthenticationPolicy;
}
public void setLegacyAuthenticationPolicy(
LegacyAuthenticationPolicy legacyAuthenticationPolicy) {
this.legacyAuthenticationPolicy = legacyAuthenticationPolicy;
}
public ActivityDefinition getActivityDefinition() {
return activityDefinition;
}
public void setActivityDefinition(ActivityDefinition activityDefinition) {
this.activityDefinition = activityDefinition;
}
// these are not used, left here for compatibility with SDK 4.0
@Deprecated
public Object getFactorsDefinition() {
return factorsDefinition;
}
@Deprecated
public void setFactorsDefinition(Object factorsDefinition) {
this.factorsDefinition = factorsDefinition;
}
@Deprecated
public Object getScripts() {
return scripts;
}
@Deprecated()
public void setScripts(Object scripts) {
this.scripts = scripts;
}
public FIDOPolicy getFidoPolicy() {
return fidoPolicy;
}
public void setFidoPolicy(FIDOPolicy fidoPolicy) {
this.fidoPolicy = fidoPolicy;
}
public Application getApplication() {
return application;
}
public void setApplication(Application application) {
this.application = application;
}
public Tenant getTenant() {
return tenant;
}
public void setTenant(Tenant tenant) {
this.tenant = tenant;
}
public RestCollection getAuthenticationRequests() {
return authenticationRequests;
}
public void setAuthenticationRequests(RestCollection authenticationRequests) {
this.authenticationRequests = authenticationRequests;
}
public CredentialExtensions getCredentialExtensions() {
return credentialExtensions;
}
public void setCredentialExtensions(CredentialExtensions credentialExtensions) {
this.credentialExtensions = credentialExtensions;
}
public W3CPolicy getW3cPolicy() {
return w3cPolicy;
}
public void setW3cPolicy(W3CPolicy w3cPolicy) {
this.w3cPolicy = w3cPolicy;
}
public OtpPolicy getOtpPolicy() {
return otpPolicy;
}
public void setOtpPolicy(OtpPolicy otpPolicy) {
this.otpPolicy = otpPolicy;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy