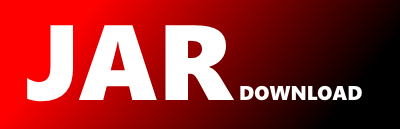
com.iiifi.kite.boot.utils.WebUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kite-boot Show documentation
Show all versions of kite-boot Show documentation
Spring Cloud Core Component Extension.
/*
* Copyright 2019-2025 the original author or authors.
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* https://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.iiifi.kite.boot.utils;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.core.annotation.AnnotatedElementUtils;
import org.springframework.util.Assert;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.context.request.RequestAttributes;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import org.springframework.web.method.HandlerMethod;
import com.iiifi.kite.boot.properties.KiteLocaleProperties;
import com.iiifi.kite.configuration.KiteHeader;
import com.iiifi.kite.core.spring.SpringContextUtils;
import com.iiifi.kite.core.util.ClassUtils;
import com.iiifi.kite.core.util.StringUtils;
import lombok.extern.slf4j.Slf4j;
/**
* Miscellaneous utilities for web applications.
*
* @author [email protected] 花朝
*/
@Slf4j
public class WebUtils extends org.springframework.web.util.WebUtils {
private static KiteLocaleProperties kiteLocaleProperties = SpringContextUtils.getBean(KiteLocaleProperties.class);
/**
* unknown Header key
*/
public static final String HEADER_IP_UNKNOWN = "unknown";
/**
* 判断是否ajax请求
* spring ajax 返回含有 ResponseBody 或者 RestController注解
*
* @param handlerMethod HandlerMethod
* @return 是否ajax请求
*/
public static boolean isBody(HandlerMethod handlerMethod) {
ResponseBody responseBody = ClassUtils.getAnnotation(handlerMethod, ResponseBody.class);
return responseBody != null;
}
/**
* 读取cookie
*
* @param name cookie name
* @return cookie value
*/
public static String getCookieVal(String name) {
HttpServletRequest request = WebUtils.getRequest();
Assert.notNull(request, "request from RequestContextHolder is null");
return getCookieVal(request, name);
}
/**
* 读取cookie
*
* @param request HttpServletRequest
* @param name cookie name
* @return cookie value
*/
public static String getCookieVal(HttpServletRequest request, String name) {
Cookie cookie = getCookie(request, name);
return cookie != null ? cookie.getValue() : null;
}
/**
* 清除 某个指定的cookie
*
* @param response HttpServletResponse
* @param key cookie key
*/
public static void removeCookie(HttpServletResponse response, String key) {
setCookie(response, key, null, 0);
}
/**
* 设置cookie
*
* @param response HttpServletResponse
* @param name cookie name
* @param value cookie value
* @param maxAgeInSeconds maxage
*/
public static void setCookie(HttpServletResponse response, String name, String value, int maxAgeInSeconds) {
Cookie cookie = new Cookie(name, value);
cookie.setPath("/");
cookie.setMaxAge(maxAgeInSeconds);
cookie.setHttpOnly(true);
response.addCookie(cookie);
}
/**
* 获取request
*
* @return 请求
*/
public static HttpServletRequest getRequest() {
RequestAttributes requestAttributes = RequestContextHolder.getRequestAttributes();
if (requestAttributes == null) {
return null;
}
return ((ServletRequestAttributes) requestAttributes).getRequest();
}
/**
* 获取response
*
* @return 回复
*/
public static HttpServletResponse getResponse() {
RequestAttributes requestAttributes = RequestContextHolder.getRequestAttributes();
if (requestAttributes == null) {
return null;
}
return ((ServletRequestAttributes) requestAttributes).getResponse();
}
/**
* 查询参数
*
* @param request request
* @return Map
*/
public static Map getRequestParamMap(HttpServletRequest request) {
Map map = new HashMap<>(16);
// 得到枚举类型的参数名称,参数名称若有重复的只能得到第一个
Enumeration enums = request.getParameterNames();
while (enums.hasMoreElements()) {
String paramName = enums.nextElement();
String paramValue = request.getParameter(paramName);
// 形成键值对应的map
map.put(paramName, paramValue);
}
return map;
}
/**
* 获取ip
*
* @return {String}
*/
public static String getIP() {
return getIP(WebUtils.getRequest());
}
/**
* 获取ip
*
* @param request HttpServletRequest
* @return {String}
*/
public static String getIP(HttpServletRequest request) {
if (request == null) {
return null;
}
String ip = request.getHeader("X-Requested-For");
if (StringUtils.isBlank(ip) || HEADER_IP_UNKNOWN.equalsIgnoreCase(ip)) {
ip = request.getHeader("X-Forwarded-For");
}
if (StringUtils.isBlank(ip) || HEADER_IP_UNKNOWN.equalsIgnoreCase(ip)) {
ip = request.getHeader("Proxy-Client-IP");
}
if (StringUtils.isBlank(ip) || HEADER_IP_UNKNOWN.equalsIgnoreCase(ip)) {
ip = request.getHeader("WL-Proxy-Client-IP");
}
if (StringUtils.isBlank(ip) || HEADER_IP_UNKNOWN.equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_CLIENT_IP");
}
if (StringUtils.isBlank(ip) || HEADER_IP_UNKNOWN.equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_X_FORWARDED_FOR");
}
if (StringUtils.isBlank(ip) || HEADER_IP_UNKNOWN.equalsIgnoreCase(ip)) {
ip = request.getRemoteAddr();
}
return StringUtils.isBlank(ip) ? null : ip.split(",")[0];
}
/**
* 获取 X-Language
*
* @return X-Language
*/
public static String getLanguage() {
String language = kiteLocaleProperties.getDefaultLocale().getLanguage();
HttpServletRequest request = WebUtils.getRequest();
if (request == null) {
return language;
}
language = request.getHeader(KiteHeader.X_LANGUAGE);
if (StringUtils.isBlank(language)) {
language = request.getParameter(kiteLocaleProperties.getParamName());
}
if (StringUtils.isBlank(language)) {
language = request.getLocale().getLanguage();
}
return language;
}
/**
* 获取Annotation
*
* @param method Method
* @param annotationType 注解类
* @param 泛型标记
* @return {Annotation}
*/
public static A getAnnotation(Method method, Class annotationType) {
// 先找方法,再找方法上的类
A annotation = method.getAnnotation(annotationType);
if (null != annotation) {
return annotation;
}
// 获取类上面的Annotation,可能包含组合注解,故采用spring的工具类
Class> beanType = method.getDeclaringClass();
return AnnotatedElementUtils.findMergedAnnotation(beanType, annotationType);
}
/**
* 获取Annotation
*
* @param handlerMethod HandlerMethod
* @param annotationType 注解类
* @param 泛型标记
* @return {Annotation}
*/
public static A getAnnotation(HandlerMethod handlerMethod, Class annotationType) {
// 先找方法,再找方法上的类
A annotation = handlerMethod.getMethodAnnotation(annotationType);
if (null != annotation) {
return annotation;
}
// 获取类上面的Annotation,可能包含组合注解,故采用spring的工具类
Class> beanType = handlerMethod.getBeanType();
return AnnotatedElementUtils.findMergedAnnotation(beanType, annotationType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy