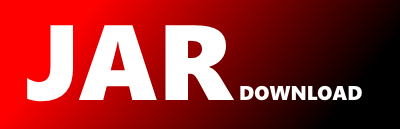
com.daon.identityx.rest.model.pojo.RestCollection Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import com.daon.identityx.rest.model.def.IRestResource;
/**
* Base class used to manage lists of entities that can be accessed at a particular url of the Rest services.
* The url can be simple or followed by a query string specifying various options for retrieving the list like filtering, sorting, paging or expansion of sub-entities
*
*
* @param type of an entity
*/
public class RestCollection implements IRestResource {
private RestSearchMetadata metadata;
private RestPaging paging;
private String href;
private T[] items;
private String requestURL;
private String requestQuery;
private String allowedPermissions;
/**
* @return url of the list resource
*/
public String getHref() {
return href;
}
/**
* @param href url of the list resource
*/
public void setHref(String href) {
this.href = href;
}
/**
*
* @return array of entities
*/
public T[] getItems() {
return items;
}
/**
*
* @param items array of entities
*/
public void setItems(T[] items) {
this.items = items;
}
/**
* @return base url of the list
*/
public String getRequestURL() {
return requestURL;
}
/**
* @param requestURL base url of the list
*/
public void setRequestURL(String requestURL) {
this.requestURL = requestURL;
}
/**
* @return query string used for obtaining the particular list
*/
public String getRequestQuery() {
return requestQuery;
}
/**
* @param requestQuery query string used for obtaining the particular list
*/
public void setRequestQuery(String requestQuery) {
this.requestQuery = requestQuery;
}
/**
* @return permissions the authenticating token used for retrieving the list has on the collection of entities, typically CREATE and/or DELETE.
* This means that the same token can be used to add new entities to the list or delete entities from the list.
*/
public String getAllowedPermissions() {
return allowedPermissions;
}
/**
* @param allowedPermissions permissions the authenticating token used for retrieving the list has on the collection of entities, typically CREATE and/or DELETE.
* This means that the same token can be used to add new entities to the list or delete entities from the list.
*/
public void setAllowedPermissions(String allowedPermissions) {
this.allowedPermissions = allowedPermissions;
}
/**
*
* @return search metadata {@link RestSearchMetadata}
*/
public RestSearchMetadata getMetadata() {
return metadata;
}
/**
* @return search metadata {@link RestSearchMetadata}
*/
public RestPaging getPaging() {
return paging;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy