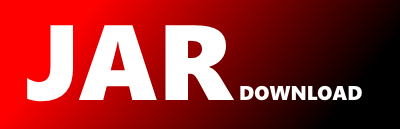
com.infobip.model.EmailReport Maven / Gradle / Ivy
/*
* This class is auto generated from the Infobip OpenAPI specification
* through the OpenAPI Specification Client API libraries (Re)Generator (OSCAR),
* powered by the OpenAPI Generator (https://openapi-generator.tech).
*
* Do not edit manually. To learn how to raise an issue, see the CONTRIBUTING guide
* or contact us @ [email protected].
*/
package com.infobip.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
import java.util.Objects;
/**
* Represents EmailReport model.
*/
public class EmailReport {
private String applicationId;
private String entityId;
private String bulkId;
private String messageId;
private String to;
private OffsetDateTime sentAt;
private OffsetDateTime doneAt;
private Integer messageCount;
private MessagePrice price;
private MessageStatus status;
private EmailError error;
/**
* Sets applicationId.
*
* Field description:
* The Application ID sent in the email request.
*
* @param applicationId
* @return This {@link EmailReport instance}.
*/
public EmailReport applicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
/**
* Returns applicationId.
*
* Field description:
* The Application ID sent in the email request.
*
* @return applicationId
*/
@JsonProperty("applicationId")
public String getApplicationId() {
return applicationId;
}
/**
* Sets applicationId.
*
* Field description:
* The Application ID sent in the email request.
*
* @param applicationId
*/
@JsonProperty("applicationId")
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
/**
* Sets entityId.
*
* Field description:
* The Entity ID sent in the email request.
*
* @param entityId
* @return This {@link EmailReport instance}.
*/
public EmailReport entityId(String entityId) {
this.entityId = entityId;
return this;
}
/**
* Returns entityId.
*
* Field description:
* The Entity ID sent in the email request.
*
* @return entityId
*/
@JsonProperty("entityId")
public String getEntityId() {
return entityId;
}
/**
* Sets entityId.
*
* Field description:
* The Entity ID sent in the email request.
*
* @param entityId
*/
@JsonProperty("entityId")
public void setEntityId(String entityId) {
this.entityId = entityId;
}
/**
* Sets bulkId.
*
* Field description:
* The ID that uniquely identifies bulks of request.
*
* @param bulkId
* @return This {@link EmailReport instance}.
*/
public EmailReport bulkId(String bulkId) {
this.bulkId = bulkId;
return this;
}
/**
* Returns bulkId.
*
* Field description:
* The ID that uniquely identifies bulks of request.
*
* @return bulkId
*/
@JsonProperty("bulkId")
public String getBulkId() {
return bulkId;
}
/**
* Sets bulkId.
*
* Field description:
* The ID that uniquely identifies bulks of request.
*
* @param bulkId
*/
@JsonProperty("bulkId")
public void setBulkId(String bulkId) {
this.bulkId = bulkId;
}
/**
* Sets messageId.
*
* Field description:
* The ID that uniquely identifies the sent email request.
*
* @param messageId
* @return This {@link EmailReport instance}.
*/
public EmailReport messageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Returns messageId.
*
* Field description:
* The ID that uniquely identifies the sent email request.
*
* @return messageId
*/
@JsonProperty("messageId")
public String getMessageId() {
return messageId;
}
/**
* Sets messageId.
*
* Field description:
* The ID that uniquely identifies the sent email request.
*
* @param messageId
*/
@JsonProperty("messageId")
public void setMessageId(String messageId) {
this.messageId = messageId;
}
/**
* Sets to.
*
* Field description:
* The recipient email address.
*
* @param to
* @return This {@link EmailReport instance}.
*/
public EmailReport to(String to) {
this.to = to;
return this;
}
/**
* Returns to.
*
* Field description:
* The recipient email address.
*
* @return to
*/
@JsonProperty("to")
public String getTo() {
return to;
}
/**
* Sets to.
*
* Field description:
* The recipient email address.
*
* @param to
*/
@JsonProperty("to")
public void setTo(String to) {
this.to = to;
}
/**
* Sets sentAt.
*
* Field description:
* Tells when the email was initiated. Has the following format: `yyyy-MM-dd'T'HH:mm:ss.SSSZ`.
*
* @param sentAt
* @return This {@link EmailReport instance}.
*/
public EmailReport sentAt(OffsetDateTime sentAt) {
this.sentAt = sentAt;
return this;
}
/**
* Returns sentAt.
*
* Field description:
* Tells when the email was initiated. Has the following format: `yyyy-MM-dd'T'HH:mm:ss.SSSZ`.
*
* @return sentAt
*/
@JsonProperty("sentAt")
public OffsetDateTime getSentAt() {
return sentAt;
}
/**
* Sets sentAt.
*
* Field description:
* Tells when the email was initiated. Has the following format: `yyyy-MM-dd'T'HH:mm:ss.SSSZ`.
*
* @param sentAt
*/
@JsonProperty("sentAt")
public void setSentAt(OffsetDateTime sentAt) {
this.sentAt = sentAt;
}
/**
* Sets doneAt.
*
* Field description:
* Tells when the email request was processed by Infobip.
*
* @param doneAt
* @return This {@link EmailReport instance}.
*/
public EmailReport doneAt(OffsetDateTime doneAt) {
this.doneAt = doneAt;
return this;
}
/**
* Returns doneAt.
*
* Field description:
* Tells when the email request was processed by Infobip.
*
* @return doneAt
*/
@JsonProperty("doneAt")
public OffsetDateTime getDoneAt() {
return doneAt;
}
/**
* Sets doneAt.
*
* Field description:
* Tells when the email request was processed by Infobip.
*
* @param doneAt
*/
@JsonProperty("doneAt")
public void setDoneAt(OffsetDateTime doneAt) {
this.doneAt = doneAt;
}
/**
* Sets messageCount.
*
* Field description:
* Email request count.
*
* @param messageCount
* @return This {@link EmailReport instance}.
*/
public EmailReport messageCount(Integer messageCount) {
this.messageCount = messageCount;
return this;
}
/**
* Returns messageCount.
*
* Field description:
* Email request count.
*
* @return messageCount
*/
@JsonProperty("messageCount")
public Integer getMessageCount() {
return messageCount;
}
/**
* Sets messageCount.
*
* Field description:
* Email request count.
*
* @param messageCount
*/
@JsonProperty("messageCount")
public void setMessageCount(Integer messageCount) {
this.messageCount = messageCount;
}
/**
* Sets price.
*
* @param price
* @return This {@link EmailReport instance}.
*/
public EmailReport price(MessagePrice price) {
this.price = price;
return this;
}
/**
* Returns price.
*
* @return price
*/
@JsonProperty("price")
public MessagePrice getPrice() {
return price;
}
/**
* Sets price.
*
* @param price
*/
@JsonProperty("price")
public void setPrice(MessagePrice price) {
this.price = price;
}
/**
* Sets status.
*
* @param status
* @return This {@link EmailReport instance}.
*/
public EmailReport status(MessageStatus status) {
this.status = status;
return this;
}
/**
* Returns status.
*
* @return status
*/
@JsonProperty("status")
public MessageStatus getStatus() {
return status;
}
/**
* Sets status.
*
* @param status
*/
@JsonProperty("status")
public void setStatus(MessageStatus status) {
this.status = status;
}
/**
* Sets error.
*
* @param error
* @return This {@link EmailReport instance}.
*/
public EmailReport error(EmailError error) {
this.error = error;
return this;
}
/**
* Returns error.
*
* @return error
*/
@JsonProperty("error")
public EmailError getError() {
return error;
}
/**
* Sets error.
*
* @param error
*/
@JsonProperty("error")
public void setError(EmailError error) {
this.error = error;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EmailReport emailReport = (EmailReport) o;
return Objects.equals(this.applicationId, emailReport.applicationId)
&& Objects.equals(this.entityId, emailReport.entityId)
&& Objects.equals(this.bulkId, emailReport.bulkId)
&& Objects.equals(this.messageId, emailReport.messageId)
&& Objects.equals(this.to, emailReport.to)
&& Objects.equals(this.sentAt, emailReport.sentAt)
&& Objects.equals(this.doneAt, emailReport.doneAt)
&& Objects.equals(this.messageCount, emailReport.messageCount)
&& Objects.equals(this.price, emailReport.price)
&& Objects.equals(this.status, emailReport.status)
&& Objects.equals(this.error, emailReport.error);
}
@Override
public int hashCode() {
return Objects.hash(
applicationId, entityId, bulkId, messageId, to, sentAt, doneAt, messageCount, price, status, error);
}
@Override
public String toString() {
String newLine = System.lineSeparator();
return new StringBuilder()
.append("class EmailReport {")
.append(newLine)
.append(" applicationId: ")
.append(toIndentedString(applicationId))
.append(newLine)
.append(" entityId: ")
.append(toIndentedString(entityId))
.append(newLine)
.append(" bulkId: ")
.append(toIndentedString(bulkId))
.append(newLine)
.append(" messageId: ")
.append(toIndentedString(messageId))
.append(newLine)
.append(" to: ")
.append(toIndentedString(to))
.append(newLine)
.append(" sentAt: ")
.append(toIndentedString(sentAt))
.append(newLine)
.append(" doneAt: ")
.append(toIndentedString(doneAt))
.append(newLine)
.append(" messageCount: ")
.append(toIndentedString(messageCount))
.append(newLine)
.append(" price: ")
.append(toIndentedString(price))
.append(newLine)
.append(" status: ")
.append(toIndentedString(status))
.append(newLine)
.append(" error: ")
.append(toIndentedString(error))
.append(newLine)
.append("}")
.toString();
}
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
String lineSeparator = System.lineSeparator();
String lineSeparatorFollowedByIndentation = lineSeparator + " ";
return o.toString().replace(lineSeparator, lineSeparatorFollowedByIndentation);
}
}