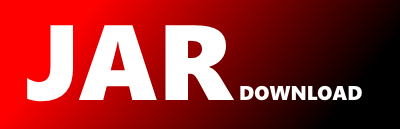
com.infobip.model.SmsInboundMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infobip-api-java-client Show documentation
Show all versions of infobip-api-java-client Show documentation
API client in Java for Infobip's API (http://dev.infobip.com/).
/*
* Infobip Client API Libraries OpenAPI Specification
* OpenAPI specification containing public endpoints supported in client API libraries.
*
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.infobip.model;
import com.google.gson.annotations.SerializedName;
import java.time.OffsetDateTime;
import java.util.Objects;
/** SmsInboundMessage */
public class SmsInboundMessage {
public static final String SERIALIZED_NAME_CALLBACK_DATA = "callbackData";
@SerializedName(SERIALIZED_NAME_CALLBACK_DATA)
private String callbackData;
public static final String SERIALIZED_NAME_CLEAN_TEXT = "cleanText";
@SerializedName(SERIALIZED_NAME_CLEAN_TEXT)
private String cleanText;
public static final String SERIALIZED_NAME_FROM = "from";
@SerializedName(SERIALIZED_NAME_FROM)
private String from;
public static final String SERIALIZED_NAME_KEYWORD = "keyword";
@SerializedName(SERIALIZED_NAME_KEYWORD)
private String keyword;
public static final String SERIALIZED_NAME_MESSAGE_ID = "messageId";
@SerializedName(SERIALIZED_NAME_MESSAGE_ID)
private String messageId;
public static final String SERIALIZED_NAME_PRICE = "price";
@SerializedName(SERIALIZED_NAME_PRICE)
private SmsPrice price = null;
public static final String SERIALIZED_NAME_RECEIVED_AT = "receivedAt";
@SerializedName(SERIALIZED_NAME_RECEIVED_AT)
private OffsetDateTime receivedAt;
public static final String SERIALIZED_NAME_SMS_COUNT = "smsCount";
@SerializedName(SERIALIZED_NAME_SMS_COUNT)
private Integer smsCount;
public static final String SERIALIZED_NAME_TEXT = "text";
@SerializedName(SERIALIZED_NAME_TEXT)
private String text;
public static final String SERIALIZED_NAME_TO = "to";
@SerializedName(SERIALIZED_NAME_TO)
private String to;
/**
* Custom callback data can be inserted during the setup phase.
*
* @return callbackData
*/
public String getCallbackData() {
return callbackData;
}
/**
* Text of received message without a keyword (if a keyword was sent).
*
* @return cleanText
*/
public String getCleanText() {
return cleanText;
}
/**
* Sender ID that can be alphanumeric or numeric.
*
* @return from
*/
public String getFrom() {
return from;
}
/**
* Keyword extracted from the message text.
*
* @return keyword
*/
public String getKeyword() {
return keyword;
}
/**
* The ID that uniquely identifies the received message.
*
* @return messageId
*/
public String getMessageId() {
return messageId;
}
public SmsInboundMessage price(SmsPrice price) {
this.price = price;
return this;
}
/**
* Get price
*
* @return price
*/
public SmsPrice getPrice() {
return price;
}
public void setPrice(SmsPrice price) {
this.price = price;
}
/**
* Tells when Infobip platform received the message. It has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`.
*
* @return receivedAt
*/
public OffsetDateTime getReceivedAt() {
return receivedAt;
}
/**
* The number of sent message segments.
*
* @return smsCount
*/
public Integer getSmsCount() {
return smsCount;
}
/**
* Full text of the received message.
*
* @return text
*/
public String getText() {
return text;
}
/**
* The message destination address.
*
* @return to
*/
public String getTo() {
return to;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SmsInboundMessage smsInboundMessage = (SmsInboundMessage) o;
return Objects.equals(this.callbackData, smsInboundMessage.callbackData)
&& Objects.equals(this.cleanText, smsInboundMessage.cleanText)
&& Objects.equals(this.from, smsInboundMessage.from)
&& Objects.equals(this.keyword, smsInboundMessage.keyword)
&& Objects.equals(this.messageId, smsInboundMessage.messageId)
&& Objects.equals(this.price, smsInboundMessage.price)
&& Objects.equals(this.receivedAt, smsInboundMessage.receivedAt)
&& Objects.equals(this.smsCount, smsInboundMessage.smsCount)
&& Objects.equals(this.text, smsInboundMessage.text)
&& Objects.equals(this.to, smsInboundMessage.to);
}
@Override
public int hashCode() {
return Objects.hash(
callbackData, cleanText, from, keyword, messageId, price, receivedAt, smsCount, text, to);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SmsInboundMessage {\n");
sb.append(" callbackData: ").append(toIndentedString(callbackData)).append("\n");
sb.append(" cleanText: ").append(toIndentedString(cleanText)).append("\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" keyword: ").append(toIndentedString(keyword)).append("\n");
sb.append(" messageId: ").append(toIndentedString(messageId)).append("\n");
sb.append(" price: ").append(toIndentedString(price)).append("\n");
sb.append(" receivedAt: ").append(toIndentedString(receivedAt)).append("\n");
sb.append(" smsCount: ").append(toIndentedString(smsCount)).append("\n");
sb.append(" text: ").append(toIndentedString(text)).append("\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy