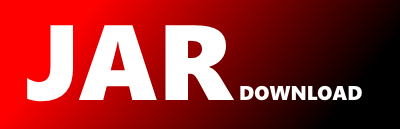
com.daon.identityx.rest.model.pojo.Sponsorship Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import java.util.Date;
import com.daon.identityx.rest.model.def.SponsorshipStatusEnum;
import com.daon.identityx.rest.model.support.FailedFaceData;
import com.daon.identityx.rest.model.support.FailedVoiceData;
public class Sponsorship extends RestResource {
public enum SponsorshipTypeEnum {
USER,
AUTHENTICATOR
}
private String registrationId;
private String userId;
private String authenticationRequestId;
private String sponsorUserId;
private SponsorshipTypeEnum type;
private String sponsorshipToken;
private Date expiration;
private Long availableRetries;
private SponsorshipStatusEnum status;
private Date sponsorship;
private Long totalRetriesAllowed;
private Boolean createRegistrationEnabled;
private Date sponsorshipLastVerified;
private Boolean certificateCreated;
private String clientApplicationIdentifier;
private Date created;
private byte[] qrCode;
private Boolean sponsorshipCompleted;
private Boolean notificationSent;
private String notificationURL;
private Boolean generateProfileId;
private String termsURL;
private String authenticationGatewayURL;
private String rpsaURL;
private FailedFaceData[] failedFace;
private FailedVoiceData[] failedVoiceTextDependent;
private FailedVoiceData[] failedVoiceTextPromptedDigits;
// Links
private Application application;
private Policy policy;
private Tenant tenant;
//////////////////////////////////////////////////////////////////////////////////////
public String getRegistrationId() {
return registrationId;
}
public void setRegistrationId(String registrationId) {
this.registrationId = registrationId;
}
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public String getAuthenticationRequestId() {
return authenticationRequestId;
}
public void setAuthenticationRequestId(String authenticationRequestId) {
this.authenticationRequestId = authenticationRequestId;
}
public String getSponsorUserId() {
return sponsorUserId;
}
public void setSponsorUserId(String sponsorUserId) {
this.sponsorUserId = sponsorUserId;
}
public SponsorshipTypeEnum getType() {
return type;
}
public void setType(SponsorshipTypeEnum type) {
this.type = type;
}
public String getSponsorshipToken() {
return sponsorshipToken;
}
public void setSponsorshipToken(String sponsorshipToken) {
this.sponsorshipToken = sponsorshipToken;
}
public Date getExpiration() {
return expiration;
}
public void setExpiration(Date expirationDate) {
this.expiration = expirationDate;
}
public Long getAvailableRetries() {
return availableRetries;
}
public void setAvailableRetries(Long availableRetries) {
this.availableRetries = availableRetries;
}
public SponsorshipStatusEnum getStatus() {
return status;
}
public void setStatus(SponsorshipStatusEnum status) {
this.status = status;
}
public Date getSponsorship() {
return sponsorship;
}
public void setSponsorship(Date sponsorshipDate) {
this.sponsorship = sponsorshipDate;
}
public Long getTotalRetriesAllowed() {
return totalRetriesAllowed;
}
public void setTotalRetriesAllowed(Long totalRetriesAllowed) {
this.totalRetriesAllowed = totalRetriesAllowed;
}
public Boolean getCreateRegistrationEnabled() {
return createRegistrationEnabled;
}
public void setCreateRegistrationEnabled(Boolean createRegistrationEnabled) {
this.createRegistrationEnabled = createRegistrationEnabled;
}
public Date getSponsorshipLastVerified() {
return sponsorshipLastVerified;
}
public void setSponsorshipLastVerified(Date sponsorshipLastVerifiedDate) {
this.sponsorshipLastVerified = sponsorshipLastVerifiedDate;
}
public Boolean getCertificateCreated() {
return certificateCreated;
}
public void setCertificateCreated(Boolean certificateCreated) {
this.certificateCreated = certificateCreated;
}
public String getClientApplicationIdentifier() {
return clientApplicationIdentifier;
}
public void setClientApplicationIdentifier(String clientApplicationIdentifier) {
this.clientApplicationIdentifier = clientApplicationIdentifier;
}
public Date getCreated() {
return created;
}
public void setCreated(Date createdDate) {
this.created = createdDate;
}
public byte[] getQrCode() {
return qrCode;
}
public void setQrCode(byte[] qrCode) {
this.qrCode = qrCode;
}
public Boolean getSponsorshipCompleted() {
return sponsorshipCompleted;
}
public void setSponsorshipCompleted(Boolean sponsorshipCompleted) {
this.sponsorshipCompleted = sponsorshipCompleted;
}
public Boolean getNotificationSent() {
return notificationSent;
}
public void setNotificationSent(Boolean notificationSent) {
this.notificationSent = notificationSent;
}
public String getNotificationURL() {
return notificationURL;
}
public void setNotificationURL(String notificationURL) {
this.notificationURL = notificationURL;
}
public Boolean getGenerateProfileId() {
return generateProfileId;
}
public void setGenerateProfileId(Boolean generateProfileId) {
this.generateProfileId = generateProfileId;
}
public String getTermsURL() {
return termsURL;
}
public void setTermsURL(String termsURL) {
this.termsURL = termsURL;
}
public Application getApplication() {
return application;
}
public void setApplication(Application application) {
this.application = application;
}
public Policy getPolicy() {
return policy;
}
public void setPolicy(Policy policy) {
this.policy = policy;
}
public Tenant getTenant() {
return tenant;
}
public void setTenant(Tenant tenant) {
this.tenant = tenant;
}
public String getAuthenticationGatewayURL() {
return authenticationGatewayURL;
}
public void setAuthenticationGatewayURL(String authenticationGatewayURL) {
this.authenticationGatewayURL = authenticationGatewayURL;
}
public String getRpsaURL() {
return rpsaURL;
}
public void setRpsaURL(String rpsaURL) {
this.rpsaURL = rpsaURL;
}
public FailedFaceData[] getFailedFace() {
return this.failedFace;
}
public void setFailedFace(FailedFaceData[] face) {
this.failedFace = face;
}
public FailedVoiceData[] getFailedVoiceTextDependent() {
return this.failedVoiceTextDependent;
}
public void setFailedVoiceTextDependent(FailedVoiceData[] voiceSamples) {
this.failedVoiceTextDependent = voiceSamples;
}
public FailedVoiceData[] getFailedVoiceTextPromptedDigits() {
return this.failedVoiceTextPromptedDigits;
}
public void setFailedVoiceTextPromptedDigits(FailedVoiceData[] voiceDigits) {
this.failedVoiceTextPromptedDigits = voiceDigits;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy