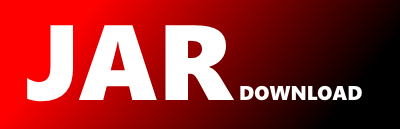
com.infobip.kafkistry.kafka.ops.TopicOffsetsOps.kt Maven / Gradle / Ivy
package com.infobip.kafkistry.kafka.ops
import com.infobip.kafkistry.kafka.Partition
import com.infobip.kafkistry.kafka.PartitionOffsets
import com.infobip.kafkistry.model.TopicName
import com.infobip.kafkistry.service.KafkistryClusterReadException
import org.apache.kafka.clients.admin.DescribeTopicsOptions
import org.apache.kafka.clients.admin.ListOffsetsOptions
import org.apache.kafka.clients.admin.ListOffsetsResult
import org.apache.kafka.clients.admin.OffsetSpec
import org.apache.kafka.common.TopicPartition
import java.util.concurrent.CompletableFuture
class TopicOffsetsOps(
clientCtx: ClientCtx,
): BaseOps(clientCtx) {
fun topicsOffsets(topicNames: List): CompletableFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy