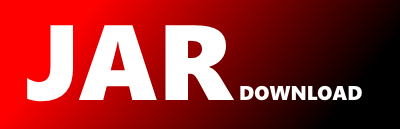
org.jpedal.io.RandomAccessFCTest Maven / Gradle / Ivy
/*
* ===========================================
* Java Pdf Extraction Decoding Access Library
* ===========================================
*
* Project Info: http://www.idrsolutions.com
* Help section for developers at http://www.idrsolutions.com/support/
*
* (C) Copyright 1997-2017 IDRsolutions and Contributors.
*
* This file is part of JPedal/JPDF2HTML5
*
@LICENSE@
*
* ---------------
* RandomAccessFCTest.java
* ---------------
*/
package org.jpedal.io;
import java.io.FileInputStream;
import java.io.IOException;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
import org.jpedal.utils.LogWriter;
public class RandomAccessFCTest implements RandomAccessBuffer {
//private byte[] data;
private long pointer;
private int length;
private FileChannel fc;
private MappedByteBuffer mb;
public RandomAccessFCTest(final FileInputStream inFile) {
try {
length = inFile.available();
fc = (inFile).getChannel();
mb = fc.map(FileChannel.MapMode.READ_ONLY, 0L, fc.size());
} catch (final Exception e) {
LogWriter.writeLog("Exception: " + e.getMessage());
}
}
@Override
public long getFilePointer() throws IOException {
return pointer;
}
@Override
public void seek(final long pos) throws IOException {
if (checkPos(pos)) {
this.pointer = pos;
} else {
throw new IOException("Position out of bounds");
}
}
@Override
public void close() throws IOException {
if (fc != null) {
fc.close();
fc = null;
}
if (mb != null) {
mb = null;
}
this.pointer = -1;
}
/**/
@Override
protected void finalize() {
try {
super.finalize();
} catch (final Throwable e) {
LogWriter.writeLog("Exception: " + e.getMessage());
}
//ensure removal actual file
try {
close();
} catch (final IOException e) {
LogWriter.writeLog("Exception: " + e.getMessage());
}
}
@Override
public long length() throws IOException {
if (mb != null) {
return length;
} else {
throw new IOException("Data buffer not initialized.");
}
}
@Override
public int read() throws IOException {
if (checkPos(this.pointer)) {
mb.position((int) pointer);
pointer++;
return mb.get();
} else {
return -1;
}
}
private int peek() throws IOException {
if (checkPos(this.pointer)) {
mb.position((int) pointer);
return mb.get();
} else {
return -1;
}
}
/**
* return next line (returns null if no line)
*/
@Override
public String readLine() throws IOException {
if (this.pointer >= this.length - 1) {
return null;
} else {
final StringBuilder buf = new StringBuilder();
int c;
while ((c = read()) >= 0) {
if ((c == 10) || (c == 13)) {
if (((peek() == 10) || (peek() == 13)) && (peek() != c)) {
read();
}
break;
}
buf.append((char) c);
}
return buf.toString();
}
}
@Override
public int read(final byte[] b) throws IOException {
if (mb == null) {
throw new IOException("Data buffer not initialized.");
}
if (pointer < 0 || pointer >= length) {
return -1;
}
int length = this.length - (int) pointer;
if (length > b.length) {
length = b.length;
}
for (int i = 0; i < length; i++) {
mb.position((int) pointer);
pointer++;
b[i] = mb.get();
}
return length;
}
private boolean checkPos(final long pos) throws IOException {
return ((pos >= 0) && (pos < length()));
}
/* returns the byte data*/
@Override
public byte[] getPdfBuffer() {
final byte[] bytes = new byte[length];
mb.position(0);
mb.get(bytes);
return bytes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy