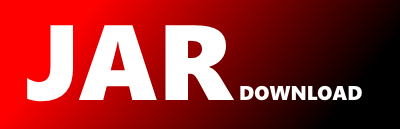
com.idilia.services.kb.QueryCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of idilia-java-sdk Show documentation
Show all versions of idilia-java-sdk Show documentation
Idilia Java SDK provides Java APIs for building software using Idilia linguistic services (Language graph, word sense disambiguation, paraphrasing, matching).
The newest version!
package com.idilia.services.kb;
import java.io.IOException;
import java.util.ArrayList;
import org.apache.http.Header;
import org.apache.http.HttpEntity;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonToken;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.idilia.services.base.IdiliaClientException;
class QueryCodec {
static QueryResponse decode(
ObjectMapper jsonMapper, Class tpRef,
HttpEntity rxEntity) throws IdiliaClientException, JsonParseException, UnsupportedOperationException, IOException {
Header ctHdr = rxEntity.getContentType();
if (ctHdr == null)
throw new IdiliaClientException("Unexpected no content type");
String ct = ctHdr.getValue();
if (!ct.startsWith("application/json"))
throw new IdiliaClientException("Unexpected content type: " + ct);
// Parse the JSON response where we cast the "result" member into the supplied Result
QueryResponse resp = new QueryResponse();
JsonParser jp = jsonMapper.getFactory().createParser(rxEntity.getContent());
jp.nextToken(); // skip object boundary
while (jp.nextToken() != JsonToken.END_OBJECT) {
String fieldName = jp.getCurrentName();
jp.nextToken();
if (fieldName.contentEquals("status"))
resp.setStatus(jp.getIntValue());
else if (fieldName.contentEquals("requestId"))
resp.setRequestId(jp.getText());
else if (fieldName.contentEquals("errorMsg"))
resp.setErrorMsg(jp.getText());
else if (fieldName.contentEquals("result"))
{
jp.nextToken(); // skip over array start
resp.setResult(new ArrayList<>());
while (jp.getCurrentToken() != JsonToken.END_ARRAY) {
T r = (T) jp.readValueAs(tpRef);
resp.addResult(r);
jp.nextToken(); // skip end of object
}
} else {
jp.skipChildren();
}
}
return resp;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy