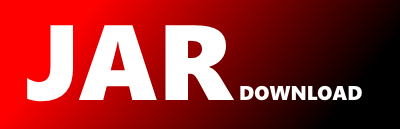
com.infobip.model.ViberOutboundVideoContent Maven / Gradle / Ivy
/*
* This class is auto generated from the Infobip OpenAPI specification
* through the OpenAPI Specification Client API libraries (Re)Generator (OSCAR),
* powered by the OpenAPI Generator (https://openapi-generator.tech).
*
* Do not edit manually. To learn how to raise an issue, see the CONTRIBUTING guide
* or contact us @ [email protected].
*/
package com.infobip.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Objects;
/**
* Represents ViberOutboundVideoContent model.
*/
public class ViberOutboundVideoContent extends ViberOutboundContent {
private String text;
private String mediaUrl;
private String mediaDuration;
private String thumbnailUrl;
private String buttonTitle;
/**
* Constructs a new {@link ViberOutboundVideoContent} instance.
*/
public ViberOutboundVideoContent() {
super("VIDEO");
}
/**
* Sets text.
*
* Field description:
* Text to be displayed alongside the video.
*
* @param text
* @return This {@link ViberOutboundVideoContent instance}.
*/
public ViberOutboundVideoContent text(String text) {
this.text = text;
return this;
}
/**
* Returns text.
*
* Field description:
* Text to be displayed alongside the video.
*
* @return text
*/
@JsonProperty("text")
public String getText() {
return text;
}
/**
* Sets text.
*
* Field description:
* Text to be displayed alongside the video.
*
* @param text
*/
@JsonProperty("text")
public void setText(String text) {
this.text = text;
}
/**
* Sets mediaUrl.
*
* Field description:
* URL of the video being sent. Must be a valid URL starting with https:// or http://. The resource must allow HEAD request with 'Content-Length' header. Supported video types are .3gp, .m4v, .mov, .mp4. Maximum video size is 200 MB.
*
* The field is required.
*
* @param mediaUrl
* @return This {@link ViberOutboundVideoContent instance}.
*/
public ViberOutboundVideoContent mediaUrl(String mediaUrl) {
this.mediaUrl = mediaUrl;
return this;
}
/**
* Returns mediaUrl.
*
* Field description:
* URL of the video being sent. Must be a valid URL starting with https:// or http://. The resource must allow HEAD request with 'Content-Length' header. Supported video types are .3gp, .m4v, .mov, .mp4. Maximum video size is 200 MB.
*
* The field is required.
*
* @return mediaUrl
*/
@JsonProperty("mediaUrl")
public String getMediaUrl() {
return mediaUrl;
}
/**
* Sets mediaUrl.
*
* Field description:
* URL of the video being sent. Must be a valid URL starting with https:// or http://. The resource must allow HEAD request with 'Content-Length' header. Supported video types are .3gp, .m4v, .mov, .mp4. Maximum video size is 200 MB.
*
* The field is required.
*
* @param mediaUrl
*/
@JsonProperty("mediaUrl")
public void setMediaUrl(String mediaUrl) {
this.mediaUrl = mediaUrl;
}
/**
* Sets mediaDuration.
*
* Field description:
* How long is the video.
*
* The field is required.
*
* @param mediaDuration
* @return This {@link ViberOutboundVideoContent instance}.
*/
public ViberOutboundVideoContent mediaDuration(String mediaDuration) {
this.mediaDuration = mediaDuration;
return this;
}
/**
* Returns mediaDuration.
*
* Field description:
* How long is the video.
*
* The field is required.
*
* @return mediaDuration
*/
@JsonProperty("mediaDuration")
public String getMediaDuration() {
return mediaDuration;
}
/**
* Sets mediaDuration.
*
* Field description:
* How long is the video.
*
* The field is required.
*
* @param mediaDuration
*/
@JsonProperty("mediaDuration")
public void setMediaDuration(String mediaDuration) {
this.mediaDuration = mediaDuration;
}
/**
* Sets thumbnailUrl.
*
* Field description:
* URL of the thumbnail being sent. Must be a valid URL starting with https:// or http://. Supported thumbnail types are .jpg, .jpeg, .png. Recommended resolution: 800 px x 800 px.
*
* The field is required.
*
* @param thumbnailUrl
* @return This {@link ViberOutboundVideoContent instance}.
*/
public ViberOutboundVideoContent thumbnailUrl(String thumbnailUrl) {
this.thumbnailUrl = thumbnailUrl;
return this;
}
/**
* Returns thumbnailUrl.
*
* Field description:
* URL of the thumbnail being sent. Must be a valid URL starting with https:// or http://. Supported thumbnail types are .jpg, .jpeg, .png. Recommended resolution: 800 px x 800 px.
*
* The field is required.
*
* @return thumbnailUrl
*/
@JsonProperty("thumbnailUrl")
public String getThumbnailUrl() {
return thumbnailUrl;
}
/**
* Sets thumbnailUrl.
*
* Field description:
* URL of the thumbnail being sent. Must be a valid URL starting with https:// or http://. Supported thumbnail types are .jpg, .jpeg, .png. Recommended resolution: 800 px x 800 px.
*
* The field is required.
*
* @param thumbnailUrl
*/
@JsonProperty("thumbnailUrl")
public void setThumbnailUrl(String thumbnailUrl) {
this.thumbnailUrl = thumbnailUrl;
}
/**
* Sets buttonTitle.
*
* Field description:
* The text that will appear on the button.
*
* @param buttonTitle
* @return This {@link ViberOutboundVideoContent instance}.
*/
public ViberOutboundVideoContent buttonTitle(String buttonTitle) {
this.buttonTitle = buttonTitle;
return this;
}
/**
* Returns buttonTitle.
*
* Field description:
* The text that will appear on the button.
*
* @return buttonTitle
*/
@JsonProperty("buttonTitle")
public String getButtonTitle() {
return buttonTitle;
}
/**
* Sets buttonTitle.
*
* Field description:
* The text that will appear on the button.
*
* @param buttonTitle
*/
@JsonProperty("buttonTitle")
public void setButtonTitle(String buttonTitle) {
this.buttonTitle = buttonTitle;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ViberOutboundVideoContent viberOutboundVideoContent = (ViberOutboundVideoContent) o;
return Objects.equals(this.text, viberOutboundVideoContent.text)
&& Objects.equals(this.mediaUrl, viberOutboundVideoContent.mediaUrl)
&& Objects.equals(this.mediaDuration, viberOutboundVideoContent.mediaDuration)
&& Objects.equals(this.thumbnailUrl, viberOutboundVideoContent.thumbnailUrl)
&& Objects.equals(this.buttonTitle, viberOutboundVideoContent.buttonTitle)
&& super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(text, mediaUrl, mediaDuration, thumbnailUrl, buttonTitle, super.hashCode());
}
@Override
public String toString() {
String newLine = System.lineSeparator();
return new StringBuilder()
.append("class ViberOutboundVideoContent {")
.append(newLine)
.append(" ")
.append(toIndentedString(super.toString()))
.append(newLine)
.append(" text: ")
.append(toIndentedString(text))
.append(newLine)
.append(" mediaUrl: ")
.append(toIndentedString(mediaUrl))
.append(newLine)
.append(" mediaDuration: ")
.append(toIndentedString(mediaDuration))
.append(newLine)
.append(" thumbnailUrl: ")
.append(toIndentedString(thumbnailUrl))
.append(newLine)
.append(" buttonTitle: ")
.append(toIndentedString(buttonTitle))
.append(newLine)
.append("}")
.toString();
}
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
String lineSeparator = System.lineSeparator();
String lineSeparatorFollowedByIndentation = lineSeparator + " ";
return o.toString().replace(lineSeparator, lineSeparatorFollowedByIndentation);
}
}