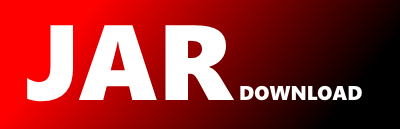
com.infobip.model.CallsSendingSpeed Maven / Gradle / Ivy
/*
* This class is auto generated from the Infobip OpenAPI specification
* through the OpenAPI Specification Client API libraries (Re)Generator (OSCAR),
* powered by the OpenAPI Generator (https://openapi-generator.tech).
*
* Do not edit manually. To learn how to raise an issue, see the CONTRIBUTING guide
* or contact us @ [email protected].
*/
package com.infobip.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Objects;
/**
* Sending rate defined in number of messages sent per second, minute, hour or day. First message will be sent immediately (or at _sendAt_ time if scheduling is used) and subsequent messages will be sent respecting defined speed. For example, if _sendingSpeed_ is defined as 10 messages per hour, messages will be sent every 6 minutes. If this parameter is defined, _validityPeriod_ is ignored.
*/
public class CallsSendingSpeed {
private Integer speed;
private String timeUnit;
/**
* Sets speed.
*
* Field description:
* Defines the number of messages that will be sent per specified time unit.
*
* @param speed
* @return This {@link CallsSendingSpeed instance}.
*/
public CallsSendingSpeed speed(Integer speed) {
this.speed = speed;
return this;
}
/**
* Returns speed.
*
* Field description:
* Defines the number of messages that will be sent per specified time unit.
*
* @return speed
*/
@JsonProperty("speed")
public Integer getSpeed() {
return speed;
}
/**
* Sets speed.
*
* Field description:
* Defines the number of messages that will be sent per specified time unit.
*
* @param speed
*/
@JsonProperty("speed")
public void setSpeed(Integer speed) {
this.speed = speed;
}
/**
* Sets timeUnit.
*
* Field description:
* Defines time unit used for calculating sending speed. Possible values: `second`, `minute`, `hour` and `day`.
*
* @param timeUnit
* @return This {@link CallsSendingSpeed instance}.
*/
public CallsSendingSpeed timeUnit(String timeUnit) {
this.timeUnit = timeUnit;
return this;
}
/**
* Returns timeUnit.
*
* Field description:
* Defines time unit used for calculating sending speed. Possible values: `second`, `minute`, `hour` and `day`.
*
* @return timeUnit
*/
@JsonProperty("timeUnit")
public String getTimeUnit() {
return timeUnit;
}
/**
* Sets timeUnit.
*
* Field description:
* Defines time unit used for calculating sending speed. Possible values: `second`, `minute`, `hour` and `day`.
*
* @param timeUnit
*/
@JsonProperty("timeUnit")
public void setTimeUnit(String timeUnit) {
this.timeUnit = timeUnit;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CallsSendingSpeed callsSendingSpeed = (CallsSendingSpeed) o;
return Objects.equals(this.speed, callsSendingSpeed.speed)
&& Objects.equals(this.timeUnit, callsSendingSpeed.timeUnit);
}
@Override
public int hashCode() {
return Objects.hash(speed, timeUnit);
}
@Override
public String toString() {
String newLine = System.lineSeparator();
return new StringBuilder()
.append("class CallsSendingSpeed {")
.append(newLine)
.append(" speed: ")
.append(toIndentedString(speed))
.append(newLine)
.append(" timeUnit: ")
.append(toIndentedString(timeUnit))
.append(newLine)
.append("}")
.toString();
}
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
String lineSeparator = System.lineSeparator();
String lineSeparatorFollowedByIndentation = lineSeparator + " ";
return o.toString().replace(lineSeparator, lineSeparatorFollowedByIndentation);
}
}