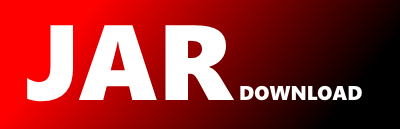
com.daon.identityx.rest.model.pojo.RegistrationChallenge Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import java.util.Date;
import com.daon.identityx.rest.model.def.RegistrationChallengeStatusEnum;
import com.daon.identityx.rest.model.support.RegistrationChallengeAuthData;
public class RegistrationChallenge extends RestResource {
private String challenge;
private String fidoRegistrationRequest;
private String fidoRegistrationResponse;
private String w3cMakeCredentialRequest;
private String w3cMakeCredentialResponse;
private RegistrationChallengeStatusEnum status;
private byte[] tlsCertificate;
private String tlsUnique;
private byte[] cidPublicKey;
private String serverData;
private Long fidoResponseCode;
private String fidoResponseMsg;
private Date created;
private Date updated;
private Date archived;
private String relyingPartyUsername;
private String sponsorshipToken;
private Version upv;
private String deviceStatus;
private Tenant tenant;
private ChannelBinding channelBinding;
private RegistrationChallengeAuthData[] authenticationData;
// Links
private Registration registration;
private Policy policy;
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////
public RegistrationChallenge()
{
super();
}
public RegistrationChallenge(String href)
{
this.setHref(href);
}
public String getChallenge() {
return challenge;
}
public void setChallenge(String challenge) {
this.challenge = challenge;
}
public String getFidoRegistrationRequest() {
return fidoRegistrationRequest;
}
public void setFidoRegistrationRequest(String fidoRegistrationRequest) {
this.fidoRegistrationRequest = fidoRegistrationRequest;
}
public String getFidoRegistrationResponse() {
return fidoRegistrationResponse;
}
public void setFidoRegistrationResponse(String fidoRegistrationResponse) {
this.fidoRegistrationResponse = fidoRegistrationResponse;
}
public RegistrationChallengeStatusEnum getStatus() {
return status;
}
public void setStatus(RegistrationChallengeStatusEnum status) {
this.status = status;
}
public byte[] getTlsCertificate() {
return tlsCertificate;
}
public void setTlsCertificate(byte[] tlsCertificate) {
this.tlsCertificate = tlsCertificate;
}
public String getTlsUnique() {
return tlsUnique;
}
public void setTlsUnique(String tlsUnique) {
this.tlsUnique = tlsUnique;
}
public byte[] getCidPublicKey() {
return cidPublicKey;
}
public void setCidPublicKey(byte[] cidPublicKey) {
this.cidPublicKey = cidPublicKey;
}
public String getServerData() {
return serverData;
}
public void setServerData(String serverData) {
this.serverData = serverData;
}
public Long getFidoResponseCode() {
return fidoResponseCode;
}
public void setFidoResponseCode(Long fidoResponseCode) {
this.fidoResponseCode = fidoResponseCode;
}
public String getFidoResponseMsg() {
return fidoResponseMsg;
}
public void setFidoResponseMsg(String fidoResponseMsg) {
this.fidoResponseMsg = fidoResponseMsg;
}
public Date getCreated() {
return created;
}
public void setCreated(Date createdDate) {
this.created = createdDate;
}
public Date getUpdated() {
return updated;
}
public void setUpdated(Date updatedDate) {
this.updated = updatedDate;
}
public void setArchived(Date inactiveDate) {
this.archived = inactiveDate;
}
public Date getArchived() {
return archived;
}
public void setRegistration(Registration registration) {
this.registration = registration;
}
public Registration getRegistration() {
return registration;
}
public Policy getPolicy() {
return policy;
}
public void setPolicy(Policy policy) {
this.policy = policy;
}
public ChannelBinding getChannelBinding() {
return channelBinding;
}
public void setChannelBinding(ChannelBinding channelBinding) {
this.channelBinding = channelBinding;
}
public Tenant getTenant() {
return tenant;
}
public void setTenant(Tenant tenant) {
this.tenant = tenant;
}
public String getW3cMakeCredentialRequest() {
return w3cMakeCredentialRequest;
}
public void setW3cMakeCredentialRequest(String w3cMakeCredentialRequest) {
this.w3cMakeCredentialRequest = w3cMakeCredentialRequest;
}
public String getW3cMakeCredentialResponse() {
return w3cMakeCredentialResponse;
}
public void setW3cMakeCredentialResponse(String w3cMakeCredentialResponse) {
this.w3cMakeCredentialResponse = w3cMakeCredentialResponse;
}
public String getRelyingPartyUsername() {
return relyingPartyUsername;
}
public void setRelyingPartyUsername(String relyingPartyUsername) {
this.relyingPartyUsername = relyingPartyUsername;
}
public String getDeviceStatus() {
return deviceStatus;
}
public void setDeviceStatus(String deviceStatus) {
this.deviceStatus = deviceStatus;
}
public String getSponsorshipToken() {
return sponsorshipToken;
}
public void setSponsorshipToken(String sponsorshipToken) {
this.sponsorshipToken = sponsorshipToken;
}
public Version getUpv() {
return upv;
}
public void setUpv(Version upv) {
this.upv = upv;
}
public RegistrationChallengeAuthData[] getAuthenticationData() {
return authenticationData;
}
public void setAuthenticationData(RegistrationChallengeAuthData[] authenticationData) {
this.authenticationData = authenticationData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy