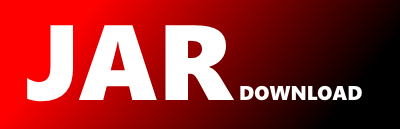
com.infobip.model.SmsReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infobip-api-java-client Show documentation
Show all versions of infobip-api-java-client Show documentation
API client in Java for Infobip's API (http://dev.infobip.com/).
/*
* Infobip Client API Libraries OpenAPI Specification
* OpenAPI specification containing public endpoints supported in client API libraries.
*
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.infobip.model;
import com.google.gson.annotations.SerializedName;
import java.time.OffsetDateTime;
import java.util.Objects;
/** SmsReport */
public class SmsReport {
public static final String SERIALIZED_NAME_BULK_ID = "bulkId";
@SerializedName(SERIALIZED_NAME_BULK_ID)
private String bulkId;
public static final String SERIALIZED_NAME_CALLBACK_DATA = "callbackData";
@SerializedName(SERIALIZED_NAME_CALLBACK_DATA)
private String callbackData;
public static final String SERIALIZED_NAME_DONE_AT = "doneAt";
@SerializedName(SERIALIZED_NAME_DONE_AT)
private OffsetDateTime doneAt;
public static final String SERIALIZED_NAME_ERROR = "error";
@SerializedName(SERIALIZED_NAME_ERROR)
private SmsError error = null;
public static final String SERIALIZED_NAME_FROM = "from";
@SerializedName(SERIALIZED_NAME_FROM)
private String from;
public static final String SERIALIZED_NAME_MCC_MNC = "mccMnc";
@SerializedName(SERIALIZED_NAME_MCC_MNC)
private String mccMnc;
public static final String SERIALIZED_NAME_MESSAGE_ID = "messageId";
@SerializedName(SERIALIZED_NAME_MESSAGE_ID)
private String messageId;
public static final String SERIALIZED_NAME_PRICE = "price";
@SerializedName(SERIALIZED_NAME_PRICE)
private SmsPrice price = null;
public static final String SERIALIZED_NAME_SENT_AT = "sentAt";
@SerializedName(SERIALIZED_NAME_SENT_AT)
private OffsetDateTime sentAt;
public static final String SERIALIZED_NAME_SMS_COUNT = "smsCount";
@SerializedName(SERIALIZED_NAME_SMS_COUNT)
private Integer smsCount;
public static final String SERIALIZED_NAME_STATUS = "status";
@SerializedName(SERIALIZED_NAME_STATUS)
private SmsStatus status = null;
public static final String SERIALIZED_NAME_TO = "to";
@SerializedName(SERIALIZED_NAME_TO)
private String to;
/**
* Bulk ID.
*
* @return bulkId
*/
public String getBulkId() {
return bulkId;
}
/**
* Callback data sent through `callbackData` field in fully featured SMS message.
*
* @return callbackData
*/
public String getCallbackData() {
return callbackData;
}
/**
* Tells when the SMS was finished processing by Infobip (i.e., delivered to the destination,
* delivered to the destination network, etc.). Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`.
*
* @return doneAt
*/
public OffsetDateTime getDoneAt() {
return doneAt;
}
/**
* Indicates whether the error occurred during the query execution.
*
* @return error
*/
public SmsError getError() {
return error;
}
/**
* Sender ID that can be alphanumeric or numeric.
*
* @return from
*/
public String getFrom() {
return from;
}
/**
* Mobile country and network codes.
*
* @return mccMnc
*/
public String getMccMnc() {
return mccMnc;
}
/**
* Message ID.
*
* @return messageId
*/
public String getMessageId() {
return messageId;
}
/**
* Sent SMS price.
*
* @return price
*/
public SmsPrice getPrice() {
return price;
}
/**
* Tells when the SMS was sent. Has the following format:
* `yyyy-MM-dd'T'HH:mm:ss.SSSZ`.
*
* @return sentAt
*/
public OffsetDateTime getSentAt() {
return sentAt;
}
/**
* The number of parts the sent SMS was split into.
*
* @return smsCount
*/
public Integer getSmsCount() {
return smsCount;
}
/**
* Indicates whether the message is successfully sent, not sent, delivered, not delivered, waiting
* for delivery or any other possible status.
*
* @return status
*/
public SmsStatus getStatus() {
return status;
}
/**
* Destination address.
*
* @return to
*/
public String getTo() {
return to;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
SmsReport smsReport = (SmsReport) o;
return Objects.equals(this.bulkId, smsReport.bulkId)
&& Objects.equals(this.callbackData, smsReport.callbackData)
&& Objects.equals(this.doneAt, smsReport.doneAt)
&& Objects.equals(this.error, smsReport.error)
&& Objects.equals(this.from, smsReport.from)
&& Objects.equals(this.mccMnc, smsReport.mccMnc)
&& Objects.equals(this.messageId, smsReport.messageId)
&& Objects.equals(this.price, smsReport.price)
&& Objects.equals(this.sentAt, smsReport.sentAt)
&& Objects.equals(this.smsCount, smsReport.smsCount)
&& Objects.equals(this.status, smsReport.status)
&& Objects.equals(this.to, smsReport.to);
}
@Override
public int hashCode() {
return Objects.hash(
bulkId,
callbackData,
doneAt,
error,
from,
mccMnc,
messageId,
price,
sentAt,
smsCount,
status,
to);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class SmsReport {\n");
sb.append(" bulkId: ").append(toIndentedString(bulkId)).append("\n");
sb.append(" callbackData: ").append(toIndentedString(callbackData)).append("\n");
sb.append(" doneAt: ").append(toIndentedString(doneAt)).append("\n");
sb.append(" error: ").append(toIndentedString(error)).append("\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" mccMnc: ").append(toIndentedString(mccMnc)).append("\n");
sb.append(" messageId: ").append(toIndentedString(messageId)).append("\n");
sb.append(" price: ").append(toIndentedString(price)).append("\n");
sb.append(" sentAt: ").append(toIndentedString(sentAt)).append("\n");
sb.append(" smsCount: ").append(toIndentedString(smsCount)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy