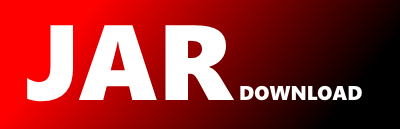
org.jpedal.function.PDFExponential Maven / Gradle / Ivy
/*
* ===========================================
* Java Pdf Extraction Decoding Access Library
* ===========================================
*
* Project Info: http://www.idrsolutions.com
* Help section for developers at http://www.idrsolutions.com/support/
*
* (C) Copyright 1997-2017 IDRsolutions and Contributors.
*
* This file is part of JPedal/JPDF2HTML5
*
@LICENSE@
*
* ---------------
* PDFExponential.java
* ---------------
*/
package org.jpedal.function;
/**
* Class to handle Type 2 shading (Exponential)
*/
public class PDFExponential extends PDFGenericFunction implements PDFFunction {
private float[] C0 = {0.0f}, C1 = {1.0f};
private final float N;
final int returnValues;
private final float[] diffs;
public PDFExponential(final float N, final float[] C0, final float[] C1, final float[] domain, final float[] range) {
super(domain, range);
this.N = N;
if (C0 != null) {
this.C0 = C0;
}
if (C1 != null) {
this.C1 = C1;
}
//note C0 might be null so use this.C0
returnValues = this.C0.length;
diffs = new float[returnValues];
for (int i = 0; i < diffs.length; i++) {
diffs[i] = this.C1[i] - this.C0[i];
}
}
/**
* Compute the required values for exponential shading (Only used by
* Stitching)
*
* @param subinput : input values
* @return The shading values as float[]
*/
@Override
public float[] computeStitch(final float[] subinput) {
return compute(subinput);
}
@Override
public float[] compute(final float[] values) {
final float[] output = new float[returnValues];
float x = min(max(values[0], domain[0]), domain[1]);
if (N != 1.0) {
x = (float) Math.pow(x, N);
}
for (int j = 0; j < C0.length; j++) {
output[j] = C0[j] + x * diffs[j];
}
if (range != null) {
for (int i = 0; i < C0.length; i++) {
output[i] = (min(max(output[i], range[i * 2]), range[i * 2 + 1]));
}
}
return output;
}
}
// //Only first value required
// final float x=min(max(values[0],domain[0*2]),domain[0*2+1]);
//
// if (N==1f){ // special case
//
// for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy