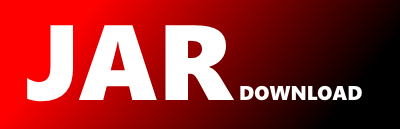
com.iih5.smartorm.generator.TableMetaUtil Maven / Gradle / Ivy
package com.iih5.smartorm.generator;
import com.alibaba.fastjson.JSON;
import com.iih5.smartorm.model.Db;
import java.sql.*;
import java.util.*;
public class TableMetaUtil {
/**
* 从db获取库表和字段信息
* @param dataSource
* @return
* @throws Exception
*/
public static List findTableMetaList(String dataSource) throws Exception{
List tableList = new ArrayList();
List sets = null;
if (dataSource !=null){
sets = Db.use(dataSource).findList("show tables ;",new Object[]{} ,String.class);
}else {
sets = Db.findList("show tables ;",new Object[]{} ,String.class);
}
for (String name:sets) {
String sql = " show full columns from "+name+" ;";
TableMeta meta = new TableMeta();
meta.name = name;
Map javaTypeMap = toJavaTypeMap(name);
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy