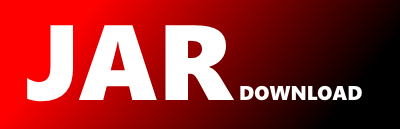
com.infobip.model.TfaStartAuthenticationRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infobip-api-java-client Show documentation
Show all versions of infobip-api-java-client Show documentation
API client in Java for Infobip's API (http://dev.infobip.com/).
/*
* Infobip Client API Libraries OpenAPI Specification
* OpenAPI specification containing public endpoints supported in client API libraries.
*
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.infobip.model;
import com.google.gson.annotations.SerializedName;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/** TfaStartAuthenticationRequest */
public class TfaStartAuthenticationRequest {
public static final String SERIALIZED_NAME_APPLICATION_ID = "applicationId";
@SerializedName(SERIALIZED_NAME_APPLICATION_ID)
private String applicationId;
public static final String SERIALIZED_NAME_FROM = "from";
@SerializedName(SERIALIZED_NAME_FROM)
private String from;
public static final String SERIALIZED_NAME_MESSAGE_ID = "messageId";
@SerializedName(SERIALIZED_NAME_MESSAGE_ID)
private String messageId;
public static final String SERIALIZED_NAME_PLACEHOLDERS = "placeholders";
@SerializedName(SERIALIZED_NAME_PLACEHOLDERS)
private Map placeholders = null;
public static final String SERIALIZED_NAME_TO = "to";
@SerializedName(SERIALIZED_NAME_TO)
private String to;
public TfaStartAuthenticationRequest applicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
/**
* 2FA application ID.
*
* @return applicationId
*/
public String getApplicationId() {
return applicationId;
}
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
public TfaStartAuthenticationRequest from(String from) {
this.from = from;
return this;
}
/**
* Use this parameter if you wish to override the sender ID from the
* [created](#channels/sms/create-2fa-message-template) message template parameter
* `senderId`.
*
* @return from
*/
public String getFrom() {
return from;
}
public void setFrom(String from) {
this.from = from;
}
public TfaStartAuthenticationRequest messageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Message template ID that will be sent to phone number.
*
* @return messageId
*/
public String getMessageId() {
return messageId;
}
public void setMessageId(String messageId) {
this.messageId = messageId;
}
public TfaStartAuthenticationRequest placeholders(Map placeholders) {
this.placeholders = placeholders;
return this;
}
public TfaStartAuthenticationRequest putPlaceholdersItem(String key, String placeholdersItem) {
if (this.placeholders == null) {
this.placeholders = new HashMap<>();
}
this.placeholders.put(key, placeholdersItem);
return this;
}
/**
* Key value pairs that will be replaced during message sending. Placeholder keys should NOT
* contain curly brackets and should NOT contain a `pin` placeholder. Valid example:
* `\"placeholders\":{\"firstName\":\"John\"}`
*
* @return placeholders
*/
public Map getPlaceholders() {
return placeholders;
}
public void setPlaceholders(Map placeholders) {
this.placeholders = placeholders;
}
public TfaStartAuthenticationRequest to(String to) {
this.to = to;
return this;
}
/**
* Phone number to which the 2FA message will be sent. Example: 41793026727.
*
* @return to
*/
public String getTo() {
return to;
}
public void setTo(String to) {
this.to = to;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TfaStartAuthenticationRequest tfaStartAuthenticationRequest = (TfaStartAuthenticationRequest) o;
return Objects.equals(this.applicationId, tfaStartAuthenticationRequest.applicationId)
&& Objects.equals(this.from, tfaStartAuthenticationRequest.from)
&& Objects.equals(this.messageId, tfaStartAuthenticationRequest.messageId)
&& Objects.equals(this.placeholders, tfaStartAuthenticationRequest.placeholders)
&& Objects.equals(this.to, tfaStartAuthenticationRequest.to);
}
@Override
public int hashCode() {
return Objects.hash(applicationId, from, messageId, placeholders, to);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TfaStartAuthenticationRequest {\n");
sb.append(" applicationId: ").append(toIndentedString(applicationId)).append("\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" messageId: ").append(toIndentedString(messageId)).append("\n");
sb.append(" placeholders: ").append(toIndentedString(placeholders)).append("\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy