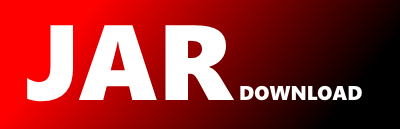
com.imsweb.algorithms.prcda.PrcdaUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algorithms Show documentation
Show all versions of algorithms Show documentation
Java implementation of cancer-related algorithms (NHIA, NAPIIA, Survival Time, etc...)
/*
* Copyright (C) 2013 Information Management Services, Inc.
*/
package com.imsweb.algorithms.prcda;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import com.imsweb.algorithms.StateCountyInputDto;
/**
* This class can be used to calculate PRCDA.
*
* View documentation
*
* Created on Aug 12, 2019 by howew
* @author howew
*/
public final class PrcdaUtils {
public static final String ALG_NAME = "NPCR PRCDA Linkage Program";
public static final String ALG_VERSION = "version 2.2 released in June 2022";
public static final String PRCDA_NO = "0";
public static final String PRCDA_YES = "1";
public static final String PRCDA_UNKNOWN = "9";
// States where every county is PRCDA
public static final List ENTIRE_STATE_PRCDA = Collections.unmodifiableList(Arrays.asList("AK", "CT", "NV", "OK", "SC"));
// States where every county is non-PRCDA
public static final List ENTIRE_STATE_NON_PRCDA;
static {
List nonPrcda = new ArrayList<>(Arrays.asList("AR", "DE", "DC", "GA", "HI", "IL", "KY", "MD", "MO", "NH", "NJ", "OH", "TN", "VT", "WV"));
List territory = Arrays.asList("AS", "GU", "MP", "PW", "PR", "UM", "VI", "FM", "MH", "TT");
List province = Arrays.asList("AB", "BC", "MB", "NB", "NL", "NS", "NT", "NU", "ON", "PE", "QC", "SK", "YT");
List military = Arrays.asList("AA", "AE", "AP");
//List UNKNOWN_VALUES_DO_NOT_INCLUDE_IN_NON_PRCDA = Arrays.asList("CD", "US", "XX", "YY", "ZZ");
nonPrcda.addAll(territory);
nonPrcda.addAll(province);
nonPrcda.addAll(military);
ENTIRE_STATE_NON_PRCDA = Collections.unmodifiableList(nonPrcda);
}
private static final PrcdaDataProvider _PROVIDER = new PrcdaDataProvider();
private PrcdaUtils() {
// no instances of this class allowed!
}
/**
* Calculates PRCDA and PRCDA2017 for the provided record
*
* The provided record doesn't need to contain all the input variables, but the algorithm will use the following ones:
*
* - addrAtDxState (#80)
* - countyAtDxAnalysis (#89)
*
* All those properties are defined as constants in this class.
*
* PRCDA and PRCDA2017 will have one the following values:
* 0 = Not a PRCDA county
* 1 = PRCDA county
* 9 = Unknown
*
* @param input a StateCountyInputDto
input object
* @return PrcdaOutputDto
output object
*/
public static PrcdaOutputDto computePrcda(StateCountyInputDto input) {
PrcdaOutputDto result = new PrcdaOutputDto();
input.applyRecodes();
if (ENTIRE_STATE_PRCDA.contains(input.getAddressAtDxState())) {
result.setPrcda(PRCDA_YES);
result.setPrcda2017(PRCDA_YES);
}
else if (ENTIRE_STATE_NON_PRCDA.contains(input.getAddressAtDxState())) {
result.setPrcda(PRCDA_NO);
result.setPrcda2017(PRCDA_NO);
}
else {
if (input.hasInvalidStateOrCounty() || input.hasUnknownStateOrCounty() || input.countyIsNotReported()) {
result.setPrcda(PRCDA_UNKNOWN);
result.setPrcda2017(PRCDA_UNKNOWN);
}
else {
result.setPrcda(_PROVIDER.getPrcda(input.getAddressAtDxState(), input.getCountyAtDxAnalysis()));
result.setPrcda2017(_PROVIDER.getPrcda2017(input.getAddressAtDxState(), input.getCountyAtDxAnalysis()));
}
}
// get methods should never return null, but let's make sure we don't return null value anyway
if (result.getPrcda() == null)
result.setPrcda(PRCDA_NO);
if (result.getPrcda2017() == null)
result.setPrcda2017(PRCDA_NO);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy