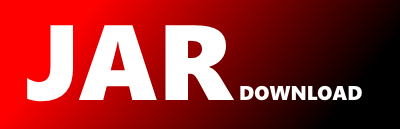
com.daon.identityx.rest.model.pojo.User Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.pojo;
import java.util.Date;
import com.daon.identityx.rest.model.def.UserStatusEnum;
import com.daon.identityx.rest.model.support.FaceData;
import com.daon.identityx.rest.model.support.SecretQuestion;
import com.daon.identityx.rest.model.support.VoiceData;
public class User extends RestResource {
private String userId;
private String externalName;
private String email;
private String firstName;
private String lastName;
private String middleName;
private String prefix;
private String suffix;
private String primaryPhone;
private String primaryPhoneCountryCode;
private String alternatePhone;
private String alternatePhoneCountryCode;
private String addressLine1;
private String addressLine2;
private String city;
private String state;
private String postalCode;
private String country;
private Date archived;
private Date created;
private Date updated;
private String language;
private String speakerId;
private String pin;
private FaceData face;
private VoiceData[] voiceTextDependent;
private VoiceData[] voiceTextPromptedDigits;
private Boolean pinEnrolled;
private Boolean faceEnrolled;
private Boolean voiceEnrolled;
private Boolean voiceDigitsEnrolled;
private Boolean keystrokesEnrolled;
private SecretQuestion[] secretQuestion;
private Long failedVerificationCount;
private Date lockedUntil;
private Long accountUnlockedCount;
private Long adosPasscodeFailedAttempts;
private Long adosPasscodeUnlockCount;
private Date adosPasscodeLockedUntilDtm;
private Long adosFaceFailedAttempts;
private Long adosFaceUnlockCount;
private Date adosFaceLockedUntilDtm;
private Long adosVoiceFailedAttempts;
private Long adosVoiceUnlockCount;
private Date adosVoiceLockedUntilDtm;
private Long otpFailedAttempts;
private Long otpUnlockCount;
private Date otpLockedUntilDtm;
private Long directOtpFailedAttempts;
private Long directOtpUnlockCount;
private Date directOtpLockedUntilDtm;
private Long keystrokesFailedAttempts;
private Long keystrokesUnlockCount;
private Date keystrokesLockedUntilDtm;
private Date nextPossibleDirectOtpDtm;
private UserStatusEnum status;
// Links
private Tenant tenant;
private RestCollection applications;
private RestCollection registrations;
private RestCollection authenticators;
private RestCollection authenticationRequests;
private RestCollection sponsorships;
private RestCollection registrationChallenges;
private RestCollection enrollments;
private RestCollection samples;
public String getUserId() {
return userId;
}
public void setUserId(String value) {
this.userId = value;
}
public String getExternalName() {
return externalName;
}
public void setExternalName(String value) {
this.externalName = value;
}
public String getEmail() {
return email;
}
public void setEmail(String value) {
this.email = value;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String value) {
this.firstName = value;
}
public String getLastName() {
return lastName;
}
public void setLastName(String value) {
this.lastName = value;
}
public String getMiddleName() {
return middleName;
}
public void setMiddleName(String value) {
this.middleName = value;
}
public String getPrefix() {
return prefix;
}
public void setPrefix(String value) {
this.prefix = value;
}
public String getSuffix() {
return suffix;
}
public void setSuffix(String value) {
this.suffix = value;
}
public String getPrimaryPhone() {
return primaryPhone;
}
public void setPrimaryPhone(String value) {
this.primaryPhone = value;
}
public String getPrimaryPhoneCountryCode() {
return primaryPhoneCountryCode;
}
public void setPrimaryPhoneCountryCode(String value) {
this.primaryPhoneCountryCode = value;
}
public String getAlternatePhone() {
return alternatePhone;
}
public void setAlternatePhone(String value) {
this.alternatePhone = value;
}
public String getAlternatePhoneCountryCode() {
return alternatePhoneCountryCode;
}
public void setAlternatePhoneCountryCode(String value) {
this.alternatePhoneCountryCode = value;
}
public String getAddressLine1() {
return addressLine1;
}
public void setAddressLine1(String value) {
this.addressLine1 = value;
}
public String getAddressLine2() {
return addressLine2;
}
public void setAddressLine2(String value) {
this.addressLine2 = value;
}
public String getCity() {
return city;
}
public void setCity(String value) {
this.city = value;
}
public String getState() {
return state;
}
public void setState(String value) {
this.state = value;
}
public String getPostalCode() {
return postalCode;
}
public void setPostalCode(String value) {
this.postalCode = value;
}
public String getCountry() {
return country;
}
public void setCountry(String value) {
this.country = value;
}
public String getPin() {
return pin;
}
public void setPin(String value) {
this.pin = value;
}
public FaceData getFace() {
return face;
}
public void setFace(FaceData value) {
this.face = value;
}
public VoiceData[] getVoiceTextDependent() {
return this.voiceTextDependent;
}
public void setVoiceTextDependent(VoiceData[] voiceSamples) {
this.voiceTextDependent = voiceSamples;
}
public VoiceData[] getVoiceTextPromptedDigits() {
return this.voiceTextPromptedDigits;
}
public void setVoiceTextPromptedDigits(VoiceData[] voiceDigits) {
this.voiceTextPromptedDigits = voiceDigits;
}
public Boolean getPinEnrolled() {
return pinEnrolled;
}
public void setPinEnrolled(Boolean value) {
this.pinEnrolled = value;
}
public Boolean getFaceEnrolled() {
return faceEnrolled;
}
public void setFaceEnrolled(Boolean value) {
this.faceEnrolled = value;
}
public Boolean getVoiceEnrolled() {
return voiceEnrolled;
}
public void setVoiceEnrolled(Boolean value) {
this.voiceEnrolled = value;
}
public Boolean getKeystrokesEnrolled() {
return keystrokesEnrolled;
}
public void setKeystrokesEnrolled(Boolean value) {
this.keystrokesEnrolled = value;
}
public Boolean getVoiceDigitsEnrolled() {
return voiceDigitsEnrolled;
}
public void setVoiceDigitsEnrolled(Boolean value) {
this.voiceDigitsEnrolled = value;
}
public Date getArchived() {
return archived;
}
public void setArchived(Date value) {
this.archived = value;
}
public Date getLockedUntil() {
return lockedUntil;
}
public void setLockedUntil(Date value) {
this.lockedUntil = value;
}
public Date getCreated() {
return created;
}
public void setCreated(Date createdDate) {
this.created = createdDate;
}
public Date getUpdated() {
return updated;
}
public void setUpdated(Date updated) {
this.updated = updated;
}
public String getLanguage() {
return language;
}
public void setLanguage(String value) {
this.language = value;
}
public String getSpeakerId() {
return speakerId;
}
public void setSpeakerId(String speakerId) {
this.speakerId = speakerId;
}
public UserStatusEnum getStatus() {
return status;
}
public void setStatus(UserStatusEnum status) {
this.status = status;
}
public SecretQuestion[] getSecretQuestion() {
return secretQuestion;
}
public void setSecretQuestion(SecretQuestion[] secretQuestion) {
this.secretQuestion = secretQuestion;
}
public Long getFailedVerificationCount() {
return failedVerificationCount;
}
public void setFailedVerificationCount(Long failedVerificationCount) {
this.failedVerificationCount = failedVerificationCount;
}
public Long getAccountUnlockedCount() {
return accountUnlockedCount;
}
public void setAccountUnlockedCount(Long accountUnlockedCount) {
this.accountUnlockedCount = accountUnlockedCount;
}
public Tenant getTenant() {
return tenant;
}
public void setTenant(Tenant tenant) {
this.tenant = tenant;
}
public RestCollection getApplications() {
return applications;
}
public void setApplications(RestCollection applications) {
this.applications = applications;
}
public User() {
}
public User(String href) {
super(href);
}
public RestCollection getRegistrations() {
return registrations;
}
public void setRegistrations(RestCollection registrations) {
this.registrations = registrations;
}
public RestCollection getAuthenticators() {
return authenticators;
}
public void setAuthenticators(RestCollection authenticators) {
this.authenticators = authenticators;
}
public RestCollection getAuthenticationRequests() {
return authenticationRequests;
}
public void setAuthenticationRequests(RestCollection authenticationRequests) {
this.authenticationRequests = authenticationRequests;
}
public RestCollection getSponsorships() {
return sponsorships;
}
public void setSponsorships(RestCollection sponsorships) {
this.sponsorships = sponsorships;
}
public RestCollection getRegistrationChallenges() {
return registrationChallenges;
}
public void setRegistrationChallenges(RestCollection registrationChallenges) {
this.registrationChallenges = registrationChallenges;
}
public Long getAdosPasscodeFailedAttempts() {
return adosPasscodeFailedAttempts;
}
public void setAdosPasscodeFailedAttempts(Long adosPasscodeFailedAttempts) {
this.adosPasscodeFailedAttempts = adosPasscodeFailedAttempts;
}
public Long getAdosPasscodeUnlockCount() {
return adosPasscodeUnlockCount;
}
public void setAdosPasscodeUnlockCount(Long adosPasscodeUnlockCount) {
this.adosPasscodeUnlockCount = adosPasscodeUnlockCount;
}
public Date getAdosPasscodeLockedUntilDtm() {
return adosPasscodeLockedUntilDtm;
}
public void setAdosPasscodeLockedUntilDtm(Date adosPasscodeLockedUntilDtm) {
this.adosPasscodeLockedUntilDtm = adosPasscodeLockedUntilDtm;
}
public Long getAdosFaceFailedAttempts() {
return adosFaceFailedAttempts;
}
public void setAdosFaceFailedAttempts(Long adosFaceFailedAttempts) {
this.adosFaceFailedAttempts = adosFaceFailedAttempts;
}
public Long getAdosFaceUnlockCount() {
return adosFaceUnlockCount;
}
public void setAdosFaceUnlockCount(Long adosFaceUnlockCount) {
this.adosFaceUnlockCount = adosFaceUnlockCount;
}
public Date getAdosFaceLockedUntilDtm() {
return adosFaceLockedUntilDtm;
}
public void setAdosFaceLockedUntilDtm(Date adosFaceLockedUntilDtm) {
this.adosFaceLockedUntilDtm = adosFaceLockedUntilDtm;
}
public Long getAdosVoiceFailedAttempts() {
return adosVoiceFailedAttempts;
}
public void setAdosVoiceFailedAttempts(Long adosVoiceFailedAttempts) {
this.adosVoiceFailedAttempts = adosVoiceFailedAttempts;
}
public Long getAdosVoiceUnlockCount() {
return adosVoiceUnlockCount;
}
public void setAdosVoiceUnlockCount(Long adosVoiceUnlockCount) {
this.adosVoiceUnlockCount = adosVoiceUnlockCount;
}
public Date getAdosVoiceLockedUntilDtm() {
return adosVoiceLockedUntilDtm;
}
public void setAdosVoiceLockedUntilDtm(Date adosVoiceLockedUntilDtm) {
this.adosVoiceLockedUntilDtm = adosVoiceLockedUntilDtm;
}
public Long getOtpFailedAttempts() {
return otpFailedAttempts;
}
public void setOtpFailedAttempts(Long otpFailedAttempts) {
this.otpFailedAttempts = otpFailedAttempts;
}
public Long getOtpUnlockCount() {
return otpUnlockCount;
}
public void setOtpUnlockCount(Long otpUnlockCount) {
this.otpUnlockCount = otpUnlockCount;
}
public Date getOtpLockedUntilDtm() {
return otpLockedUntilDtm;
}
public void setOtpLockedUntilDtm(Date otpLockedUntilDtm) {
this.otpLockedUntilDtm = otpLockedUntilDtm;
}
public Long getKeystrokesFailedAttempts() {
return keystrokesFailedAttempts;
}
public void setKeystrokesFailedAttempts(Long keystrokesFailedAttempts) {
this.keystrokesFailedAttempts = keystrokesFailedAttempts;
}
public Long getKeystrokesUnlockCount() {
return keystrokesUnlockCount;
}
public void setKeystrokesUnlockCount(Long keystrokesUnlockCount) {
this.keystrokesUnlockCount = keystrokesUnlockCount;
}
public Date getKeystrokesLockedUntilDtm() {
return keystrokesLockedUntilDtm;
}
public void setKeystrokesLockedUntilDtm(Date keystrokesLockedUntilDtm) {
this.keystrokesLockedUntilDtm = keystrokesLockedUntilDtm;
}
public RestCollection getEnrollments() {
return enrollments;
}
public void setEnrollments(RestCollection enrollments) {
this.enrollments = enrollments;
}
public RestCollection getSamples() {
return samples;
}
public void setSamples(RestCollection samples) {
this.samples = samples;
}
public Long getDirectOtpFailedAttempts() {
return directOtpFailedAttempts;
}
public void setDirectOtpFailedAttempts(Long directOtpFailedAttempts) {
this.directOtpFailedAttempts = directOtpFailedAttempts;
}
public Long getDirectOtpUnlockCount() {
return directOtpUnlockCount;
}
public void setDirectOtpUnlockCount(Long directOtpUnlockCount) {
this.directOtpUnlockCount = directOtpUnlockCount;
}
public Date getDirectOtpLockedUntilDtm() {
return directOtpLockedUntilDtm;
}
public void setDirectOtpLockedUntilDtm(Date directOtpLockedUntilDtm) {
this.directOtpLockedUntilDtm = directOtpLockedUntilDtm;
}
public Date getNextPossibleDirectOtpDtm() {
return nextPossibleDirectOtpDtm;
}
public void setNextPossibleDirectOtpDtm(Date nextPossibleDirectOtpDtm) {
this.nextPossibleDirectOtpDtm = nextPossibleDirectOtpDtm;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy