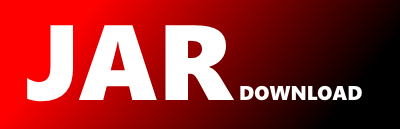
com.infobip.model.CallsClickToCallMessage Maven / Gradle / Ivy
/*
* This class is auto generated from the Infobip OpenAPI specification
* through the OpenAPI Specification Client API libraries (Re)Generator (OSCAR),
* powered by the OpenAPI Generator (https://openapi-generator.tech).
*
* Do not edit manually. To learn how to raise an issue, see the CONTRIBUTING guide
* or contact us @ [email protected].
*/
package com.infobip.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Objects;
/**
* Array of click to call messages to be sent.
*/
public class CallsClickToCallMessage {
private Boolean anonymization;
private String audioFileUrl;
private CallsDeliveryTimeWindow deliveryTimeWindow;
private String destinationA;
private String destinationB;
private String from;
private String fromB;
private String language;
private String machineDetection;
private Integer maxDuration;
private String messageId;
private String notifyContentType;
private Integer notifyContentVersion;
private String notifyUrl;
private Boolean record;
private CallsRetry retry;
private String text;
private CallsVoice voice;
private Integer warningTime;
/**
* Sets anonymization.
*
* Field description:
* If set, same numeric sender (defined in _from_) is used for both calls (towards _destinationA_ and _destinationB_). If not set, _destinationA_ will be shown to _destinationB_. If _fromB_ is set up, _anonymization_ will not be applied; _from_ will be shown to _destinationA_ and _fromB_ will be shown to _destinationB_. Default value is `false`.
*
* @param anonymization
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage anonymization(Boolean anonymization) {
this.anonymization = anonymization;
return this;
}
/**
* Returns anonymization.
*
* Field description:
* If set, same numeric sender (defined in _from_) is used for both calls (towards _destinationA_ and _destinationB_). If not set, _destinationA_ will be shown to _destinationB_. If _fromB_ is set up, _anonymization_ will not be applied; _from_ will be shown to _destinationA_ and _fromB_ will be shown to _destinationB_. Default value is `false`.
*
* @return anonymization
*/
@JsonProperty("anonymization")
public Boolean getAnonymization() {
return anonymization;
}
/**
* Sets anonymization.
*
* Field description:
* If set, same numeric sender (defined in _from_) is used for both calls (towards _destinationA_ and _destinationB_). If not set, _destinationA_ will be shown to _destinationB_. If _fromB_ is set up, _anonymization_ will not be applied; _from_ will be shown to _destinationA_ and _fromB_ will be shown to _destinationB_. Default value is `false`.
*
* @param anonymization
*/
@JsonProperty("anonymization")
public void setAnonymization(Boolean anonymization) {
this.anonymization = anonymization;
}
/**
* Sets audioFileUrl.
*
* Field description:
* An audio file can be delivered as a voice message to the recipients. An audio file must be uploaded online, so that the existing URL can be available for file download. Size of the audio file must be below 4 MB. Supported formats of the provided file are aac, aiff, m4a, mp2, mp3, mp4 (audio only), ogg, wav and wma. Our platform needs to have permission to make GET and HEAD HTTP requests on the provided URL. Standard http ports (like 80, 8080, etc.) are advised.
*
* @param audioFileUrl
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage audioFileUrl(String audioFileUrl) {
this.audioFileUrl = audioFileUrl;
return this;
}
/**
* Returns audioFileUrl.
*
* Field description:
* An audio file can be delivered as a voice message to the recipients. An audio file must be uploaded online, so that the existing URL can be available for file download. Size of the audio file must be below 4 MB. Supported formats of the provided file are aac, aiff, m4a, mp2, mp3, mp4 (audio only), ogg, wav and wma. Our platform needs to have permission to make GET and HEAD HTTP requests on the provided URL. Standard http ports (like 80, 8080, etc.) are advised.
*
* @return audioFileUrl
*/
@JsonProperty("audioFileUrl")
public String getAudioFileUrl() {
return audioFileUrl;
}
/**
* Sets audioFileUrl.
*
* Field description:
* An audio file can be delivered as a voice message to the recipients. An audio file must be uploaded online, so that the existing URL can be available for file download. Size of the audio file must be below 4 MB. Supported formats of the provided file are aac, aiff, m4a, mp2, mp3, mp4 (audio only), ogg, wav and wma. Our platform needs to have permission to make GET and HEAD HTTP requests on the provided URL. Standard http ports (like 80, 8080, etc.) are advised.
*
* @param audioFileUrl
*/
@JsonProperty("audioFileUrl")
public void setAudioFileUrl(String audioFileUrl) {
this.audioFileUrl = audioFileUrl;
}
/**
* Sets deliveryTimeWindow.
*
* @param deliveryTimeWindow
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage deliveryTimeWindow(CallsDeliveryTimeWindow deliveryTimeWindow) {
this.deliveryTimeWindow = deliveryTimeWindow;
return this;
}
/**
* Returns deliveryTimeWindow.
*
* @return deliveryTimeWindow
*/
@JsonProperty("deliveryTimeWindow")
public CallsDeliveryTimeWindow getDeliveryTimeWindow() {
return deliveryTimeWindow;
}
/**
* Sets deliveryTimeWindow.
*
* @param deliveryTimeWindow
*/
@JsonProperty("deliveryTimeWindow")
public void setDeliveryTimeWindow(CallsDeliveryTimeWindow deliveryTimeWindow) {
this.deliveryTimeWindow = deliveryTimeWindow;
}
/**
* Sets destinationA.
*
* Field description:
* Original destination address. Address must be in the international format (Example: `41793026727`).
*
* The field is required.
*
* @param destinationA
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage destinationA(String destinationA) {
this.destinationA = destinationA;
return this;
}
/**
* Returns destinationA.
*
* Field description:
* Original destination address. Address must be in the international format (Example: `41793026727`).
*
* The field is required.
*
* @return destinationA
*/
@JsonProperty("destinationA")
public String getDestinationA() {
return destinationA;
}
/**
* Sets destinationA.
*
* Field description:
* Original destination address. Address must be in the international format (Example: `41793026727`).
*
* The field is required.
*
* @param destinationA
*/
@JsonProperty("destinationA")
public void setDestinationA(String destinationA) {
this.destinationA = destinationA;
}
/**
* Sets destinationB.
*
* Field description:
* Destination address to which the call will be transferred after successful answering. Address must be in the international format (Example: `41793026727`).
*
* The field is required.
*
* @param destinationB
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage destinationB(String destinationB) {
this.destinationB = destinationB;
return this;
}
/**
* Returns destinationB.
*
* Field description:
* Destination address to which the call will be transferred after successful answering. Address must be in the international format (Example: `41793026727`).
*
* The field is required.
*
* @return destinationB
*/
@JsonProperty("destinationB")
public String getDestinationB() {
return destinationB;
}
/**
* Sets destinationB.
*
* Field description:
* Destination address to which the call will be transferred after successful answering. Address must be in the international format (Example: `41793026727`).
*
* The field is required.
*
* @param destinationB
*/
@JsonProperty("destinationB")
public void setDestinationB(String destinationB) {
this.destinationB = destinationB;
}
/**
* Sets from.
*
* Field description:
* Numeric sender ID in E.164 format. Will be shown to _destinationA_.
*
* The field is required.
*
* @param from
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage from(String from) {
this.from = from;
return this;
}
/**
* Returns from.
*
* Field description:
* Numeric sender ID in E.164 format. Will be shown to _destinationA_.
*
* The field is required.
*
* @return from
*/
@JsonProperty("from")
public String getFrom() {
return from;
}
/**
* Sets from.
*
* Field description:
* Numeric sender ID in E.164 format. Will be shown to _destinationA_.
*
* The field is required.
*
* @param from
*/
@JsonProperty("from")
public void setFrom(String from) {
this.from = from;
}
/**
* Sets fromB.
*
* Field description:
* Numeric sender ID in E.164 format. If set, it will be shown to _destinationB_. If not set, _from_ or _destinationA_ will be shown to _destinationB_, depending on the value of _anonymization_.
*
* @param fromB
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage fromB(String fromB) {
this.fromB = fromB;
return this;
}
/**
* Returns fromB.
*
* Field description:
* Numeric sender ID in E.164 format. If set, it will be shown to _destinationB_. If not set, _from_ or _destinationA_ will be shown to _destinationB_, depending on the value of _anonymization_.
*
* @return fromB
*/
@JsonProperty("fromB")
public String getFromB() {
return fromB;
}
/**
* Sets fromB.
*
* Field description:
* Numeric sender ID in E.164 format. If set, it will be shown to _destinationB_. If not set, _from_ or _destinationA_ will be shown to _destinationB_, depending on the value of _anonymization_.
*
* @param fromB
*/
@JsonProperty("fromB")
public void setFromB(String fromB) {
this.fromB = fromB;
}
/**
* Sets language.
*
* Field description:
* If the message is in text format, language in which the message is written must be defined for correct pronunciation. In the Languages section, you can find the list of languages that we support. If not set, default language is `English [en].`
*
* @param language
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage language(String language) {
this.language = language;
return this;
}
/**
* Returns language.
*
* Field description:
* If the message is in text format, language in which the message is written must be defined for correct pronunciation. In the Languages section, you can find the list of languages that we support. If not set, default language is `English [en].`
*
* @return language
*/
@JsonProperty("language")
public String getLanguage() {
return language;
}
/**
* Sets language.
*
* Field description:
* If the message is in text format, language in which the message is written must be defined for correct pronunciation. In the Languages section, you can find the list of languages that we support. If not set, default language is `English [en].`
*
* @param language
*/
@JsonProperty("language")
public void setLanguage(String language) {
this.language = language;
}
/**
* Sets machineDetection.
*
* Field description:
* Used for enabling detection of answering machine after the call has been answered on `destinationA`. It can be set to `hangup` which means if a machine is detected, the call is hung up. If `machineDetection` is used, there is a minimum of 4 seconds detection time, which can result in delay of playing the message. Answering machine detection is additionally charged. For more information please contact your account manager.
*
* @param machineDetection
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage machineDetection(String machineDetection) {
this.machineDetection = machineDetection;
return this;
}
/**
* Returns machineDetection.
*
* Field description:
* Used for enabling detection of answering machine after the call has been answered on `destinationA`. It can be set to `hangup` which means if a machine is detected, the call is hung up. If `machineDetection` is used, there is a minimum of 4 seconds detection time, which can result in delay of playing the message. Answering machine detection is additionally charged. For more information please contact your account manager.
*
* @return machineDetection
*/
@JsonProperty("machineDetection")
public String getMachineDetection() {
return machineDetection;
}
/**
* Sets machineDetection.
*
* Field description:
* Used for enabling detection of answering machine after the call has been answered on `destinationA`. It can be set to `hangup` which means if a machine is detected, the call is hung up. If `machineDetection` is used, there is a minimum of 4 seconds detection time, which can result in delay of playing the message. Answering machine detection is additionally charged. For more information please contact your account manager.
*
* @param machineDetection
*/
@JsonProperty("machineDetection")
public void setMachineDetection(String machineDetection) {
this.machineDetection = machineDetection;
}
/**
* Sets maxDuration.
*
* Field description:
* Maximum duration of transferred call in seconds. If set, when _maxDuration_ is reached call will be terminated.
*
* @param maxDuration
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage maxDuration(Integer maxDuration) {
this.maxDuration = maxDuration;
return this;
}
/**
* Returns maxDuration.
*
* Field description:
* Maximum duration of transferred call in seconds. If set, when _maxDuration_ is reached call will be terminated.
*
* @return maxDuration
*/
@JsonProperty("maxDuration")
public Integer getMaxDuration() {
return maxDuration;
}
/**
* Sets maxDuration.
*
* Field description:
* Maximum duration of transferred call in seconds. If set, when _maxDuration_ is reached call will be terminated.
*
* @param maxDuration
*/
@JsonProperty("maxDuration")
public void setMaxDuration(Integer maxDuration) {
this.maxDuration = maxDuration;
}
/**
* Sets messageId.
*
* Field description:
* The ID that uniquely identifies the message sent. It can be defined by you or Infobip will generate unique message ID for this specific call. The field is not mandatory.
*
* @param messageId
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage messageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Returns messageId.
*
* Field description:
* The ID that uniquely identifies the message sent. It can be defined by you or Infobip will generate unique message ID for this specific call. The field is not mandatory.
*
* @return messageId
*/
@JsonProperty("messageId")
public String getMessageId() {
return messageId;
}
/**
* Sets messageId.
*
* Field description:
* The ID that uniquely identifies the message sent. It can be defined by you or Infobip will generate unique message ID for this specific call. The field is not mandatory.
*
* @param messageId
*/
@JsonProperty("messageId")
public void setMessageId(String messageId) {
this.messageId = messageId;
}
/**
* Sets notifyContentType.
*
* Field description:
* Preferred Delivery report content type. Can be `application/json` or `application/xml`.
*
* @param notifyContentType
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage notifyContentType(String notifyContentType) {
this.notifyContentType = notifyContentType;
return this;
}
/**
* Returns notifyContentType.
*
* Field description:
* Preferred Delivery report content type. Can be `application/json` or `application/xml`.
*
* @return notifyContentType
*/
@JsonProperty("notifyContentType")
public String getNotifyContentType() {
return notifyContentType;
}
/**
* Sets notifyContentType.
*
* Field description:
* Preferred Delivery report content type. Can be `application/json` or `application/xml`.
*
* @param notifyContentType
*/
@JsonProperty("notifyContentType")
public void setNotifyContentType(String notifyContentType) {
this.notifyContentType = notifyContentType;
}
/**
* Sets notifyContentVersion.
*
* Field description:
* Specifies the version of the report model to be sent. Can be `1` ([deprecated version 1](#programmable-communications/voice/receive-voice-delivery-reports-deprecated)) or `2` ([current version 2](#programmable-communications/voice/receive-voice-delivery-reports)). The default is version 2.
*
* @param notifyContentVersion
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage notifyContentVersion(Integer notifyContentVersion) {
this.notifyContentVersion = notifyContentVersion;
return this;
}
/**
* Returns notifyContentVersion.
*
* Field description:
* Specifies the version of the report model to be sent. Can be `1` ([deprecated version 1](#programmable-communications/voice/receive-voice-delivery-reports-deprecated)) or `2` ([current version 2](#programmable-communications/voice/receive-voice-delivery-reports)). The default is version 2.
*
* @return notifyContentVersion
*/
@JsonProperty("notifyContentVersion")
public Integer getNotifyContentVersion() {
return notifyContentVersion;
}
/**
* Sets notifyContentVersion.
*
* Field description:
* Specifies the version of the report model to be sent. Can be `1` ([deprecated version 1](#programmable-communications/voice/receive-voice-delivery-reports-deprecated)) or `2` ([current version 2](#programmable-communications/voice/receive-voice-delivery-reports)). The default is version 2.
*
* @param notifyContentVersion
*/
@JsonProperty("notifyContentVersion")
public void setNotifyContentVersion(Integer notifyContentVersion) {
this.notifyContentVersion = notifyContentVersion;
}
/**
* Sets notifyUrl.
*
* Field description:
* The URL on your callback server on which the Delivery report will be sent.
*
* @param notifyUrl
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage notifyUrl(String notifyUrl) {
this.notifyUrl = notifyUrl;
return this;
}
/**
* Returns notifyUrl.
*
* Field description:
* The URL on your callback server on which the Delivery report will be sent.
*
* @return notifyUrl
*/
@JsonProperty("notifyUrl")
public String getNotifyUrl() {
return notifyUrl;
}
/**
* Sets notifyUrl.
*
* Field description:
* The URL on your callback server on which the Delivery report will be sent.
*
* @param notifyUrl
*/
@JsonProperty("notifyUrl")
public void setNotifyUrl(String notifyUrl) {
this.notifyUrl = notifyUrl;
}
/**
* Sets record.
*
* Field description:
* [Early access: Contact your account manager to enable the usage] Record the call and expose it to client as URL inside the delivery report. Can be `true` or `false`.
*
* @param record
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage record(Boolean record) {
this.record = record;
return this;
}
/**
* Returns record.
*
* Field description:
* [Early access: Contact your account manager to enable the usage] Record the call and expose it to client as URL inside the delivery report. Can be `true` or `false`.
*
* @return record
*/
@JsonProperty("record")
public Boolean getRecord() {
return record;
}
/**
* Sets record.
*
* Field description:
* [Early access: Contact your account manager to enable the usage] Record the call and expose it to client as URL inside the delivery report. Can be `true` or `false`.
*
* @param record
*/
@JsonProperty("record")
public void setRecord(Boolean record) {
this.record = record;
}
/**
* Sets retry.
*
* @param retry
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage retry(CallsRetry retry) {
this.retry = retry;
return this;
}
/**
* Returns retry.
*
* @return retry
*/
@JsonProperty("retry")
public CallsRetry getRetry() {
return retry;
}
/**
* Sets retry.
*
* @param retry
*/
@JsonProperty("retry")
public void setRetry(CallsRetry retry) {
this.retry = retry;
}
/**
* Sets text.
*
* Field description:
* Text of the message that will be played before call transfer.
*
* @param text
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage text(String text) {
this.text = text;
return this;
}
/**
* Returns text.
*
* Field description:
* Text of the message that will be played before call transfer.
*
* @return text
*/
@JsonProperty("text")
public String getText() {
return text;
}
/**
* Sets text.
*
* Field description:
* Text of the message that will be played before call transfer.
*
* @param text
*/
@JsonProperty("text")
public void setText(String text) {
this.text = text;
}
/**
* Sets voice.
*
* @param voice
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage voice(CallsVoice voice) {
this.voice = voice;
return this;
}
/**
* Returns voice.
*
* @return voice
*/
@JsonProperty("voice")
public CallsVoice getVoice() {
return voice;
}
/**
* Sets voice.
*
* @param voice
*/
@JsonProperty("voice")
public void setVoice(CallsVoice voice) {
this.voice = voice;
}
/**
* Sets warningTime.
*
* Field description:
* Time before the end of the transferred call, in seconds, when warning beep sound will be played. For example, if _maxDuration_ is set to `60` and warningTime is set to `5`, this means that warning beep will be played on `55.` second of the transferred call, or `5` seconds before it's end.
*
* @param warningTime
* @return This {@link CallsClickToCallMessage instance}.
*/
public CallsClickToCallMessage warningTime(Integer warningTime) {
this.warningTime = warningTime;
return this;
}
/**
* Returns warningTime.
*
* Field description:
* Time before the end of the transferred call, in seconds, when warning beep sound will be played. For example, if _maxDuration_ is set to `60` and warningTime is set to `5`, this means that warning beep will be played on `55.` second of the transferred call, or `5` seconds before it's end.
*
* @return warningTime
*/
@JsonProperty("warningTime")
public Integer getWarningTime() {
return warningTime;
}
/**
* Sets warningTime.
*
* Field description:
* Time before the end of the transferred call, in seconds, when warning beep sound will be played. For example, if _maxDuration_ is set to `60` and warningTime is set to `5`, this means that warning beep will be played on `55.` second of the transferred call, or `5` seconds before it's end.
*
* @param warningTime
*/
@JsonProperty("warningTime")
public void setWarningTime(Integer warningTime) {
this.warningTime = warningTime;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CallsClickToCallMessage callsClickToCallMessage = (CallsClickToCallMessage) o;
return Objects.equals(this.anonymization, callsClickToCallMessage.anonymization)
&& Objects.equals(this.audioFileUrl, callsClickToCallMessage.audioFileUrl)
&& Objects.equals(this.deliveryTimeWindow, callsClickToCallMessage.deliveryTimeWindow)
&& Objects.equals(this.destinationA, callsClickToCallMessage.destinationA)
&& Objects.equals(this.destinationB, callsClickToCallMessage.destinationB)
&& Objects.equals(this.from, callsClickToCallMessage.from)
&& Objects.equals(this.fromB, callsClickToCallMessage.fromB)
&& Objects.equals(this.language, callsClickToCallMessage.language)
&& Objects.equals(this.machineDetection, callsClickToCallMessage.machineDetection)
&& Objects.equals(this.maxDuration, callsClickToCallMessage.maxDuration)
&& Objects.equals(this.messageId, callsClickToCallMessage.messageId)
&& Objects.equals(this.notifyContentType, callsClickToCallMessage.notifyContentType)
&& Objects.equals(this.notifyContentVersion, callsClickToCallMessage.notifyContentVersion)
&& Objects.equals(this.notifyUrl, callsClickToCallMessage.notifyUrl)
&& Objects.equals(this.record, callsClickToCallMessage.record)
&& Objects.equals(this.retry, callsClickToCallMessage.retry)
&& Objects.equals(this.text, callsClickToCallMessage.text)
&& Objects.equals(this.voice, callsClickToCallMessage.voice)
&& Objects.equals(this.warningTime, callsClickToCallMessage.warningTime);
}
@Override
public int hashCode() {
return Objects.hash(
anonymization,
audioFileUrl,
deliveryTimeWindow,
destinationA,
destinationB,
from,
fromB,
language,
machineDetection,
maxDuration,
messageId,
notifyContentType,
notifyContentVersion,
notifyUrl,
record,
retry,
text,
voice,
warningTime);
}
@Override
public String toString() {
String newLine = System.lineSeparator();
return new StringBuilder()
.append("class CallsClickToCallMessage {")
.append(newLine)
.append(" anonymization: ")
.append(toIndentedString(anonymization))
.append(newLine)
.append(" audioFileUrl: ")
.append(toIndentedString(audioFileUrl))
.append(newLine)
.append(" deliveryTimeWindow: ")
.append(toIndentedString(deliveryTimeWindow))
.append(newLine)
.append(" destinationA: ")
.append(toIndentedString(destinationA))
.append(newLine)
.append(" destinationB: ")
.append(toIndentedString(destinationB))
.append(newLine)
.append(" from: ")
.append(toIndentedString(from))
.append(newLine)
.append(" fromB: ")
.append(toIndentedString(fromB))
.append(newLine)
.append(" language: ")
.append(toIndentedString(language))
.append(newLine)
.append(" machineDetection: ")
.append(toIndentedString(machineDetection))
.append(newLine)
.append(" maxDuration: ")
.append(toIndentedString(maxDuration))
.append(newLine)
.append(" messageId: ")
.append(toIndentedString(messageId))
.append(newLine)
.append(" notifyContentType: ")
.append(toIndentedString(notifyContentType))
.append(newLine)
.append(" notifyContentVersion: ")
.append(toIndentedString(notifyContentVersion))
.append(newLine)
.append(" notifyUrl: ")
.append(toIndentedString(notifyUrl))
.append(newLine)
.append(" record: ")
.append(toIndentedString(record))
.append(newLine)
.append(" retry: ")
.append(toIndentedString(retry))
.append(newLine)
.append(" text: ")
.append(toIndentedString(text))
.append(newLine)
.append(" voice: ")
.append(toIndentedString(voice))
.append(newLine)
.append(" warningTime: ")
.append(toIndentedString(warningTime))
.append(newLine)
.append("}")
.toString();
}
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
String lineSeparator = System.lineSeparator();
String lineSeparatorFollowedByIndentation = lineSeparator + " ";
return o.toString().replace(lineSeparator, lineSeparatorFollowedByIndentation);
}
}