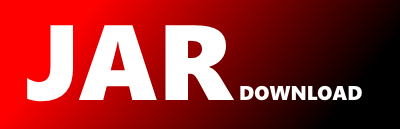
com.infobip.model.SmsLog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infobip-api-java-client Show documentation
Show all versions of infobip-api-java-client Show documentation
API client in Java for Infobip's API (http://dev.infobip.com/).
/*
* Infobip Client API Libraries OpenAPI Specification
* OpenAPI specification containing public endpoints supported in client API libraries.
*
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.infobip.model;
import com.google.gson.annotations.SerializedName;
import java.time.OffsetDateTime;
import java.util.Objects;
/** SmsLog */
public class SmsLog {
public static final String SERIALIZED_NAME_BULK_ID = "bulkId";
@SerializedName(SERIALIZED_NAME_BULK_ID)
private String bulkId;
public static final String SERIALIZED_NAME_DONE_AT = "doneAt";
@SerializedName(SERIALIZED_NAME_DONE_AT)
private OffsetDateTime doneAt;
public static final String SERIALIZED_NAME_ERROR = "error";
@SerializedName(SERIALIZED_NAME_ERROR)
private SmsError error = null;
public static final String SERIALIZED_NAME_FROM = "from";
@SerializedName(SERIALIZED_NAME_FROM)
private String from;
public static final String SERIALIZED_NAME_MCC_MNC = "mccMnc";
@SerializedName(SERIALIZED_NAME_MCC_MNC)
private String mccMnc;
public static final String SERIALIZED_NAME_MESSAGE_ID = "messageId";
@SerializedName(SERIALIZED_NAME_MESSAGE_ID)
private String messageId;
public static final String SERIALIZED_NAME_PRICE = "price";
@SerializedName(SERIALIZED_NAME_PRICE)
private SmsPrice price = null;
public static final String SERIALIZED_NAME_SENT_AT = "sentAt";
@SerializedName(SERIALIZED_NAME_SENT_AT)
private OffsetDateTime sentAt;
public static final String SERIALIZED_NAME_SMS_COUNT = "smsCount";
@SerializedName(SERIALIZED_NAME_SMS_COUNT)
private Integer smsCount;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private SmsStatus status = null;
public static final String SERIALIZED_NAME_TEXT = "text";
@SerializedName(SERIALIZED_NAME_TEXT)
private String text;
public static final String SERIALIZED_NAME_TO = "to";
@SerializedName(SERIALIZED_NAME_TO)
private String to;
/**
* The ID that uniquely identifies the request.
*
* @return bulkId
*/
public String getBulkId() {
return bulkId;
}
/**
* Tells when the SMS was finished processing by Infobip (i.e. delivered to the destination,
* delivered to the destination network, etc.). Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`.
*
* @return doneAt
*/
public OffsetDateTime getDoneAt() {
return doneAt;
}
public SmsLog error(SmsError error) {
this.error = error;
return this;
}
/**
* Get error
*
* @return error
*/
public SmsError getError() {
return error;
}
public void setError(SmsError error) {
this.error = error;
}
/**
* Sender ID that can be alphanumeric or numeric.
*
* @return from
*/
public String getFrom() {
return from;
}
/**
* Mobile country and network codes.
*
* @return mccMnc
*/
public String getMccMnc() {
return mccMnc;
}
/**
* The ID that uniquely identifies the message sent.
*
* @return messageId
*/
public String getMessageId() {
return messageId;
}
public SmsLog price(SmsPrice price) {
this.price = price;
return this;
}
/**
* Get price
*
* @return price
*/
public SmsPrice getPrice() {
return price;
}
public void setPrice(SmsPrice price) {
this.price = price;
}
/**
* Tells when the SMS was sent. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`.
*
* @return sentAt
*/
public OffsetDateTime getSentAt() {
return sentAt;
}
/**
* The number of sent message segments.
*
* @return smsCount
*/
public Integer getSmsCount() {
return smsCount;
}
public SmsLog status(SmsStatus status) {
this.status = status;
return this;
}
/**
* Get status
*
* @return status
*/
public SmsStatus getStatus() {
return status;
}
public void setStatus(SmsStatus status) {
this.status = status;
}
/**
* Text of the message that was sent.
*
* @return text
*/
public String getText() {
return text;
}
/**
* The message destination address.
*
* @return to
*/
public String getTo() {
return to;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SmsLog smsLog = (SmsLog) o;
return Objects.equals(this.bulkId, smsLog.bulkId)
&& Objects.equals(this.doneAt, smsLog.doneAt)
&& Objects.equals(this.error, smsLog.error)
&& Objects.equals(this.from, smsLog.from)
&& Objects.equals(this.mccMnc, smsLog.mccMnc)
&& Objects.equals(this.messageId, smsLog.messageId)
&& Objects.equals(this.price, smsLog.price)
&& Objects.equals(this.sentAt, smsLog.sentAt)
&& Objects.equals(this.smsCount, smsLog.smsCount)
&& Objects.equals(this.status, smsLog.status)
&& Objects.equals(this.text, smsLog.text)
&& Objects.equals(this.to, smsLog.to);
}
@Override
public int hashCode() {
return Objects.hash(
bulkId, doneAt, error, from, mccMnc, messageId, price, sentAt, smsCount, status, text, to);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SmsLog {\n");
sb.append(" bulkId: ").append(toIndentedString(bulkId)).append("\n");
sb.append(" doneAt: ").append(toIndentedString(doneAt)).append("\n");
sb.append(" error: ").append(toIndentedString(error)).append("\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" mccMnc: ").append(toIndentedString(mccMnc)).append("\n");
sb.append(" messageId: ").append(toIndentedString(messageId)).append("\n");
sb.append(" price: ").append(toIndentedString(price)).append("\n");
sb.append(" sentAt: ").append(toIndentedString(sentAt)).append("\n");
sb.append(" smsCount: ").append(toIndentedString(smsCount)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" text: ").append(toIndentedString(text)).append("\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy