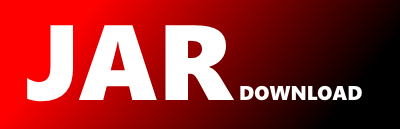
org.jpedal.examples.samples.javafx.DrawAdditionalObjects Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of OpenViewerFX Show documentation
Show all versions of OpenViewerFX Show documentation
Open Source (LGPL) JavaFX PDF Viewer
/*
* ===========================================
* Java Pdf Extraction Decoding Access Library
* ===========================================
*
* Project Info: http://www.idrsolutions.com
* Help section for developers at http://www.idrsolutions.com/support/
*
* (C) Copyright 1997-2017 IDRsolutions and Contributors.
*
* This file is part of JPedal/JPDF2HTML5
*
@LICENSE@
*
* ---------------
* DrawAdditionalObjects.java
* ---------------
*/
package org.jpedal.examples.samples.javafx;
import java.io.File;
import javafx.application.Application;
import javafx.application.Platform;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Text;
import javafx.scene.transform.Transform;
import javafx.stage.Stage;
import org.jpedal.PdfDecoderFX;
import org.jpedal.examples.viewer.Commands;
import org.jpedal.examples.viewer.ViewerFX;
import org.jpedal.exception.PdfException;
public class DrawAdditionalObjects extends Application {
ViewerFX myViewer;
public static void main(final String[] args) {
launch(args);
}
@Override
public void start(final Stage stage) throws Exception {
// Create and initialise JPedal viewer component
myViewer = new ViewerFX(stage, null);
myViewer.setupViewer();
myViewer.executeCommand(Commands.OPENFILE, new Object[]{new File("/PDFdata/test_data/Hand_Test/awjune2003.pdf")});
drawOverFXDisplay();
/*
* Start never exits so I need to remove on thread to demo feature
*/
final Thread thread = new Thread() {
@Override
public void run() {
try {
Thread.sleep(5000);
} catch (final InterruptedException ex) {
ex.printStackTrace();
}
Platform.runLater(new Runnable() {
@Override
public void run() {
System.out.println("Clear page 5 seconds later");
clearFXDisplay();
}
});
}
};
thread.start();
}
public void clearFXDisplay() {
final PdfDecoderFX decode_pdf = (PdfDecoderFX) myViewer.getPdfDecoder();
// Pass into JPEDAL after page decoded - will be removed automatically on new page/open file
try {
decode_pdf.flushAdditionalObjectsOnPage(1);
} catch (final PdfException e) {
e.printStackTrace();
}
}
public void drawOverFXDisplay() {
final PdfDecoderFX decode_pdf = (PdfDecoderFX) myViewer.getPdfDecoder();
/*
* In this example, we create a rectangle, a filled rectangle and draw some text.
* Initialise objects arrays - we will put 3 shapes on the page
* (using PDF co-ordinates with origin bottom left corner)
*/
final int count = 3;
/*
* Due to the way some pdf's are created it is necessery to take the offset of a page
* into account when addding custom objects to the page. Variables mX and mY represent
* that offset and need to be taken in to account when placing any additional object
* on a page.
*/
final int mX = decode_pdf.getPdfPageData().getMediaBoxX(1);
final int mY = decode_pdf.getPdfPageData().getMediaBoxY(1);
final int[] type = new int[count];
final Object[] obj = new Object[count];
//
/*
* JavaFX implementation
*
* JavaFX is much more flexible than Swing so we just implement the custom method and allow user to create Shape in FX
*
* Critical part of the code is to invert the Text with
* t.getTransforms().setAll(Transform.affine(1,0,0,-1,0,0));
* This is because PDF page is upside down compared to JavaFX.
*/
final Rectangle strokedRect = new Rectangle(0, 0, 510, 50); //ALSO sets location. Any shape can be used
strokedRect.setTranslateX(35 + mX);
strokedRect.setTranslateY(35 + mY);
strokedRect.setStroke(javafx.scene.paint.Color.RED);
strokedRect.setFill(null);
type[0] = org.jpedal.render.DynamicVectorRenderer.CUSTOM;
obj[0] = strokedRect;
final Rectangle filledRect = new Rectangle(0, 0, 500, 40); //ALSO sets location. Any shape can be used
filledRect.setTranslateX(40 + mX);
filledRect.setTranslateY(40 + mY);
filledRect.setStroke(null);
filledRect.setFill(javafx.scene.paint.Color.GREEN);
type[1] = org.jpedal.render.DynamicVectorRenderer.CUSTOM;
obj[1] = filledRect;
final Text t = new Text("Example text on page ");
t.getTransforms().setAll(Transform.affine(1, 0, 0, -1, 0, 0)); //critical as first step fo text
t.setFill(javafx.scene.paint.Color.BLUE);
t.setFont(new javafx.scene.text.Font("Tahoma", 48));
t.setTranslateX(40 + mX);
t.setTranslateY(40 + mY);
type[2] = org.jpedal.render.DynamicVectorRenderer.CUSTOM;
obj[2] = t;
//pass into JPEDAL after page decoded - will be removed automatically on new page/open file
try {
decode_pdf.drawAdditionalObjectsOverPage(1, type, null, obj);
} catch (final PdfException e) {
e.printStackTrace();
}
//recode the page to flush your objects
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy