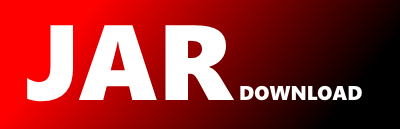
com.igormaznitsa.meta.Complexity Maven / Gradle / Ivy
/*
* Copyright 2002-2019 Igor Maznitsa (http://www.igormaznitsa.com)
*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.igormaznitsa.meta;
import javax.annotation.Nonnull;
/**
* Complexity constants. List was taken from the wiki page.
*
* @since 1.1.2
*/
public enum Complexity {
/**
* Constant value. Example: Determining if an integer (represented in binary) is even or odd.
*
* O(1)
*
* @since 1.1.2
*/
CONSTANT("O(1)"),
/**
* Inverse Ackermann. Example: Amortized time per operation using a disjoint set.
*
* O(α(n))
*
* @since 1.1.2
*/
INVERSE_ACKERMANN("O(a(n))"),
/**
* Iterated logarithmic. Example:
* Distributed coloring of cycles.
*
* O(log* n)
*
* @since 1.1.2
*/
ITERATED_LOGARITHMIC("O(log* n)"),
/**
* Log-logarithmic. Example: Amortized time per operation using a bounded priority queue.
*
* O(log log n)
*
* @since 1.1.2
*/
LOG_LOGARITHMIC("O(log log n)"),
/**
* Logarithmic. Example: Binary search.
*
* O(log n)
*
* @since 1.1.2
*/
LOGARITHMIC("O(log n)"),
/**
* Polylogarithmic.
*
* poly(log n)
*
* @since 1.1.2
*/
POLYLOGARITHMIC("poly(log n)"),
/**
* Fractional power. Example: Searching in a kd-tree.
*
* O(nc) where 0 < c < 1
*
* @since 1.1.2
*/
FRACTIONAL_POWER("O(n^c)"),
/**
* Linear. Example: Finding the smallest or largest item in an unsorted array.
*
* O(n)
*
* @since 1.1.2
*/
LINEAR("O(n)"),
/**
* n log star n. Example: Seidel's polygon triangulation algorithm.
*
* O(n log* n)
*
* @since 1.1.2
*/
N_LOG_STAR_N("O(n log* n)"),
/**
* Lineqarithmic. Example: Fastest possible comparison sort.
*
* O(n log n)
*
* @since 1.1.2
*/
LINEARITHMIC("O(n log n)"),
/**
* Quadratic. Example: Bubble sort; Insertion sort; Direct convolution.
*
* O(n2)
*
* @since 1.1.2
*/
QUADRATIC("O(n^2)"),
/**
* Cubic. Example: Naive multiplication of two n×n matrices. Calculating partial correlation.
*
* O(n3)
*
* @since 1.1.2
*/
CUBIC("O(n^3)"),
/**
* Polynomial. Example: Karmarkar's
* algorithm for linear programming; AKS primality test.
*
* 2O(log n) = poly(n)
*
* @since 1.1.2
*/
POLYNOMIAL("poly(n)"),
/**
* Quasi-polinomial. Example: Best-known O(log2 n)-approximation algorithm for the directed
* Steiner tree problem.
*
* 2poly(log n)
*
* @since 1.1.2
*/
QUASI_POLYNOMIAL("2^poly(log n)"),
/**
* Sub-exponential. Example: Assuming complexity theoretic conjectures, BPP is contained in
* SUBEXP.
*
* O(2nε) for all ε > 0
*
* @since 1.1.2
*/
SUB_EXPONENTIAL("O(2^n^e)"),
/**
* Exponential. Example: Solving matrix chain multiplication via brute-force search.
*
* 2O(n)
*
* @since 1.1.2
*/
EXPONENTIAL("2^O(n)"),
/**
* Factorial. Example: Solving the traveling salesman problem via brute-force search.
*
* O(n!)
*
* @since 1.1.2
*/
FACTORIAL("O(n!)"),
/**
* Double exponential. Example: Deciding the truth of a given statement in Presburger
* arithmetic.
*
* 22poly(n)
*
* @since 1.1.2
*/
DOUBLE_EXPONENTIAL("2^2^poly(n)");
private final String formula;
Complexity(@Nonnull final String formula) {
this.formula = formula;
}
/**
* Get the formula.
*
* @return formula as string
* @since 1.1.2
*/
@Nonnull
public String getFormula() {
return this.formula;
}
@Override
@Nonnull
public String toString() {
return this.formula;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy