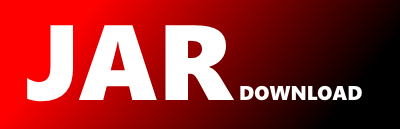
com.impossibl.postgres.jdbc.UDTGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pgjdbc-ng-udt Show documentation
Show all versions of pgjdbc-ng-udt Show documentation
UDT code generator for PGJDBC-NG driver
The newest version!
/**
* Copyright (c) 2013, impossibl.com
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of impossibl.com nor the names of its contributors may
* be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
package com.impossibl.postgres.jdbc;
import com.impossibl.postgres.types.CompositeType;
import com.impossibl.postgres.types.Registry;
import com.impossibl.postgres.types.Type;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import com.beust.jcommander.JCommander;
import com.beust.jcommander.Parameter;
import com.beust.jcommander.converters.CommaSeparatedConverter;
import org.stringtemplate.v4.AutoIndentWriter;
import org.stringtemplate.v4.ST;
import org.stringtemplate.v4.STGroup;
import org.stringtemplate.v4.STGroupFile;
/**
* UDT Generator Tool
*
* @author kdubb
*
*/
public class UDTGenerator {
@Parameter(names = "-types", description = "List of types to generate code for", required = true, converter = CommaSeparatedConverter.class)
public List typeNames;
@Parameter(names = {"-h", "--host" }, description = "Host of server connection")
public String host = "localhost";
@Parameter(names = {"-t", "--port" }, description = "Port of server connection")
public int port = 5432;
@Parameter(names = {"-d", "--database" }, description = "Database name", required = true)
public String database;
@Parameter(names = {"-u", "--user" }, description = "Database user", required = true)
public String user;
@Parameter(names = {"-p", "--password" }, description = "Database password", required = true)
public String password;
@Parameter(names = {"-o", "--out" }, description = "Target output directory (package directories are appended)")
public String targetDirName = ".";
@Parameter(names = {"-pkg", "--package" }, description = "Target Java package")
public String targetPackage = "";
/**
* Executes the code generation for the supplied parameters
*
* @return List of generated source files
* @throws SQLException
* @throws IOException
*/
List execute() throws SQLException, IOException {
String finalDirName = targetDirName + File.separator + targetPackage.replace('.', File.separatorChar);
File finalDir = new File(finalDirName);
if (!finalDir.isDirectory()) {
if (!finalDir.exists() && !finalDir.mkdirs()) {
throw new IllegalArgumentException("cannot make final output directory: " + finalDirName);
}
}
List generatedFiles = new ArrayList<>();
try (Connection conn = DriverManager.getConnection("jdbc:postgresql://" + host + ":" + port + "/" + database, user, password)) {
if (conn instanceof PGConnectionImpl == false) {
throw new IllegalStateException("Postgres driver must be PGJDBC-NG");
}
PGConnectionImpl pgConn = (PGConnectionImpl) conn;
Registry registry = pgConn.getRegistry();
for (String typeName : typeNames) {
File generatedFile = generate(registry, typeName, finalDirName);
if (generatedFile != null) {
generatedFiles.add(generatedFile);
}
}
}
return generatedFiles;
}
/**
* Generates a java for a specific composite type
*
* @param registry
* @param typeName
* @return Name of generated file
* @throws IOException
*/
File generate(Registry registry, String typeName, String dirName) throws IOException {
Type type = registry.loadType(typeName);
if (type == null) {
System.err.println("Type not found: " + typeName);
return null;
}
if (type instanceof CompositeType == false) {
System.err.println("Type not a composite: " + typeName);
return null;
}
CompositeType compType = (CompositeType) type;
File outFile = new File(dirName + File.separator + Names.getFullCamelCase(typeName) + ".java");
try (FileWriter out = new FileWriter(outFile)) {
STGroup udtTemplates = new STGroupFile("templates/udt.stg");
ST mainTemplate = udtTemplates.getInstanceOf("main");
mainTemplate.add("isInPackage", targetPackage != null && !targetPackage.isEmpty());
mainTemplate.add("package", targetPackage);
mainTemplate.add("type", type);
mainTemplate.add("typeInfo", new TypeInfo(compType));
mainTemplate.write(new AutoIndentWriter(out));
}
return outFile;
}
public static List run(String[] args) throws SQLException, IOException {
UDTGenerator tool = new UDTGenerator();
new JCommander(tool, args);
return tool.execute();
}
public static void main(String[] args) throws SQLException, IOException {
run(args);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy