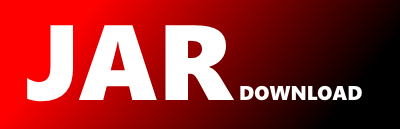
com.daon.identityx.rest.model.support.ActivityDefinitionBuilder Maven / Gradle / Ivy
/*
* Copyright Daon.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.daon.identityx.rest.model.support;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
public class ActivityDefinitionBuilder {
private static final String BASE_ACTIVITY_DEFINITION = "{\"enrollmentOptions\": [{\"_id\": \"none\"}],\"verificationOptions\": [%s]}";
private static final String FACE_OPTION_WITH_THRESHOLD = "\"face\": {\"face\": {\"match\": {\"threshold\": {\"type\": \"fmr\",\"value\": \"%s\"}}}}";
private static final String VOICE_OPTION_WITH_THRESHOLD = "\"voice\": {\"voice\": {\"match\": {\"threshold\": {\"type\": \"fmr\",\"value\": \"%s\"}}}}";
private static final String VOICE_OPTION_WITH_THRESHOLD_AND_MIN_SPEECH_DURATION = "\"voice\": {\"voice\": {\"minSpeechDuration\": %s, \"match\": {\"threshold\": {\"type\": \"fmr\",\"value\": \"%s\"}}}}";
private static final String FACE_OPTION = "\"face\": {\"face\": {}}";
private static final String VOICE_OPTION = "\"voice\": {\"voice\": {}}";
private static final String KEYSTROKES_OPTION = "\"keystrokes\": {\"keystrokes\": {}}";
private static final String KEYSTROKES_OPTION_WITH_THRESHOLD = "\"keystrokes\": {\"keystrokes\": {\"match\": {\"threshold\": {\"type\": \"fmr\",\"value\": \"%s\"}}}}";
private static final String VOICE_OPTION_WITH_MIN_SPEECH_DURATION = "\"voice\": {\"voice\": {\"minSpeechDuration\": %s}}";
private static final String OPTION = "{\"_id\": \"%s\", %s}";
private boolean orGroup;
private List options;
private ActivityDefinitionBuilder() {
super();
options = new ArrayList<>();
}
public String build() {
StringBuffer sb = new StringBuffer();
if (options.isEmpty()) {
sb.append(String.format(BASE_ACTIVITY_DEFINITION, "{\"_id\": \"none\"}"));
} else {
if (orGroup) {
StringBuffer groupsSb = new StringBuffer();
for (String option : options) {
String groupId = UUID.randomUUID().toString();
groupsSb.append(String.format(OPTION, groupId, option));
groupsSb.append(",");
}
groupsSb.deleteCharAt(groupsSb.length()-1);
sb.append(String.format(BASE_ACTIVITY_DEFINITION, groupsSb.toString()));
} else {
StringBuffer groupSb = new StringBuffer();
String groupId = UUID.randomUUID().toString();
for (String option : options) {
groupSb.append(option);
groupSb.append(",");
}
groupSb.deleteCharAt(groupSb.length()-1);
sb.append(String.format(BASE_ACTIVITY_DEFINITION, String.format(OPTION, groupId, groupSb.toString())));
}
}
return sb.toString();
}
public static ActivityDefinitionBuilder createORGroup() {
ActivityDefinitionBuilder b = new ActivityDefinitionBuilder();
b.orGroup = true;
return b;
}
public static ActivityDefinitionBuilder createANDGroup() {
ActivityDefinitionBuilder b = new ActivityDefinitionBuilder();
b.orGroup = false;
return b;
}
public ActivityDefinitionBuilder withFace() {
return withFace(null);
}
public ActivityDefinitionBuilder withKeystrokes() {
return withKeystrokes(null);
}
public ActivityDefinitionBuilder withVoice() {
return withVoice(null);
}
public ActivityDefinitionBuilder withVoice(Double threshold) {
if (threshold != null) {
addOption(VOICE_OPTION_WITH_THRESHOLD, threshold.toString());
} else {
addOption(VOICE_OPTION);
}
return this;
}
public ActivityDefinitionBuilder withVoice(Double threshold, Integer minSpeechDuration) {
if (threshold != null) {
if (minSpeechDuration != null) {
addOption(VOICE_OPTION_WITH_THRESHOLD_AND_MIN_SPEECH_DURATION, minSpeechDuration.toString(), threshold.toString());
} else {
addOption(VOICE_OPTION_WITH_THRESHOLD, threshold.toString());
}
} else {
if (minSpeechDuration != null) {
addOption(VOICE_OPTION_WITH_MIN_SPEECH_DURATION, minSpeechDuration.toString());
} else {
addOption(VOICE_OPTION);
}
}
return this;
}
public ActivityDefinitionBuilder withFace(Double threshold) {
if (threshold != null) {
addOption(FACE_OPTION_WITH_THRESHOLD, threshold.toString());
} else {
addOption(FACE_OPTION);
}
return this;
}
public ActivityDefinitionBuilder withKeystrokes(Double threshold) {
if (threshold != null) {
addOption(KEYSTROKES_OPTION_WITH_THRESHOLD, threshold.toString());
} else {
addOption(KEYSTROKES_OPTION);
}
return this;
}
protected void addOption(String format, Object...parameters) {
String option = String.format(format, parameters);
options.add(option);
}
protected void addOption(String format) {
options.add(format);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy