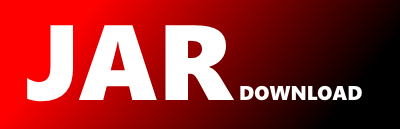
com.ig.orchestrations.chart.data.HistoricCandleResponse Maven / Gradle / Ivy
package com.ig.orchestrations.chart.data;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"MsgType",
"ApplVerID",
"CstmApplVerID",
"ReqID",
"Interval",
"SendingTime",
"CandleData"
})
public class HistoricCandleResponse {
@JsonProperty("MsgType")
private String msgType;
@JsonProperty("ApplVerID")
private HistoricCandleResponse.ApplVerID applVerID;
@JsonProperty("CstmApplVerID")
private String cstmApplVerID;
@JsonProperty("ReqID")
private String reqID;
@JsonProperty("Interval")
private Interval interval;
@JsonProperty("SendingTime")
private String sendingTime;
@JsonProperty("CandleData")
private List candleData = new ArrayList();
@JsonIgnore
private Map additionalProperties = new HashMap();
@JsonProperty("MsgType")
public String getMsgType() {
return msgType;
}
@JsonProperty("MsgType")
public void setMsgType(String msgType) {
this.msgType = msgType;
}
@JsonProperty("ApplVerID")
public HistoricCandleResponse.ApplVerID getApplVerID() {
return applVerID;
}
@JsonProperty("ApplVerID")
public void setApplVerID(HistoricCandleResponse.ApplVerID applVerID) {
this.applVerID = applVerID;
}
@JsonProperty("CstmApplVerID")
public String getCstmApplVerID() {
return cstmApplVerID;
}
@JsonProperty("CstmApplVerID")
public void setCstmApplVerID(String cstmApplVerID) {
this.cstmApplVerID = cstmApplVerID;
}
@JsonProperty("ReqID")
public String getReqID() {
return reqID;
}
@JsonProperty("ReqID")
public void setReqID(String reqID) {
this.reqID = reqID;
}
@JsonProperty("Interval")
public Interval getInterval() {
return interval;
}
@JsonProperty("Interval")
public void setInterval(Interval interval) {
this.interval = interval;
}
@JsonProperty("SendingTime")
public String getSendingTime() {
return sendingTime;
}
@JsonProperty("SendingTime")
public void setSendingTime(String sendingTime) {
this.sendingTime = sendingTime;
}
@JsonProperty("CandleData")
public List getCandleData() {
return candleData;
}
@JsonProperty("CandleData")
public void setCandleData(List candleData) {
this.candleData = candleData;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(HistoricCandleResponse.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("msgType");
sb.append('=');
sb.append(((this.msgType == null)?"":this.msgType));
sb.append(',');
sb.append("applVerID");
sb.append('=');
sb.append(((this.applVerID == null)?"":this.applVerID));
sb.append(',');
sb.append("cstmApplVerID");
sb.append('=');
sb.append(((this.cstmApplVerID == null)?"":this.cstmApplVerID));
sb.append(',');
sb.append("reqID");
sb.append('=');
sb.append(((this.reqID == null)?"":this.reqID));
sb.append(',');
sb.append("interval");
sb.append('=');
sb.append(((this.interval == null)?"":this.interval));
sb.append(',');
sb.append("sendingTime");
sb.append('=');
sb.append(((this.sendingTime == null)?"":this.sendingTime));
sb.append(',');
sb.append("candleData");
sb.append('=');
sb.append(((this.candleData == null)?"":this.candleData));
sb.append(',');
sb.append("additionalProperties");
sb.append('=');
sb.append(((this.additionalProperties == null)?"":this.additionalProperties));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.applVerID == null)? 0 :this.applVerID.hashCode()));
result = ((result* 31)+((this.msgType == null)? 0 :this.msgType.hashCode()));
result = ((result* 31)+((this.candleData == null)? 0 :this.candleData.hashCode()));
result = ((result* 31)+((this.cstmApplVerID == null)? 0 :this.cstmApplVerID.hashCode()));
result = ((result* 31)+((this.interval == null)? 0 :this.interval.hashCode()));
result = ((result* 31)+((this.additionalProperties == null)? 0 :this.additionalProperties.hashCode()));
result = ((result* 31)+((this.sendingTime == null)? 0 :this.sendingTime.hashCode()));
result = ((result* 31)+((this.reqID == null)? 0 :this.reqID.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof HistoricCandleResponse) == false) {
return false;
}
HistoricCandleResponse rhs = ((HistoricCandleResponse) other);
return (((((((((this.applVerID == rhs.applVerID)||((this.applVerID!= null)&&this.applVerID.equals(rhs.applVerID)))&&((this.msgType == rhs.msgType)||((this.msgType!= null)&&this.msgType.equals(rhs.msgType))))&&((this.candleData == rhs.candleData)||((this.candleData!= null)&&this.candleData.equals(rhs.candleData))))&&((this.cstmApplVerID == rhs.cstmApplVerID)||((this.cstmApplVerID!= null)&&this.cstmApplVerID.equals(rhs.cstmApplVerID))))&&((this.interval == rhs.interval)||((this.interval!= null)&&this.interval.equals(rhs.interval))))&&((this.additionalProperties == rhs.additionalProperties)||((this.additionalProperties!= null)&&this.additionalProperties.equals(rhs.additionalProperties))))&&((this.sendingTime == rhs.sendingTime)||((this.sendingTime!= null)&&this.sendingTime.equals(rhs.sendingTime))))&&((this.reqID == rhs.reqID)||((this.reqID!= null)&&this.reqID.equals(rhs.reqID))));
}
public enum ApplVerID {
FIX_27("FIX27"),
FIX_30("FIX30"),
FIX_40("FIX40"),
FIX_41("FIX41"),
FIX_42("FIX42"),
FIX_43("FIX43"),
FIX_44("FIX44"),
FIX_50("FIX50"),
FIX_50_SP_1("FIX50SP1"),
FIX_50_SP_2("FIX50SP2");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (HistoricCandleResponse.ApplVerID c: values()) {
CONSTANTS.put(c.value, c);
}
}
private ApplVerID(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static HistoricCandleResponse.ApplVerID fromValue(String value) {
HistoricCandleResponse.ApplVerID constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy