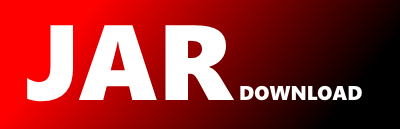
com.igumnov.common.File Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of common Show documentation
Show all versions of common Show documentation
Common Java library - Simple, small, stable and fast library with Simplified Enterprise Server (Dependency Injection, WebServer and ORM Frameworks)
package com.igumnov.common;
import java.io.*;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.stream.Stream;
public class File {
public static String readAllToString(String fileName) throws IOException {
java.io.File file = new java.io.File(fileName);
FileInputStream fis = new FileInputStream(file);
byte[] data = new byte[(int) file.length()];
fis.read(data);
fis.close();
return new String(data, "UTF-8");
}
public static void writeString(String str, String fileName) throws IOException {
try ( Writer out = new BufferedWriter( new OutputStreamWriter(
new FileOutputStream( fileName ), "UTF-8" ) ) ) {
out.write( str );
}
}
public static void appendLine(String line, String filename) throws IOException {
try(PrintWriter output = new PrintWriter(new FileWriter(filename,true)))
{
output.printf("%s\r\n", line);
}
}
public static Stream readLines(String fileName) throws IOException {
return Files.lines(Paths.get(fileName));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy