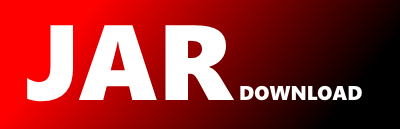
com.infobip.model.MmsResult Maven / Gradle / Ivy
/*
* This class is auto generated from the Infobip OpenAPI specification
* through the OpenAPI Specification Client API libraries (Re)Generator (OSCAR),
* powered by the OpenAPI Generator (https://openapi-generator.tech).
*
* Do not edit manually. To learn how to raise an issue, see the CONTRIBUTING guide
* or contact us @ [email protected].
*/
package com.infobip.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/**
* Collection of reports, one per every received message.
*/
public class MmsResult {
private String entityId;
private String applicationId;
private String from;
private String to;
private OffsetDateTime receivedAt;
private String messageId;
private String pairedMessageId;
private String callbackData;
private String userAgent;
private MmsInboundContent message;
private List group = null;
private MmsMessagePrice price;
/**
* Sets entityId.
*
* Field description:
* The ID of the entity, if application and entity management is used.
*
* @param entityId
* @return This {@link MmsResult instance}.
*/
public MmsResult entityId(String entityId) {
this.entityId = entityId;
return this;
}
/**
* Returns entityId.
*
* Field description:
* The ID of the entity, if application and entity management is used.
*
* @return entityId
*/
@JsonProperty("entityId")
public String getEntityId() {
return entityId;
}
/**
* Sets entityId.
*
* Field description:
* The ID of the entity, if application and entity management is used.
*
* @param entityId
*/
@JsonProperty("entityId")
public void setEntityId(String entityId) {
this.entityId = entityId;
}
/**
* Sets applicationId.
*
* Field description:
* The ID of the application, if application and entity management is used.
*
* @param applicationId
* @return This {@link MmsResult instance}.
*/
public MmsResult applicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
/**
* Returns applicationId.
*
* Field description:
* The ID of the application, if application and entity management is used.
*
* @return applicationId
*/
@JsonProperty("applicationId")
public String getApplicationId() {
return applicationId;
}
/**
* Sets applicationId.
*
* Field description:
* The ID of the application, if application and entity management is used.
*
* @param applicationId
*/
@JsonProperty("applicationId")
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
/**
* Sets from.
*
* Field description:
* Number which sent the message.
*
* @param from
* @return This {@link MmsResult instance}.
*/
public MmsResult from(String from) {
this.from = from;
return this;
}
/**
* Returns from.
*
* Field description:
* Number which sent the message.
*
* @return from
*/
@JsonProperty("from")
public String getFrom() {
return from;
}
/**
* Sets from.
*
* Field description:
* Number which sent the message.
*
* @param from
*/
@JsonProperty("from")
public void setFrom(String from) {
this.from = from;
}
/**
* Sets to.
*
* Field description:
* Sender provided during the activation process.
*
* @param to
* @return This {@link MmsResult instance}.
*/
public MmsResult to(String to) {
this.to = to;
return this;
}
/**
* Returns to.
*
* Field description:
* Sender provided during the activation process.
*
* @return to
*/
@JsonProperty("to")
public String getTo() {
return to;
}
/**
* Sets to.
*
* Field description:
* Sender provided during the activation process.
*
* @param to
*/
@JsonProperty("to")
public void setTo(String to) {
this.to = to;
}
/**
* Sets receivedAt.
*
* Field description:
* Date and time when Infobip received the message.
*
* @param receivedAt
* @return This {@link MmsResult instance}.
*/
public MmsResult receivedAt(OffsetDateTime receivedAt) {
this.receivedAt = receivedAt;
return this;
}
/**
* Returns receivedAt.
*
* Field description:
* Date and time when Infobip received the message.
*
* @return receivedAt
*/
@JsonProperty("receivedAt")
public OffsetDateTime getReceivedAt() {
return receivedAt;
}
/**
* Sets receivedAt.
*
* Field description:
* Date and time when Infobip received the message.
*
* @param receivedAt
*/
@JsonProperty("receivedAt")
public void setReceivedAt(OffsetDateTime receivedAt) {
this.receivedAt = receivedAt;
}
/**
* Sets messageId.
*
* Field description:
* The ID that uniquely identifies the received message.
*
* @param messageId
* @return This {@link MmsResult instance}.
*/
public MmsResult messageId(String messageId) {
this.messageId = messageId;
return this;
}
/**
* Returns messageId.
*
* Field description:
* The ID that uniquely identifies the received message.
*
* @return messageId
*/
@JsonProperty("messageId")
public String getMessageId() {
return messageId;
}
/**
* Sets messageId.
*
* Field description:
* The ID that uniquely identifies the received message.
*
* @param messageId
*/
@JsonProperty("messageId")
public void setMessageId(String messageId) {
this.messageId = messageId;
}
/**
* Sets pairedMessageId.
*
* Field description:
* Message ID of paired outgoing message if matched by Infobip platform.
*
* @param pairedMessageId
* @return This {@link MmsResult instance}.
*/
public MmsResult pairedMessageId(String pairedMessageId) {
this.pairedMessageId = pairedMessageId;
return this;
}
/**
* Returns pairedMessageId.
*
* Field description:
* Message ID of paired outgoing message if matched by Infobip platform.
*
* @return pairedMessageId
*/
@JsonProperty("pairedMessageId")
public String getPairedMessageId() {
return pairedMessageId;
}
/**
* Sets pairedMessageId.
*
* Field description:
* Message ID of paired outgoing message if matched by Infobip platform.
*
* @param pairedMessageId
*/
@JsonProperty("pairedMessageId")
public void setPairedMessageId(String pairedMessageId) {
this.pairedMessageId = pairedMessageId;
}
/**
* Sets callbackData.
*
* Field description:
* Callback data sent through ‛callbackData‛ field when sending message.
*
* @param callbackData
* @return This {@link MmsResult instance}.
*/
public MmsResult callbackData(String callbackData) {
this.callbackData = callbackData;
return this;
}
/**
* Returns callbackData.
*
* Field description:
* Callback data sent through ‛callbackData‛ field when sending message.
*
* @return callbackData
*/
@JsonProperty("callbackData")
public String getCallbackData() {
return callbackData;
}
/**
* Sets callbackData.
*
* Field description:
* Callback data sent through ‛callbackData‛ field when sending message.
*
* @param callbackData
*/
@JsonProperty("callbackData")
public void setCallbackData(String callbackData) {
this.callbackData = callbackData;
}
/**
* Sets userAgent.
*
* Field description:
* Identifier of the device from which the message was sent.
*
* @param userAgent
* @return This {@link MmsResult instance}.
*/
public MmsResult userAgent(String userAgent) {
this.userAgent = userAgent;
return this;
}
/**
* Returns userAgent.
*
* Field description:
* Identifier of the device from which the message was sent.
*
* @return userAgent
*/
@JsonProperty("userAgent")
public String getUserAgent() {
return userAgent;
}
/**
* Sets userAgent.
*
* Field description:
* Identifier of the device from which the message was sent.
*
* @param userAgent
*/
@JsonProperty("userAgent")
public void setUserAgent(String userAgent) {
this.userAgent = userAgent;
}
/**
* Sets message.
*
* @param message
* @return This {@link MmsResult instance}.
*/
public MmsResult message(MmsInboundContent message) {
this.message = message;
return this;
}
/**
* Returns message.
*
* @return message
*/
@JsonProperty("message")
public MmsInboundContent getMessage() {
return message;
}
/**
* Sets message.
*
* @param message
*/
@JsonProperty("message")
public void setMessage(MmsInboundContent message) {
this.message = message;
}
/**
* Sets group.
*
* Field description:
* Recipients of group MMS.
*
* @param group
* @return This {@link MmsResult instance}.
*/
public MmsResult group(List group) {
this.group = group;
return this;
}
/**
* Adds and item into group.
*
* Field description:
* Recipients of group MMS.
*
* @param groupItem The item to be added to the list.
* @return This {@link MmsResult instance}.
*/
public MmsResult addGroupItem(MmsInboundDestination groupItem) {
if (this.group == null) {
this.group = new ArrayList<>();
}
this.group.add(groupItem);
return this;
}
/**
* Returns group.
*
* Field description:
* Recipients of group MMS.
*
* @return group
*/
@JsonProperty("group")
public List getGroup() {
return group;
}
/**
* Sets group.
*
* Field description:
* Recipients of group MMS.
*
* @param group
*/
@JsonProperty("group")
public void setGroup(List group) {
this.group = group;
}
/**
* Sets price.
*
* @param price
* @return This {@link MmsResult instance}.
*/
public MmsResult price(MmsMessagePrice price) {
this.price = price;
return this;
}
/**
* Returns price.
*
* @return price
*/
@JsonProperty("price")
public MmsMessagePrice getPrice() {
return price;
}
/**
* Sets price.
*
* @param price
*/
@JsonProperty("price")
public void setPrice(MmsMessagePrice price) {
this.price = price;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MmsResult mmsResult = (MmsResult) o;
return Objects.equals(this.entityId, mmsResult.entityId)
&& Objects.equals(this.applicationId, mmsResult.applicationId)
&& Objects.equals(this.from, mmsResult.from)
&& Objects.equals(this.to, mmsResult.to)
&& Objects.equals(this.receivedAt, mmsResult.receivedAt)
&& Objects.equals(this.messageId, mmsResult.messageId)
&& Objects.equals(this.pairedMessageId, mmsResult.pairedMessageId)
&& Objects.equals(this.callbackData, mmsResult.callbackData)
&& Objects.equals(this.userAgent, mmsResult.userAgent)
&& Objects.equals(this.message, mmsResult.message)
&& Objects.equals(this.group, mmsResult.group)
&& Objects.equals(this.price, mmsResult.price);
}
@Override
public int hashCode() {
return Objects.hash(
entityId,
applicationId,
from,
to,
receivedAt,
messageId,
pairedMessageId,
callbackData,
userAgent,
message,
group,
price);
}
@Override
public String toString() {
String newLine = System.lineSeparator();
return new StringBuilder()
.append("class MmsResult {")
.append(newLine)
.append(" entityId: ")
.append(toIndentedString(entityId))
.append(newLine)
.append(" applicationId: ")
.append(toIndentedString(applicationId))
.append(newLine)
.append(" from: ")
.append(toIndentedString(from))
.append(newLine)
.append(" to: ")
.append(toIndentedString(to))
.append(newLine)
.append(" receivedAt: ")
.append(toIndentedString(receivedAt))
.append(newLine)
.append(" messageId: ")
.append(toIndentedString(messageId))
.append(newLine)
.append(" pairedMessageId: ")
.append(toIndentedString(pairedMessageId))
.append(newLine)
.append(" callbackData: ")
.append(toIndentedString(callbackData))
.append(newLine)
.append(" userAgent: ")
.append(toIndentedString(userAgent))
.append(newLine)
.append(" message: ")
.append(toIndentedString(message))
.append(newLine)
.append(" group: ")
.append(toIndentedString(group))
.append(newLine)
.append(" price: ")
.append(toIndentedString(price))
.append(newLine)
.append("}")
.toString();
}
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
String lineSeparator = System.lineSeparator();
String lineSeparatorFollowedByIndentation = lineSeparator + " ";
return o.toString().replace(lineSeparator, lineSeparatorFollowedByIndentation);
}
}