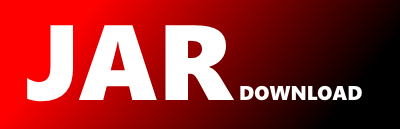
com.iih5.smartorm.dialect.MysqlDialect Maven / Gradle / Ivy
/**
* Copyright (c) 2011-2016, James Zhan 詹波 ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.iih5.smartorm.dialect;
import com.iih5.smartorm.kit.StringKit;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class MysqlDialect implements Dialect {
public String forModelFindBy(String tableName,String columns,String conditions) {
StringBuilder sql = new StringBuilder("select ");
columns = columns.trim();
if ("*".equals(columns)) {
sql.append("*");
}
else {
String[] arr = columns.split(",");
for (int i=0; i 0) {
sql.append(",");
}
sql.append(" ").append(arr[i].trim()).append(" ");
}
}
sql.append(" from ");
sql.append(tableName);
if (conditions==null || conditions.equals("")){
return sql.toString();
}
sql.append(" where 1=1 ");
sql.append(conditions);
return sql.toString();
}
public String deleteByCondition(String tableName,String conditions) {
StringBuilder sql = new StringBuilder(45);
sql.append("delete from ");
sql.append(tableName);
sql.append(" where 1=1 ");
sql.append(conditions);
return sql.toString();
}
public void forModelSave(String tableName, Map attrs, StringBuilder sql, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy