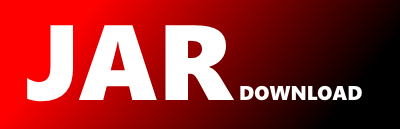
com.infobip.api.SendSmsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infobip-api-java-client Show documentation
Show all versions of infobip-api-java-client Show documentation
API client in Java for Infobip's API (http://dev.infobip.com/).
/*
* Infobip Client API Libraries OpenAPI Specification
* OpenAPI specification containing public endpoints supported in client API libraries.
*
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.infobip.api;
import com.google.gson.reflect.TypeToken;
import com.infobip.ApiCallback;
import com.infobip.ApiClient;
import com.infobip.ApiException;
import com.infobip.ApiResponse;
import com.infobip.Configuration;
import com.infobip.Pair;
import com.infobip.model.SmsAdvancedBinaryRequest;
import com.infobip.model.SmsAdvancedTextualRequest;
import com.infobip.model.SmsDeliveryResult;
import com.infobip.model.SmsLogsResponse;
import com.infobip.model.SmsPreviewRequest;
import com.infobip.model.SmsPreviewResponse;
import com.infobip.model.SmsResponse;
import java.lang.reflect.Type;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SendSmsApi {
private ApiClient localVarApiClient;
public SendSmsApi() {
this(Configuration.getDefaultApiClient());
}
public SendSmsApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
/**
* Build call for getOutboundSmsMessageDeliveryReports.
*
* @param bulkId ID of bulk which delivery report is requested. (optional)
* @param messageId ID of SMS which delivery report is requested. (optional)
* @param limit Maximal number of delivery reports that will be returned. (optional)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call getOutboundSmsMessageDeliveryReportsCall(
String bulkId, String messageId, Integer limit, final ApiCallback _callback)
throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/sms/1/reports";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (bulkId != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("bulkId", bulkId));
}
if (messageId != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("messageId", messageId));
}
if (limit != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("limit", limit));
}
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {"application/json", "application/xml"};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {};
final String localVarContentType =
localVarApiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
String[] localVarAuthNames =
new String[] {"APIKeyHeader", "Basic", "IBSSOTokenHeader", "OAuth2"};
return localVarApiClient.buildCall(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAuthNames,
_callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getOutboundSmsMessageDeliveryReportsValidateBeforeCall(
String bulkId, String messageId, Integer limit, final ApiCallback _callback)
throws ApiException {
okhttp3.Call localVarCall =
getOutboundSmsMessageDeliveryReportsCall(bulkId, messageId, limit, _callback);
return localVarCall;
}
/**
* Get outbound SMS message delivery reports. If you are for any reason unable to receive real
* time delivery reports on your endpoint, you can use this API method to learn if and when the
* message has been delivered to the recipient. Each request will return a batch of delivery
* reports - only once. The following API request will return only new reports that arrived since
* the last API request.
*
* @param bulkId ID of bulk which delivery report is requested. (optional)
* @param messageId ID of SMS which delivery report is requested. (optional)
* @param limit Maximal number of delivery reports that will be returned. (optional)
* @return SmsDeliveryResult
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public SmsDeliveryResult getOutboundSmsMessageDeliveryReports(
String bulkId, String messageId, Integer limit) throws ApiException {
ApiResponse localVarResp =
getOutboundSmsMessageDeliveryReportsWithHttpInfo(bulkId, messageId, limit);
return localVarResp.getData();
}
/**
* Get outbound SMS message delivery reports. If you are for any reason unable to receive real
* time delivery reports on your endpoint, you can use this API method to learn if and when the
* message has been delivered to the recipient. Each request will return a batch of delivery
* reports - only once. The following API request will return only new reports that arrived since
* the last API request.
