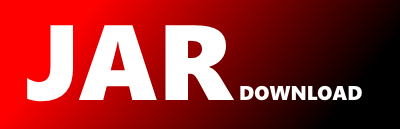
org.jpedal.parser.DecoderResults Maven / Gradle / Ivy
Show all versions of OpenViewerFX Show documentation
/*
* ===========================================
* Java Pdf Extraction Decoding Access Library
* ===========================================
*
* Project Info: http://www.idrsolutions.com
* Help section for developers at http://www.idrsolutions.com/support/
*
* (C) Copyright 1997-2017 IDRsolutions and Contributors.
*
* This file is part of JPedal/JPDF2HTML5
*
@LICENSE@
*
* ---------------
* DecoderResults.java
* ---------------
*/
package org.jpedal.parser;
import java.util.Iterator;
import org.jpedal.constants.PageInfo;
public class DecoderResults {
private boolean imagesProcessedFully = true, hasNonEmbeddedCIDFonts, hasYCCKimages, pageSuccessful;
private boolean ttHintingRequired;
//values on last decodePage
private Iterator colorSpacesUsed;
private String nonEmbeddedCIDFonts = "";
private boolean tooManyShapes;
/**
* flag to show embedded fonts present
*/
private boolean hasEmbeddedFonts;
public boolean getImagesProcessedFully() {
return imagesProcessedFully;
}
public void update(final PdfStreamDecoder current, final boolean includeAll) {
colorSpacesUsed = (Iterator) current.getObjectValue(PageInfo.COLORSPACES);
nonEmbeddedCIDFonts = (String) current.getObjectValue(DecodeStatus.NonEmbeddedCIDFonts);
hasYCCKimages = current.getBooleanValue(DecodeStatus.YCCKImages);
pageSuccessful = current.getBooleanValue(DecodeStatus.PageDecodingSuccessful);
imagesProcessedFully = current.getBooleanValue(DecodeStatus.ImagesProcessed);
tooManyShapes = current.getBooleanValue(DecodeStatus.TooManyShapes);
hasNonEmbeddedCIDFonts = current.getBooleanValue(DecodeStatus.NonEmbeddedCIDFonts);
ttHintingRequired = current.getBooleanValue(DecodeStatus.TTHintingRequired);
if (includeAll) {
hasEmbeddedFonts = current.getBooleanValue(ValueTypes.EmbeddedFonts);
}
}
public boolean getPageDecodeStatus(final int status) {
switch (status) {
case DecodeStatus.NonEmbeddedCIDFonts:
return hasNonEmbeddedCIDFonts;
case DecodeStatus.ImagesProcessed:
return imagesProcessedFully;
case DecodeStatus.PageDecodingSuccessful:
return pageSuccessful;
case DecodeStatus.YCCKImages:
return hasYCCKimages;
case DecodeStatus.TooManyShapes:
return tooManyShapes;
case DecodeStatus.TTHintingRequired:
return ttHintingRequired;
default:
throw new RuntimeException("Unknown parameter " + status);
}
}
/**
* return details on page for type (defined in org.jpedal.constants.PageInfo) or null if no values
* Unrecognised key will throw a RunTime exception
*
* null returned if JPedal not clear on result
*/
public Iterator getPageInfo(final int type) {
switch (type) {
case PageInfo.COLORSPACES:
return colorSpacesUsed;
default:
return null;
}
}
/**
* get page statuses
*/
public String getPageDecodeStatusReport(final int status) {
if (status == DecodeStatus.NonEmbeddedCIDFonts) {
return nonEmbeddedCIDFonts;
} else {
throw new RuntimeException("Unknown parameter");
}
}
/**
* shows if embedded fonts present on page just decoded
*/
public boolean hasEmbeddedFonts() {
return hasEmbeddedFonts;
}
public void resetColorSpaces() {
//disable color list if forms
colorSpacesUsed = null;
}
}