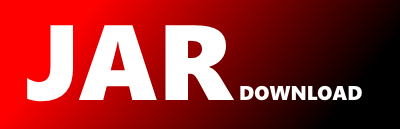
com.ig.orchestrations.chart.data.ChartDataRequestReject Maven / Gradle / Ivy
package com.ig.orchestrations.chart.data;
import java.util.HashMap;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import com.ig.orchestrations.us.rfed.fields.SecurityIDSource;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"MsgType",
"ApplVerID",
"SendingTime",
"CstmApplVerID",
"ReqID",
"Symbol",
"SecurityID",
"SecurityIDSource",
"ChartRequestRejectReason",
"Text"
})
public class ChartDataRequestReject {
@JsonProperty("MsgType")
private String msgType;
@JsonProperty("ApplVerID")
private ChartDataRequestReject.ApplVerID applVerID;
@JsonProperty("SendingTime")
private String sendingTime;
@JsonProperty("CstmApplVerID")
private String cstmApplVerID;
@JsonProperty("ReqID")
private String reqID;
@JsonProperty("Symbol")
private String symbol;
/**
*
* (Required)
*
*/
@JsonProperty("SecurityID")
private String securityID;
/**
* SecurityIDSource
*
* JSON Schema for field SecurityIDSource
*
*/
@JsonProperty("SecurityIDSource")
@JsonPropertyDescription("JSON Schema for field SecurityIDSource")
private SecurityIDSource securityIDSource;
@JsonProperty("ChartRequestRejectReason")
private Object chartRequestRejectReason;
@JsonProperty("Text")
private String text;
@JsonIgnore
private Map additionalProperties = new HashMap();
@JsonProperty("MsgType")
public String getMsgType() {
return msgType;
}
@JsonProperty("MsgType")
public void setMsgType(String msgType) {
this.msgType = msgType;
}
@JsonProperty("ApplVerID")
public ChartDataRequestReject.ApplVerID getApplVerID() {
return applVerID;
}
@JsonProperty("ApplVerID")
public void setApplVerID(ChartDataRequestReject.ApplVerID applVerID) {
this.applVerID = applVerID;
}
@JsonProperty("SendingTime")
public String getSendingTime() {
return sendingTime;
}
@JsonProperty("SendingTime")
public void setSendingTime(String sendingTime) {
this.sendingTime = sendingTime;
}
@JsonProperty("CstmApplVerID")
public String getCstmApplVerID() {
return cstmApplVerID;
}
@JsonProperty("CstmApplVerID")
public void setCstmApplVerID(String cstmApplVerID) {
this.cstmApplVerID = cstmApplVerID;
}
@JsonProperty("ReqID")
public String getReqID() {
return reqID;
}
@JsonProperty("ReqID")
public void setReqID(String reqID) {
this.reqID = reqID;
}
@JsonProperty("Symbol")
public String getSymbol() {
return symbol;
}
@JsonProperty("Symbol")
public void setSymbol(String symbol) {
this.symbol = symbol;
}
/**
*
* (Required)
*
*/
@JsonProperty("SecurityID")
public String getSecurityID() {
return securityID;
}
/**
*
* (Required)
*
*/
@JsonProperty("SecurityID")
public void setSecurityID(String securityID) {
this.securityID = securityID;
}
/**
* SecurityIDSource
*
* JSON Schema for field SecurityIDSource
*
*/
@JsonProperty("SecurityIDSource")
public SecurityIDSource getSecurityIDSource() {
return securityIDSource;
}
/**
* SecurityIDSource
*
* JSON Schema for field SecurityIDSource
*
*/
@JsonProperty("SecurityIDSource")
public void setSecurityIDSource(SecurityIDSource securityIDSource) {
this.securityIDSource = securityIDSource;
}
@JsonProperty("ChartRequestRejectReason")
public Object getChartRequestRejectReason() {
return chartRequestRejectReason;
}
@JsonProperty("ChartRequestRejectReason")
public void setChartRequestRejectReason(Object chartRequestRejectReason) {
this.chartRequestRejectReason = chartRequestRejectReason;
}
@JsonProperty("Text")
public String getText() {
return text;
}
@JsonProperty("Text")
public void setText(String text) {
this.text = text;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(ChartDataRequestReject.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("msgType");
sb.append('=');
sb.append(((this.msgType == null)?"":this.msgType));
sb.append(',');
sb.append("applVerID");
sb.append('=');
sb.append(((this.applVerID == null)?"":this.applVerID));
sb.append(',');
sb.append("sendingTime");
sb.append('=');
sb.append(((this.sendingTime == null)?"":this.sendingTime));
sb.append(',');
sb.append("cstmApplVerID");
sb.append('=');
sb.append(((this.cstmApplVerID == null)?"":this.cstmApplVerID));
sb.append(',');
sb.append("reqID");
sb.append('=');
sb.append(((this.reqID == null)?"":this.reqID));
sb.append(',');
sb.append("symbol");
sb.append('=');
sb.append(((this.symbol == null)?"":this.symbol));
sb.append(',');
sb.append("securityID");
sb.append('=');
sb.append(((this.securityID == null)?"":this.securityID));
sb.append(',');
sb.append("securityIDSource");
sb.append('=');
sb.append(((this.securityIDSource == null)?"":this.securityIDSource));
sb.append(',');
sb.append("chartRequestRejectReason");
sb.append('=');
sb.append(((this.chartRequestRejectReason == null)?"":this.chartRequestRejectReason));
sb.append(',');
sb.append("text");
sb.append('=');
sb.append(((this.text == null)?"":this.text));
sb.append(',');
sb.append("additionalProperties");
sb.append('=');
sb.append(((this.additionalProperties == null)?"":this.additionalProperties));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.applVerID == null)? 0 :this.applVerID.hashCode()));
result = ((result* 31)+((this.symbol == null)? 0 :this.symbol.hashCode()));
result = ((result* 31)+((this.msgType == null)? 0 :this.msgType.hashCode()));
result = ((result* 31)+((this.chartRequestRejectReason == null)? 0 :this.chartRequestRejectReason.hashCode()));
result = ((result* 31)+((this.cstmApplVerID == null)? 0 :this.cstmApplVerID.hashCode()));
result = ((result* 31)+((this.securityID == null)? 0 :this.securityID.hashCode()));
result = ((result* 31)+((this.text == null)? 0 :this.text.hashCode()));
result = ((result* 31)+((this.additionalProperties == null)? 0 :this.additionalProperties.hashCode()));
result = ((result* 31)+((this.securityIDSource == null)? 0 :this.securityIDSource.hashCode()));
result = ((result* 31)+((this.sendingTime == null)? 0 :this.sendingTime.hashCode()));
result = ((result* 31)+((this.reqID == null)? 0 :this.reqID.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof ChartDataRequestReject) == false) {
return false;
}
ChartDataRequestReject rhs = ((ChartDataRequestReject) other);
return ((((((((((((this.applVerID == rhs.applVerID)||((this.applVerID!= null)&&this.applVerID.equals(rhs.applVerID)))&&((this.symbol == rhs.symbol)||((this.symbol!= null)&&this.symbol.equals(rhs.symbol))))&&((this.msgType == rhs.msgType)||((this.msgType!= null)&&this.msgType.equals(rhs.msgType))))&&((this.chartRequestRejectReason == rhs.chartRequestRejectReason)||((this.chartRequestRejectReason!= null)&&this.chartRequestRejectReason.equals(rhs.chartRequestRejectReason))))&&((this.cstmApplVerID == rhs.cstmApplVerID)||((this.cstmApplVerID!= null)&&this.cstmApplVerID.equals(rhs.cstmApplVerID))))&&((this.securityID == rhs.securityID)||((this.securityID!= null)&&this.securityID.equals(rhs.securityID))))&&((this.text == rhs.text)||((this.text!= null)&&this.text.equals(rhs.text))))&&((this.additionalProperties == rhs.additionalProperties)||((this.additionalProperties!= null)&&this.additionalProperties.equals(rhs.additionalProperties))))&&((this.securityIDSource == rhs.securityIDSource)||((this.securityIDSource!= null)&&this.securityIDSource.equals(rhs.securityIDSource))))&&((this.sendingTime == rhs.sendingTime)||((this.sendingTime!= null)&&this.sendingTime.equals(rhs.sendingTime))))&&((this.reqID == rhs.reqID)||((this.reqID!= null)&&this.reqID.equals(rhs.reqID))));
}
public enum ApplVerID {
FIX_27("FIX27"),
FIX_30("FIX30"),
FIX_40("FIX40"),
FIX_41("FIX41"),
FIX_42("FIX42"),
FIX_43("FIX43"),
FIX_44("FIX44"),
FIX_50("FIX50"),
FIX_50_SP_1("FIX50SP1"),
FIX_50_SP_2("FIX50SP2");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (ChartDataRequestReject.ApplVerID c: values()) {
CONSTANTS.put(c.value, c);
}
}
private ApplVerID(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static ChartDataRequestReject.ApplVerID fromValue(String value) {
ChartDataRequestReject.ApplVerID constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}