*
* @param bulkId ID of bulk which delivery report is requested. (optional)
* @param messageId ID of SMS which delivery report is requested. (optional)
* @param limit Maximal number of delivery reports that will be returned. (optional)
* @return ApiResponse<SmsDeliveryResult>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public ApiResponse getOutboundSmsMessageDeliveryReportsWithHttpInfo(
String bulkId, String messageId, Integer limit) throws ApiException {
okhttp3.Call localVarCall =
getOutboundSmsMessageDeliveryReportsValidateBeforeCall(bulkId, messageId, limit, null);
Type localVarReturnType = new TypeToken() {}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* Get outbound SMS message delivery reports (asynchronously). If you are for any reason unable to
* receive real time delivery reports on your endpoint, you can use this API method to learn if
* and when the message has been delivered to the recipient. Each request will return a batch of
* delivery reports - only once. The following API request will return only new reports that
* arrived since the last API request.
*
* @param bulkId ID of bulk which delivery report is requested. (optional)
* @param messageId ID of SMS which delivery report is requested. (optional)
* @param limit Maximal number of delivery reports that will be returned. (optional)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call getOutboundSmsMessageDeliveryReportsAsync(
String bulkId,
String messageId,
Integer limit,
final ApiCallback _callback)
throws ApiException {
okhttp3.Call localVarCall =
getOutboundSmsMessageDeliveryReportsValidateBeforeCall(bulkId, messageId, limit, _callback);
Type localVarReturnType = new TypeToken() {}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for getOutboundSmsMessageLogs.
*
* @param from Sender address. (optional)
* @param to Destination address. (optional)
* @param bulkId Bulk ID for which log is requested. (optional)
* @param messageId SMS ID for which log is requested. (optional)
* @param generalStatus Sent SMS status. (optional)
* @param sentSince Lower limit on date and time of sending SMS. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. (optional)
* @param sentUntil Upper limit on date and time of sending SMS. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. (optional)
* @param limit Maximal number of messages in returned logs. Limit should be between `1`
* and `1000`. If you want to fetch more than 1000 logs you can retrieve them in
* pages using `sentSince` and `sentUntil` parameters. Defaults to
* `50`. (optional)
* @param mcc Mobile country code. (optional)
* @param mnc Mobile network code. (optional)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call getOutboundSmsMessageLogsCall(
String from,
String to,
List bulkId,
List messageId,
String generalStatus,
OffsetDateTime sentSince,
OffsetDateTime sentUntil,
Integer limit,
String mcc,
String mnc,
final ApiCallback _callback)
throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/sms/1/logs";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (from != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("from", from));
}
if (to != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("to", to));
}
if (bulkId != null) {
localVarCollectionQueryParams.addAll(
localVarApiClient.parameterToPairs("multi", "bulkId", bulkId));
}
if (messageId != null) {
localVarCollectionQueryParams.addAll(
localVarApiClient.parameterToPairs("multi", "messageId", messageId));
}
if (generalStatus != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("generalStatus", generalStatus));
}
if (sentSince != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("sentSince", sentSince));
}
if (sentUntil != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("sentUntil", sentUntil));
}
if (limit != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("limit", limit));
}
if (mcc != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("mcc", mcc));
}
if (mnc != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("mnc", mnc));
}
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {"application/json", "application/xml"};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {};
final String localVarContentType =
localVarApiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
String[] localVarAuthNames =
new String[] {"APIKeyHeader", "Basic", "IBSSOTokenHeader", "OAuth2"};
return localVarApiClient.buildCall(
localVarPath,
"GET",
localVarQueryParams,
localVarCollectionQueryParams,
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAuthNames,
_callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getOutboundSmsMessageLogsValidateBeforeCall(
String from,
String to,
List bulkId,
List messageId,
String generalStatus,
OffsetDateTime sentSince,
OffsetDateTime sentUntil,
Integer limit,
String mcc,
String mnc,
final ApiCallback _callback)
throws ApiException {
okhttp3.Call localVarCall =
getOutboundSmsMessageLogsCall(
from,
to,
bulkId,
messageId,
generalStatus,
sentSince,
sentUntil,
limit,
mcc,
mnc,
_callback);
return localVarCall;
}
/**
* Get outbound SMS message logs. You should use this method for displaying logs in the user
* interface or for some other less frequent usage. See [message delivery
* reports](#channels/sms/get-outbound-sms-message-delivery-reports) if your use case is to verify
* message delivery.
*
* @param from Sender address. (optional)
* @param to Destination address. (optional)
* @param bulkId Bulk ID for which log is requested. (optional)
* @param messageId SMS ID for which log is requested. (optional)
* @param generalStatus Sent SMS status. (optional)
* @param sentSince Lower limit on date and time of sending SMS. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. (optional)
* @param sentUntil Upper limit on date and time of sending SMS. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. (optional)
* @param limit Maximal number of messages in returned logs. Limit should be between `1`
* and `1000`. If you want to fetch more than 1000 logs you can retrieve them in
* pages using `sentSince` and `sentUntil` parameters. Defaults to
* `50`. (optional)
* @param mcc Mobile country code. (optional)
* @param mnc Mobile network code. (optional)
* @return SmsLogsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public SmsLogsResponse getOutboundSmsMessageLogs(
String from,
String to,
List bulkId,
List messageId,
String generalStatus,
OffsetDateTime sentSince,
OffsetDateTime sentUntil,
Integer limit,
String mcc,
String mnc)
throws ApiException {
ApiResponse localVarResp =
getOutboundSmsMessageLogsWithHttpInfo(
from, to, bulkId, messageId, generalStatus, sentSince, sentUntil, limit, mcc, mnc);
return localVarResp.getData();
}
/**
* Get outbound SMS message logs. You should use this method for displaying logs in the user
* interface or for some other less frequent usage. See [message delivery
* reports](#channels/sms/get-outbound-sms-message-delivery-reports) if your use case is to verify
* message delivery.
*
* @param from Sender address. (optional)
* @param to Destination address. (optional)
* @param bulkId Bulk ID for which log is requested. (optional)
* @param messageId SMS ID for which log is requested. (optional)
* @param generalStatus Sent SMS status. (optional)
* @param sentSince Lower limit on date and time of sending SMS. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. (optional)
* @param sentUntil Upper limit on date and time of sending SMS. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. (optional)
* @param limit Maximal number of messages in returned logs. Limit should be between `1`
* and `1000`. If you want to fetch more than 1000 logs you can retrieve them in
* pages using `sentSince` and `sentUntil` parameters. Defaults to
* `50`. (optional)
* @param mcc Mobile country code. (optional)
* @param mnc Mobile network code. (optional)
* @return ApiResponse<SmsLogsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public ApiResponse getOutboundSmsMessageLogsWithHttpInfo(
String from,
String to,
List bulkId,
List messageId,
String generalStatus,
OffsetDateTime sentSince,
OffsetDateTime sentUntil,
Integer limit,
String mcc,
String mnc)
throws ApiException {
okhttp3.Call localVarCall =
getOutboundSmsMessageLogsValidateBeforeCall(
from,
to,
bulkId,
messageId,
generalStatus,
sentSince,
sentUntil,
limit,
mcc,
mnc,
null);
Type localVarReturnType = new TypeToken() {}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* Get outbound SMS message logs (asynchronously). You should use this method for displaying logs
* in the user interface or for some other less frequent usage. See [message delivery
* reports](#channels/sms/get-outbound-sms-message-delivery-reports) if your use case is to verify
* message delivery.
*
* @param from Sender address. (optional)
* @param to Destination address. (optional)
* @param bulkId Bulk ID for which log is requested. (optional)
* @param messageId SMS ID for which log is requested. (optional)
* @param generalStatus Sent SMS status. (optional)
* @param sentSince Lower limit on date and time of sending SMS. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. (optional)
* @param sentUntil Upper limit on date and time of sending SMS. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`. (optional)
* @param limit Maximal number of messages in returned logs. Limit should be between `1`
* and `1000`. If you want to fetch more than 1000 logs you can retrieve them in
* pages using `sentSince` and `sentUntil` parameters. Defaults to
* `50`. (optional)
* @param mcc Mobile country code. (optional)
* @param mnc Mobile network code. (optional)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call getOutboundSmsMessageLogsAsync(
String from,
String to,
List bulkId,
List messageId,
String generalStatus,
OffsetDateTime sentSince,
OffsetDateTime sentUntil,
Integer limit,
String mcc,
String mnc,
final ApiCallback _callback)
throws ApiException {
okhttp3.Call localVarCall =
getOutboundSmsMessageLogsValidateBeforeCall(
from,
to,
bulkId,
messageId,
generalStatus,
sentSince,
sentUntil,
limit,
mcc,
mnc,
_callback);
Type localVarReturnType = new TypeToken() {}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for previewSmsMessage.
*
* @param smsPreviewRequest (optional)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call previewSmsMessageCall(
SmsPreviewRequest smsPreviewRequest, final ApiCallback _callback) throws ApiException {
Object localVarPostBody = smsPreviewRequest;
// create path and map variables
String localVarPath = "/sms/1/preview";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {"application/json", "application/xml"};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {"application/json", "application/xml"};
final String localVarContentType =
localVarApiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
String[] localVarAuthNames =
new String[] {"APIKeyHeader", "Basic", "IBSSOTokenHeader", "OAuth2"};
return localVarApiClient.buildCall(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAuthNames,
_callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call previewSmsMessageValidateBeforeCall(
SmsPreviewRequest smsPreviewRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = previewSmsMessageCall(smsPreviewRequest, _callback);
return localVarCall;
}
/**
* Preview SMS message. Avoid unpleasant surprises and check how different message configurations
* will affect your message text, number of characters and message parts.
*
* @param smsPreviewRequest (optional)
* @return SmsPreviewResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public SmsPreviewResponse previewSmsMessage(SmsPreviewRequest smsPreviewRequest)
throws ApiException {
ApiResponse localVarResp = previewSmsMessageWithHttpInfo(smsPreviewRequest);
return localVarResp.getData();
}
/**
* Preview SMS message. Avoid unpleasant surprises and check how different message configurations
* will affect your message text, number of characters and message parts.
*
* @param smsPreviewRequest (optional)
* @return ApiResponse<SmsPreviewResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public ApiResponse previewSmsMessageWithHttpInfo(
SmsPreviewRequest smsPreviewRequest) throws ApiException {
okhttp3.Call localVarCall = previewSmsMessageValidateBeforeCall(smsPreviewRequest, null);
Type localVarReturnType = new TypeToken() {}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* Preview SMS message (asynchronously). Avoid unpleasant surprises and check how different
* message configurations will affect your message text, number of characters and message parts.
*
* @param smsPreviewRequest (optional)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call previewSmsMessageAsync(
SmsPreviewRequest smsPreviewRequest, final ApiCallback _callback)
throws ApiException {
okhttp3.Call localVarCall = previewSmsMessageValidateBeforeCall(smsPreviewRequest, _callback);
Type localVarReturnType = new TypeToken() {}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for sendBinarySmsMessage.
*
* @param smsAdvancedBinaryRequest (optional)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call sendBinarySmsMessageCall(
SmsAdvancedBinaryRequest smsAdvancedBinaryRequest, final ApiCallback _callback)
throws ApiException {
Object localVarPostBody = smsAdvancedBinaryRequest;
// create path and map variables
String localVarPath = "/sms/2/binary/advanced";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {"application/json", "application/xml"};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {"application/json", "application/xml"};
final String localVarContentType =
localVarApiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
String[] localVarAuthNames =
new String[] {"APIKeyHeader", "Basic", "IBSSOTokenHeader", "OAuth2"};
return localVarApiClient.buildCall(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAuthNames,
_callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call sendBinarySmsMessageValidateBeforeCall(
SmsAdvancedBinaryRequest smsAdvancedBinaryRequest, final ApiCallback _callback)
throws ApiException {
okhttp3.Call localVarCall = sendBinarySmsMessageCall(smsAdvancedBinaryRequest, _callback);
return localVarCall;
}
/**
* Send binary SMS message. Send single or multiple binary messages to one or more destination
* address.
*
* @param smsAdvancedBinaryRequest (optional)
* @return SmsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public SmsResponse sendBinarySmsMessage(SmsAdvancedBinaryRequest smsAdvancedBinaryRequest)
throws ApiException {
ApiResponse localVarResp =
sendBinarySmsMessageWithHttpInfo(smsAdvancedBinaryRequest);
return localVarResp.getData();
}
/**
* Send binary SMS message. Send single or multiple binary messages to one or more destination
* address.
*
* @param smsAdvancedBinaryRequest (optional)
* @return ApiResponse<SmsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public ApiResponse sendBinarySmsMessageWithHttpInfo(
SmsAdvancedBinaryRequest smsAdvancedBinaryRequest) throws ApiException {
okhttp3.Call localVarCall =
sendBinarySmsMessageValidateBeforeCall(smsAdvancedBinaryRequest, null);
Type localVarReturnType = new TypeToken() {}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* Send binary SMS message (asynchronously). Send single or multiple binary messages to one or
* more destination address.
*
* @param smsAdvancedBinaryRequest (optional)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call sendBinarySmsMessageAsync(
SmsAdvancedBinaryRequest smsAdvancedBinaryRequest, final ApiCallback _callback)
throws ApiException {
okhttp3.Call localVarCall =
sendBinarySmsMessageValidateBeforeCall(smsAdvancedBinaryRequest, _callback);
Type localVarReturnType = new TypeToken() {}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for sendSmsMessage.
*
* @param smsAdvancedTextualRequest (optional)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call sendSmsMessageCall(
SmsAdvancedTextualRequest smsAdvancedTextualRequest, final ApiCallback _callback)
throws ApiException {
Object localVarPostBody = smsAdvancedTextualRequest;
// create path and map variables
String localVarPath = "/sms/2/text/advanced";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {"application/json", "application/xml"};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {"application/json", "application/xml"};
final String localVarContentType =
localVarApiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
String[] localVarAuthNames =
new String[] {"APIKeyHeader", "Basic", "IBSSOTokenHeader", "OAuth2"};
return localVarApiClient.buildCall(
localVarPath,
"POST",
localVarQueryParams,
localVarCollectionQueryParams,
localVarPostBody,
localVarHeaderParams,
localVarCookieParams,
localVarFormParams,
localVarAuthNames,
_callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call sendSmsMessageValidateBeforeCall(
SmsAdvancedTextualRequest smsAdvancedTextualRequest, final ApiCallback _callback)
throws ApiException {
okhttp3.Call localVarCall = sendSmsMessageCall(smsAdvancedTextualRequest, _callback);
return localVarCall;
}
/**
* Send SMS message. 99% of all use cases can be achieved by using this API method. Everything
* from sending a simple single message to a single destination, up to batch sending of
* personalized messages to the thousands of recipients with a single API request. Language,
* transliteration, scheduling and every advanced feature you can think of is supported.
*
* @param smsAdvancedTextualRequest (optional)
* @return SmsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public SmsResponse sendSmsMessage(SmsAdvancedTextualRequest smsAdvancedTextualRequest)
throws ApiException {
ApiResponse localVarResp = sendSmsMessageWithHttpInfo(smsAdvancedTextualRequest);
return localVarResp.getData();
}
/**
* Send SMS message. 99% of all use cases can be achieved by using this API method. Everything
* from sending a simple single message to a single destination, up to batch sending of
* personalized messages to the thousands of recipients with a single API request. Language,
* transliteration, scheduling and every advanced feature you can think of is supported.
*
* @param smsAdvancedTextualRequest (optional)
* @return ApiResponse<SmsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the
* response body
* @see Learn more about SMS channel and use cases
*/
public ApiResponse sendSmsMessageWithHttpInfo(
SmsAdvancedTextualRequest smsAdvancedTextualRequest) throws ApiException {
okhttp3.Call localVarCall = sendSmsMessageValidateBeforeCall(smsAdvancedTextualRequest, null);
Type localVarReturnType = new TypeToken() {}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* Send SMS message (asynchronously). 99% of all use cases can be achieved by using this API
* method. Everything from sending a simple single message to a single destination, up to batch
* sending of personalized messages to the thousands of recipients with a single API request.
* Language, transliteration, scheduling and every advanced feature you can think of is supported.
*
* @param smsAdvancedTextualRequest (optional)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
* @see Learn more about SMS channel and use cases
*/
public okhttp3.Call sendSmsMessageAsync(
SmsAdvancedTextualRequest smsAdvancedTextualRequest, final ApiCallback _callback)
throws ApiException {
okhttp3.Call localVarCall =
sendSmsMessageValidateBeforeCall(smsAdvancedTextualRequest, _callback);
Type localVarReturnType = new TypeToken() {}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy