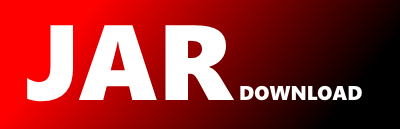
com.infobip.model.CallsStaticSipTrunkRequest Maven / Gradle / Ivy
/*
* This class is auto generated from the Infobip OpenAPI specification
* through the OpenAPI Specification Client API libraries (Re)Generator (OSCAR),
* powered by the OpenAPI Generator (https://openapi-generator.tech).
*
* Do not edit manually. To learn how to raise an issue, see the CONTRIBUTING guide
* or contact us @ [email protected].
*/
package com.infobip.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* Represents CallsStaticSipTrunkRequest model.
*/
public class CallsStaticSipTrunkRequest extends CallsSipTrunkRequest {
private List sourceHosts = null;
private List destinationHosts = null;
private CallsSelectionStrategy strategy;
private CallsSipOptions sipOptions;
/**
* Constructs a new {@link CallsStaticSipTrunkRequest} instance.
*/
public CallsStaticSipTrunkRequest() {
super("STATIC");
}
/**
* Sets sourceHosts.
*
* Field description:
* List of SIP trunk source hosts. If empty, destination host list must not be empty. Source hosts can be send in 2 formats: IP address without port or domain without port.
*
* @param sourceHosts
* @return This {@link CallsStaticSipTrunkRequest instance}.
*/
public CallsStaticSipTrunkRequest sourceHosts(List sourceHosts) {
this.sourceHosts = sourceHosts;
return this;
}
/**
* Adds and item into sourceHosts.
*
* Field description:
* List of SIP trunk source hosts. If empty, destination host list must not be empty. Source hosts can be send in 2 formats: IP address without port or domain without port.
*
* @param sourceHostsItem The item to be added to the list.
* @return This {@link CallsStaticSipTrunkRequest instance}.
*/
public CallsStaticSipTrunkRequest addSourceHostsItem(String sourceHostsItem) {
if (this.sourceHosts == null) {
this.sourceHosts = new ArrayList<>();
}
this.sourceHosts.add(sourceHostsItem);
return this;
}
/**
* Returns sourceHosts.
*
* Field description:
* List of SIP trunk source hosts. If empty, destination host list must not be empty. Source hosts can be send in 2 formats: IP address without port or domain without port.
*
* @return sourceHosts
*/
@JsonProperty("sourceHosts")
public List getSourceHosts() {
return sourceHosts;
}
/**
* Sets sourceHosts.
*
* Field description:
* List of SIP trunk source hosts. If empty, destination host list must not be empty. Source hosts can be send in 2 formats: IP address without port or domain without port.
*
* @param sourceHosts
*/
@JsonProperty("sourceHosts")
public void setSourceHosts(List sourceHosts) {
this.sourceHosts = sourceHosts;
}
/**
* Sets destinationHosts.
*
* Field description:
* List of SIP trunk destination hosts. If empty, source host list must not be empty. Destination hosts can be sent in 3 formats: IP address with port, domain name with port or domain name without port. The port must fall in the range 1025-65535 or be 0 for SRV lookup.
*
* @param destinationHosts
* @return This {@link CallsStaticSipTrunkRequest instance}.
*/
public CallsStaticSipTrunkRequest destinationHosts(List destinationHosts) {
this.destinationHosts = destinationHosts;
return this;
}
/**
* Adds and item into destinationHosts.
*
* Field description:
* List of SIP trunk destination hosts. If empty, source host list must not be empty. Destination hosts can be sent in 3 formats: IP address with port, domain name with port or domain name without port. The port must fall in the range 1025-65535 or be 0 for SRV lookup.
*
* @param destinationHostsItem The item to be added to the list.
* @return This {@link CallsStaticSipTrunkRequest instance}.
*/
public CallsStaticSipTrunkRequest addDestinationHostsItem(String destinationHostsItem) {
if (this.destinationHosts == null) {
this.destinationHosts = new ArrayList<>();
}
this.destinationHosts.add(destinationHostsItem);
return this;
}
/**
* Returns destinationHosts.
*
* Field description:
* List of SIP trunk destination hosts. If empty, source host list must not be empty. Destination hosts can be sent in 3 formats: IP address with port, domain name with port or domain name without port. The port must fall in the range 1025-65535 or be 0 for SRV lookup.
*
* @return destinationHosts
*/
@JsonProperty("destinationHosts")
public List getDestinationHosts() {
return destinationHosts;
}
/**
* Sets destinationHosts.
*
* Field description:
* List of SIP trunk destination hosts. If empty, source host list must not be empty. Destination hosts can be sent in 3 formats: IP address with port, domain name with port or domain name without port. The port must fall in the range 1025-65535 or be 0 for SRV lookup.
*
* @param destinationHosts
*/
@JsonProperty("destinationHosts")
public void setDestinationHosts(List destinationHosts) {
this.destinationHosts = destinationHosts;
}
/**
* Sets strategy.
*
* @param strategy
* @return This {@link CallsStaticSipTrunkRequest instance}.
*/
public CallsStaticSipTrunkRequest strategy(CallsSelectionStrategy strategy) {
this.strategy = strategy;
return this;
}
/**
* Returns strategy.
*
* @return strategy
*/
@JsonProperty("strategy")
public CallsSelectionStrategy getStrategy() {
return strategy;
}
/**
* Sets strategy.
*
* @param strategy
*/
@JsonProperty("strategy")
public void setStrategy(CallsSelectionStrategy strategy) {
this.strategy = strategy;
}
/**
* Sets sipOptions.
*
* @param sipOptions
* @return This {@link CallsStaticSipTrunkRequest instance}.
*/
public CallsStaticSipTrunkRequest sipOptions(CallsSipOptions sipOptions) {
this.sipOptions = sipOptions;
return this;
}
/**
* Returns sipOptions.
*
* @return sipOptions
*/
@JsonProperty("sipOptions")
public CallsSipOptions getSipOptions() {
return sipOptions;
}
/**
* Sets sipOptions.
*
* @param sipOptions
*/
@JsonProperty("sipOptions")
public void setSipOptions(CallsSipOptions sipOptions) {
this.sipOptions = sipOptions;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CallsStaticSipTrunkRequest callsStaticSipTrunkRequest = (CallsStaticSipTrunkRequest) o;
return Objects.equals(this.sourceHosts, callsStaticSipTrunkRequest.sourceHosts)
&& Objects.equals(this.destinationHosts, callsStaticSipTrunkRequest.destinationHosts)
&& Objects.equals(this.strategy, callsStaticSipTrunkRequest.strategy)
&& Objects.equals(this.sipOptions, callsStaticSipTrunkRequest.sipOptions)
&& super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(sourceHosts, destinationHosts, strategy, sipOptions, super.hashCode());
}
@Override
public String toString() {
String newLine = System.lineSeparator();
return new StringBuilder()
.append("class CallsStaticSipTrunkRequest {")
.append(newLine)
.append(" ")
.append(toIndentedString(super.toString()))
.append(newLine)
.append(" sourceHosts: ")
.append(toIndentedString(sourceHosts))
.append(newLine)
.append(" destinationHosts: ")
.append(toIndentedString(destinationHosts))
.append(newLine)
.append(" strategy: ")
.append(toIndentedString(strategy))
.append(newLine)
.append(" sipOptions: ")
.append(toIndentedString(sipOptions))
.append(newLine)
.append("}")
.toString();
}
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
String lineSeparator = System.lineSeparator();
String lineSeparatorFollowedByIndentation = lineSeparator + " ";
return o.toString().replace(lineSeparator, lineSeparatorFollowedByIndentation);
}
}